Table of Contents
Toggle
What is Object-Oriented Programming?
Object-Oriented Programming (OOP) is one of the programming pattern in computer science engineering. It focuses on the concepts of class and object. Programming languages like Java, C++, Python, C#, Ruby, and JavaScript run under the principle of OOP features. This programming paradigms has many benefits to offer in software engineering. Today, in this article, we will understand the concept of OOP in detail with the help of C++. So, let’s delve into it!

What is C++?
C++ is an extension of C. It is a multi-purpose, high-level, compiler-based, and object-oriented programming language. Using C++, you can create operating systems, editors, commercial apps, databases, translators, device drivers, games, and design protocols. So this is the simple definition of C++. Now let’s move on to the main concept of OOP.

How to Structure Object-oriented Programs?
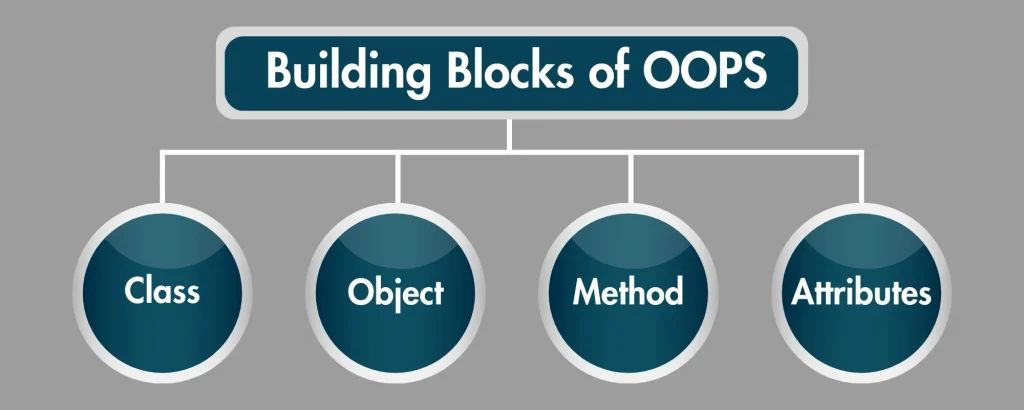
In OOP, class, object, attributes, and methods are the building blocks.
- Class: Class is a blueprint to construct the instances (objects). It is private by default and contains both data members (variables) and member functions (functions). Programmers can easily declare another class, and we can derive another class from one class. Whenever the class is created, it doesn’t create any memory. But when the objects are created, memory is allocated.
- Objects: It is an instance of a class or a physical representation of a class. In simple words, an object is a tangible entity that has some behavior or characteristics.
- Attributes: It is nothing but variables that define the state of the class objects. In class, whatever we define inside the member functions, we consider it an attribute.
- Methods: It is simply a function that is declared inside the class, which is also known as a data member or member function. Its main role is to define the behavior of the class objects.
Let’s understand these concepts with the help of a cat:
Think of cats as a class. The cat will have a name, a color of fur, an age, etc. These become the attributes of a cat, except the name. Since the objects are the physical representation of a class, the name of the cat is specific. Thus, a cat named, let’s say, Jerry, will be the instance (object) of a class cat. And the method of a cat can be something the cat can do, for example, “a cat can wag the tail” or “make a purr.” This same concept is applied in the structure of class syntax down below:
Now let’s move towards its features, which are none other than the four pillars of OOP. To write better code, you must know these features in detail.

What Are the Four Pillars of OOP?

There are four features in OOP, which are often referred to as the four pillars. Let’s have a look at them:
- Encapsulation
- Inheritance
- Polymorphism
- Abstraction
So, let’s learn about each one by one:
Encapsulation: It is a concept of wrapping or binding the methods and variables into one unit is called encapsulation. It is a way to restrict direct access to some of an object’s components inside the class or prevent any kind of violation. It keeps the data safe and secure. Think of it like a secret place where you can hide important and valuable data. And no one will have access; only the owner will have control over it. Some of the benefits of encapsulation are that the program is highly secure and has a simple debugging process.
#include<iostream>
using namespace std;
class Encap
{
private:
int age;
public:
string name;
void setValue(int a)
(
age=a;
cout<<age<<endl;
}
};
main()
{
Encap E;
E.name=” Joe”;
E.setValue(44);
cout<<E.name<<endl;
}
Inheritance: It allows to inherit properties and features from the main (parent) class to another (child) class. In simple words, it’s like a child inheriting traits from their parents. By using the concept of inheritance in the program, working with complex programs and large software becomes easy. Since the changes made in the parent class will automatically be inherited into the child class, this promotes reusability, reduces development and maintenance costs, reduces code redundancy, and supports code extensibility.
#include<iostream>
using namespace std;
class Dad
{
protected: string surname= “Smith”;
};
class Son:Dad
{
string name= ”Will”;
public:
void show()
{
cout<<name<<” ”<<surmane;
}
};
class daughter:Dad
{
string name= ”Anna”;
public:
void disp()
{
cout<<name<<” ”<<surmane;
}
};
main()
{
Son smyObj;
smyObj.show();
Daughter dmyObj;
dmyObj.disp();
}
The output of this program will be Will Smith and Anna Smith.
This is one type of inheritance, which is called a single inheritance. In this program, we saw how we can inherit the member function of the parent class of dad.
Abstraction: It refers to the concept of hiding the underlying details and only showing the necessary information to the outside world. The abstraction concept is similar to encapsulation. To understand this, let’s take a simple real-life example of a washing machine. When we use a washing machine to wash our clothes, we simply press the button, and it automatically does it. So, we don’t need to know the internal complexity. Some of the benefits of abstraction are improve security, avoid code duplication, and increase reusability.
#include<iostream>
using namespace std;
class WashingMachine
{
bool PowerOn;
public:
void start()
{
PowerOn=true;
cout<<”Washing Started :)”<<endl;
}
void dry()
{
if (PowerOn)
{
cout<<”Wash the clothes : )”;
}
else
{
cout<<”Dry the clothes ”;
}
};
main()
WashingMachine wmObj;
wmObj.start();
wmObj.dry();
Polymorphism: In programming languages, polymorphism refers to a term that has more than one form. Polymorphism is one of the important concepts in OOP. It allows the same function to execute different behaviors. Through this feature, objects can share behaviors, or two different objects can reply to one form. Polymorphism is of two types:
- Method Overloading
- Method Overriding.
To understand the concept in a better way, let’s take the example of a child. When the child is inside the school campus, he is a student; when he goes to the mall, he is a customer; and at home, he is a son. Some of the benefits of abstractions are code and class reusability, and with one variable, you can store multiple data types.
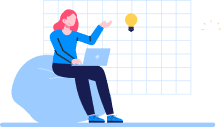
Don't miss out on your chance to work with the best
apply for top global job opportunities today!
Also Read: Top OOPs Interview Questions and Answers

In Conclusion
OOP programs are private by default. Due to this, outside functions cannot access the member functions of that class. Only member functions of the same class can access the function. To access the member function of another class, we can use the access specifiers, i.e., public, private, and protected, in the program. Objects and classes help to bind data and functions together. Leveraging the concepts of inheritance and polymorphism into the program contributes to the code’s reusability and simplifies maintenance. Therefore, OOPs robust design patterns and procedures make programs secure from outside functions. All in all, OOP is a strong foundation for modern software development. It allows programmers to create better data structures with features that promote integrity, security, and maintenance.
Take control of your career and land your dream job
sign up with us now and start applying for the best opportunities!
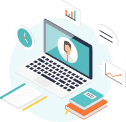

Frequently Asked Questions
Polymorphism and inheritance are different concepts in OOPS. Inheritance allows the creation of a hierarchy of classes where the child class inherits the properties and behaviors of the parent class. Polymorphism treats objects of different classes as objects of a common superclass so that it can perform multiple forms and behaviors.
Abstraction is of two kinds:
Method abstraction: This type of method does not disclose the inner workings of the application and hides them from the outside world.
Data abstraction: It hides the real complexities of applications but shows their fundamental traits.
You can use polymorphism in method overriding, interface and abstract classes, dependency injection, frameworks, and inheritance.
It makes your code flexible and adaptable. It also allows modification of the internal details without ruining the external code by separating the abstraction from its implementation.
There are two types: compile-time and runtime polymorphism. Compile-time is done in method overloading, and runtime polymorphism is done in method overriding.