Table of Contents
Toggle
Introduction
Object-oriented programming (OOP) is a paradigm that helps build reliable designs that can guarantee scalability without blocking the main thread in a program. As we all know, OOP follows the best practices to build systems and is a primary component of programming languages. This makes it essential for every interview candidate to have a good knowledge of OOPs. In this article, we have compiled the top OOP questions and answers to help you ace your interview. Read on to know more!
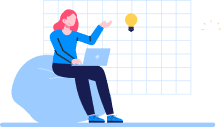
Don't miss out on your chance to work with the best
apply for top global job opportunities today!

Top OOPs Interview Questions and Answers
1. What is OOP?
OOP or object-oriented programming is a programming model in which software is organized around data and objects. An object is a collection of data and the methods operating on that data.
2. Why do we need OOP?
OOP manages code better as it reduces the gap between the language spoken by the developers and understood by users. Because of encapsulation, the methods remain the same, and it is easy to change the underlying representation. This facilitates easy maintenance and makes OOP a great choice for managing big software.
3. What are some OOP languages?
- Python
- JavaScript
- Java
- C++
- C#
- Ruby
- TypeScript
- Kotlin
- R
- PHP
4. What are the other programming paradigms apart from OOP?
A programming paradigm defines the style in which one can organize a given program or programming language. Programming paradigms can be divided into:
Imperative programming
It allows programmers to dictate the step-by-step logic of the program. It is further divided into:
- Procedural programming
- Object-Oriented Programming or OOP
- Parallel Programming
Declarative programming
It pays attention to the execution of a program rather than how it should be executed. The logic of a computation is decided without considering its control flow. It can be further divided into:
- Logical programming
- Functional programming
- Database programming
5. What is Structured Programming?
Programs that have a completely controlled flow come under Structured Programming. Structured programming has a block with a set of rules with a definitive control flow. Most programming paradigms, including OOP, Structured Programming.
6. What are the features of OOP?
Polymorphism: This feature allows the addition of different meanings to a single component.
Encapsulation: It refers to enclosing the data/variables and the methods into a class for data manipulation.
Inheritance: It allows a class to inherit the properties and methods of the parent class, the base class, or the super class.
Data Abstraction: This feature lets you remove unnecessary elements to make the program simpler.
7. What are the advantages of OOP?
- Offers enhanced code reusability
- Code easier to update and maintain
- Restricts data access and offers better security
- Can be implemented quickly and easily redesigned
8. What are the disadvantages of OOPs?
- OOPs need extensive planning.
- It takes time to solve a problem with OOP.
- OOP is not a great choice for small problems.
- It requires intensive testing.
9. What makes OOP so popular?
OOP is popular because it is easier to write complex code with OOP. Moreover, OOP makes it simpler to maintain code and solve complex scenarios.
10. What is a class?
It is a prototype that has objects in different states with different behaviors. It is like a blueprint with values (data or members) and a set of rules (behaviors or functions).
11. What is an object?
An object is a basic unit of OOP with its own state, behavior and attribute. It is the instance of the class that includes the instance of the members and behaviors defined in the class template.
12. What is Compile Time Polymorphism? How is it different from Runtime Polymorphism?
It is a type of polymorphism that occurs at compile time when the compiler decides the shape or value to be taken by the entity in the picture.
Example:
// In this program, we will see how multiple functions are created with the same name,
// but the compiler decides which function to call easily at the compile time itself.
class CompileTimePolymorphism{
// 1st method with name add
public int add(int x, int y){
return x+y;
}
// 2nd method with name add
public int add(int x, int y, int z){
return x+y+z;
}
// 3rd method with name add
public int add(double x, int y){
return (int)x+y;
}
// 4th method with name add
public int add(int x, double y){
return x+(int)y;
}
}
class Test{
public static void main(String[] args){
CompileTimePolymorphism demo=new CompileTimePolymorphism();
// In the below statement, the Compiler looks at the argument types and decides to call method 1
System.out.println(demo.add(2,3));
// Similarly, in the below statement, the compiler calls method 2
System.out.println(demo.add(2,3,4));
// Similarly, in the below statement, the compiler calls method 4
System.out.println(demo.add(2,3.4));
// Similarly, in the below statement, the compiler calls method 3
System.out.println(demo.add(2.5,3));
}
}
In runtime polymorphism, the runtime or the execution stage determines the actual implementation of the function.
Example:
class AnyVehicle{
public void move(){
System.out.println(“Any vehicle should move!!”);
}
}
class Bike extends AnyVehicle{
public void move(){
System.out.println(“Bike can move too!!”);
}
}
class Test{
public static void main(String[] args){
AnyVehicle vehicle = new Bike();
// In the above statement, as you can see, the object vehicle is of type AnyVehicle
// But the output of the below statement will be “Bike can move too!!”,
// because the actual implementation of object ‘vehicle’ is decided during runtime vehicle.move();
vehicle = new AnyVehicle();
// Now, the output of the below statement will be “Any vehicle should move!!”,
vehicle.move();
}
}
13. Does C++ support Polymorphism?
Yes, C++ is an object-oriented programming language and supports both compile time and runtime polymorphism.
14. What is meant by Inheritance? Give an example.
In terms of OOP, inheritance is a mechanism that uses the definition of another object or class (referred to as a parent) to create an object or class (referred to as a child). Inheritance helps keep the implementation simpler and facilitates code reuse.
15. What are the limitations of Inheritance?
Inheritance has to navigate through multiple classes for implementation and hence, takes more time to process.
The base class and the child class are tightly coupled. As a result, changes must be made in the form of nested changes in both classes, which can lead to incorrect outputs if not done correctly.
16. What are the various types of inheritance?
- Single inheritance
- Multiple inheritances
- Hybrid inheritance
- Multi-level inheritance
- Hierarchical inheritance
17. What is a subclass?
A subclass is an entity and a part of Inheritance. It is also known as child class and inherits from another class.
18. What is a superclass?
A superclass is an entity that allows subclasses or child classes to inherit from itself. It is also a part of inheritance.
19. What is Abstraction? How can it be achieved?
Software users want to primarily know how the software they use solves problems. Abstraction is a method that allows programmers to hide unnecessary elements and only make the necessary details visible to users. Data abstraction can be achieved through abstract class and abstract method.
20. Do classes occupy memory?
Classes are merely a blueprint that help create objects, so they do not occupy memory. However, objects consume memory upon creation as they initialize the class members and methods.
21. Is it always necessary to create objects from class?
If the base class has non-static methods, it is necessary to create an object from a class. But if the class has static methods, there is no need to create an object as the class method can be called directly using the class name.
22. What purpose does a constructor serve?
Constructors initialize objects. Here is an example of a class with the class name “MyClass.” To instantiate this class, you can use the syntax:
Constructors initialize objects. Here is an example of a class with the class name “MyClass.” To instantiate this class, you can use the syntax:
MyClass myClassObject = new MyClass();
23. Explain the various types of constructors in C++.
Default constructor: It has no parameters and takes no arguments.
class ABC
{
int x;
ABC()
{
x = 0;
}
}
Parameterized constructor: It takes arguments only in the form of parameterized constructors.
class ABC
{
int x;
ABC(int y)
{
x = y;
}
}
Copy constructor: It is a member function that uses another object of the same class to initialize an object.
class ABC
{
int x;
ABC(int y)
{
x = y;
}
// Copy constructor
ABC(ABC abc)
{
x = abc.x;
}
}
24. What is a destructor?
A destructor frees up memory and resources taken by an object. It is called when an object is being destroyed.
25. What is the difference between a class and a structure?
A class is saved in the heap memory, while a structure is saved in the stack memory. Also, structure helps achieve data abstraction.
26. What is an interface?
In the context of OOP, an interface is a concept that lets you declare methods without defining them. Interfaces do not have detailed actions or instructions to be performed. The methods of the interface can be defined by any class that implements an interface.
27. What is meant by static polymorphism?
Static polymorphism is another name for compile–time polymorphism.
28. What is meant by dynamic polymorphism?
Dynamic polymorphism is another name for runtime polymorphism.
29. What is the difference between overloading and overriding?
Overloading is a feature of compile-time polymorphism feature. Here, an entity has multiple implementations with the same name. Method overloading and operator overloading are examples of overloading.
Overriding is a feature of runtime polymorphism feature. Here too, an entity has the same name, but its implementation changes during execution. An example is method overriding.
30. Can we overload the constructor in a class?
Yes, we can overload the constructor in a class. Parameterized constructor, default constructor, and copy constructor are forms of overloaded constructor.
31. Can we overload the destructor in a class?
A destructor cannot be overloaded in a class. There can only be a single destructor in a class.
32. What is the virtual function?
The virtual function overrides methods of the parent class in the derived class. It provides abstraction in a class.
It is declared using the virtual keyword in C++.
class base {
virtual void print()
{
cout << "This is a virtual function";
}
}
Every public, non-static, and non-final method in Java is a virtual keyword.
class base {
void func()
{
System.out.printIn("This is a virtual function")
}
}
In Python, methods are generally virtual.
class base:
def func(self):
print("This is a virtual function")
33. What is pure virtual function?
An abstract function is also known as a pure virtual function. It is a member function without any statements. If needed, this function can be defined in the derived class.
Examples:
C++
class base {
virtual void pureVirFunc() = 0;
}
Java
abstract class base {
abstract void prVirFunc();
}
In Python, pure abstract function is achieved with @abstractmethod from the ABC module.
34. What is an abstract class?
A class that has abstract methods is called abstract class. Abstract methods need to be exclusively defined in a subclass for use, since they are not defined but declared.
35. List any one difference between abstract class and an interface.
Both are special types of classes. When an abstract class is inherited, it is fine if the subclass does not supply the definition of the abstract method. However, this is okay only until and unless the subclass actually uses it.
When an interface is implemented, the subclass has to specify all the methods of the interface, along with their implementation.
36. What is the significance of access specifiers?
Access specifiers are keywords that control or specify the accessibility of classes, methods, etc. Some access specifiers or access modifiers include private or public aspects and play an important role in encapsulation.
37. What is the difference between public, private and protected access modifiers?
Name | Accessibility from derived class | Accessibility from own class | Accessibility from world |
---|---|---|---|
Public | yes | yes | yes |
Private | no | yes | no |
Protected | yes | yes | no |
38. What is an exception?
An exception is a notification that interrupts the normal execution of a program. With the help of exceptions, it is possible to carve a pattern for an error and transfer it to the exception handler so that it can be resolved. As soon as an exception is raised, the state of the program is saved.
39. What is meant by exception handling?
In OOP, exception handling is a concept that helps with error management. Errors are thrown up and caught by an exception handler. It also implements a centralized mechanism to resolve the errors.
40. How is an error different from an exception?
An exception is a condition that might be caught by an application. However, an error is a problem that an application should not come across.
41. What is a try/catch block?
It is used to handle exceptions. A try block defines a set of statements that may lead to an error. The catch block catches the exception.
42. What is a finally block?
In programing, a finally block is a code that is run after the try and any catch block before the method is completed. Irrespective of the exception being thrown or caught, the finally block is executed.
43. What is Garbage Collection in terms of OOPs?
In the context of OOPs, Garbage Collection helps with memory management in a program. Garbage Collector removes objects that are not needed, thereby freeing up space.
44. Can a Java application be run without implementing the OOPs concept?
Java applications are based on OOP models and concepts and, therefore, can’t be implemented without OOP. However, C++ can be implemented without OOPs.
45. Write an output to this code.
Input:
#include<iostream>
using namespace std;
class BaseClass1 {
public:
BaseClass1()
{ cout << " BaseClass1 constructor called" << endl; }
};
class BaseClass2 {
public:
BaseClass2()
{ cout << "BaseClass2 constructor called" << endl; }
};
class DerivedClass: public BaseClass1, public BaseClass2 {
public:
DerivedClass()
{ cout << "DerivedClass constructor called" << endl; }
};
int main()
{
DerivedClass derived_class;
return 0;
}
Output:
BaseClass1 constructor called
BaseClass2 constructor called
DerivedClass constructor called
46. Write an output of this code.
Input:
class Scaler
{
static int i;
static
{
System.out.println(“a”);
i = 100;
}
}
public class StaticBlock
{
static
{
System.out.println(“b”);
}
public static void main(String[] args)
{
System.out.println(“c”);
System.out.println(Scaler.i);
}
}
Output:
b
c
a
100
47. What will be the output of the following code?
#include<iostream>
using namespace std;
class ClassA {
public:
ClassA(int ii = 0) : i(ii) {}
void show() { cout << "i = " << i << endl;}
private:
int i;
};
class ClassB {
public:
ClassB(int xx) : x(xx) {}
operator ClassA() const { return ClassA(x); }
private:
int x;
};
void g(ClassA a)
{ a.show(); }
int main() {
ClassB b(10);
g(b);
g(20);
getchar();
return 0;
}
Output:
i = 10
i = 20
48. What will be the output of the following program?
Input:
#include<iostream>
using namespace std;
class A {
public:
void print()
{ cout <<" Inside A::"; }
};
class B : public A {
public:
void print()
{ cout <<" Inside B"; }
};
class C: public B {
};
int main(void)
{
C c;
c.print();
return 0;
}
Output:
Inside B
49. What will be the output of the following code?
#include<iostream>
using namespace std;
class BaseClass{
int arr[10];
};
class DerivedBaseClass1: public BaseClass { };
class DerivedBaseClass2: public BaseClass { };
class DerivedClass: public DerivedBaseClass1, public DerivedBaseClass2{};
int main(void)
{
cout<<sizeof(DerivedClass);
return 0;
}
Output:
If the size of the integer is 4 bytes, then the output will be 80.
50. What will be the output of the following code?
public class Demo{
public static void main(String[] arr){
System.out.println(“Main1”);
}
public static void main(String arr){
System.out.println(“Main2”);
}
}
Output:
Main1

Conclusion
OOP plays a crucial role in structuring a code, and therefore, a good command over object-oriented programming is a key to successfully clearing OOP interviews. The key is to begin with a good grasp of concepts such as encapsulation, inheritance, polymorphism, data abstraction, objects, and class. Next, it is important to practice writing code related to OOP. Our compilation of questions is meant to help you get a good overview of the types of questions asked. However, this is not an exhaustive list and should be complemented with continuous learning. All the best with your interviews!
Take control of your career and land your dream job
sign up with us now and start applying for the best opportunities!
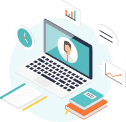
FAQs
The four main concepts to remember are abstraction, encapsulation, polymorphism, and inheritance.
OOP is easy to maintain as it allows you to create reusable classes of objects that can be used to create multiple instances of that object.
Online shopping platforms are an example of a real-life application of OOP. The shopping platform can use classes to represent different entities, such as customers, orders, shopping carts, and products.
A simple example of OOP is thinking of a car with a model name, color, and the year when it was manufactured. Here, the car would become an object and its name, engine, color, etc., will become attributes.