Table of Contents
Toggle
Introduction
We all keep hearing the term “data” all the time: big data, cloud data, data science, and so on. But have you ever wondered what exactly a data structure is? Well, in computer science, data structure is an organizational tool that helps to manage, organize, and store information efficiently. It is one of the important topics in computer science and in this article, we learn about the data structure and algorithm in detail.

What is Data Structure?
A data structure is a way to organize a huge collection of data in a particular order in the computer world. It is a technique to study how the data are logically correlated with each other. In addition, data structure enhances the program’s performance and minimizes memory requirements during runtime.

Classification of Data Structure
A data structure is classified in six different ways:
- Linear
- Non-linear
- Homogenous
- Non-homogenous
- Static
- Dynamic

Linear and Non-linear Data Structure
In the linear data structure, data is stored in a sequence in memory. There are 4 types of linear structure:
- Array
- Stack
- Linked List
- Queue
- Let’s have a look at its differences in the table below.
Factors | Array | Linked List |
---|---|---|
Definition | It is a collection of homogenous data types. | It is a collection of nodes, i.e., data and its addresses. |
Type of Data Structure | It works with static data structures, which means memory cannot be changed during runtime. | It works with dynamic structures and uses the concept of pointer. |
Memory Storage | Elements are stored in the continuous memory location. | It stores elements anywhere in the memory. |
Independent/Dependent | Independent because its elements are stored in random or different memory. We can take any element and print it. | Dependent because it works by passing the address of one element to another. |
Performance | It takes more time to carry out insertion and deletion operations. | It takes less time than the array. |
Whereas non-linear data structure is the opposite of linear as it stores data in a random memory. The following are the two types of non-linear data structure:
- Tree
- Graph
Factors | Tree | Graph |
---|---|---|
Definition | It is a collection of nodes and edges. T= {node, edges} | It is a collection of vertices and edges. G= {V, E} |
Type of Data Structure | It works with static data structures, which means memory cannot be changed during runtime. | It works with dynamic structures and uses the concept of pointer. |
Model | Hierarchal | Network |
Linear/Non-linear | Non-linear | Non-linear |
Edges | N-1 edges | It contains multiple edges. |
Loop | It does not have a loop. | We can perform loops in graphs. |
Application | It is used to make decisions for searching, deleting, and inserting. | A graph is used to find the shortest path. |

Homogenous and Non-homogenous Data Structure
Homogenous data structure deal with similar kinds of data, and this type of data structure works only with “arrays.”
Non-homogenous data structure work with different kinds of data in two different ways, i.e., union and structure.

Static and Dynamic Data Structure
The final classification of data structure is static and dynamic. The static data structure allows the programmer to work only with a fixed size of memory, and it is not possible to change the value in an array once the value is assigned.
On the other hand, using a dynamic data structure, programmers can manage the memory during runtime according to their requirements; they can increase and decrease it as well. Therefore, these are the classifications of data structure.
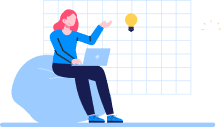
Don't miss out on your chance to work with the best
apply for top global job opportunities today!

What are the Operations of Data Structure?
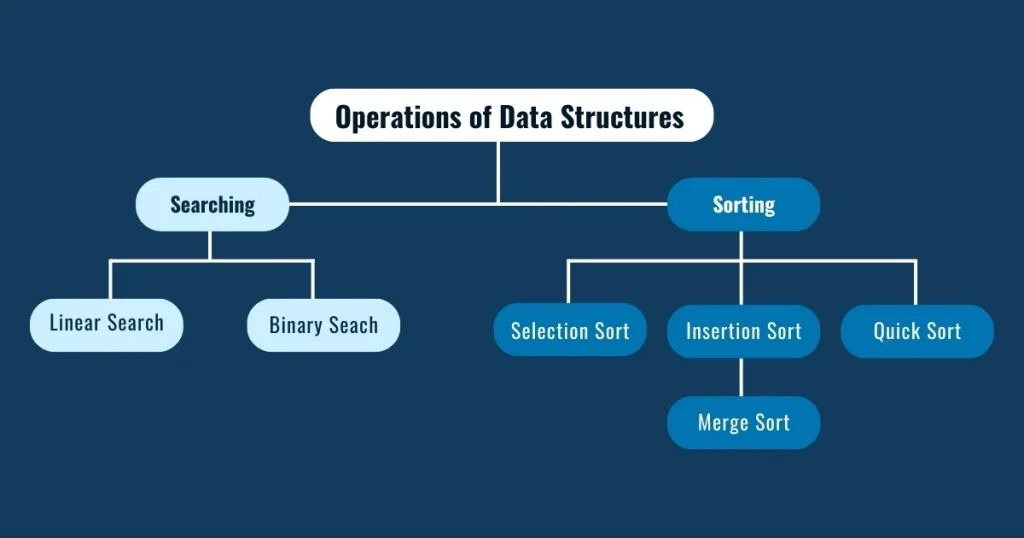
Data structure can perform the following operations. Now let’s learn about each in detail.
- Searching: It is a technique or method to find elements along with their location in data structure. This search is of two types:
- Linear Search: In this type of searching, we find the element in an array by traversing the array from the starting point to the point where the element is not found.
Example of Linear Search Program:
int main ()
{
int item, i=0;
int a[5]={22, 34, 12, 40, 79};
printf(“Enter the item to search: ”);
scanf(“%d”, &item);
while (i<5)
{
if (a[i]==item);
printf(‘’Found the search item %d”, i);
exit(0);
}
++i
}
if (i>=5)
{
printf(“Could not find the search item”)
exit(0);
}
return 0;
}
The above program will ask you to enter the input, and according to that, it will search for the item. If you put the input value that is not assigned in the array value, it will display the output “that the item could not find.”. So, this is how linear search works and operates.
- Binary Search: Unlike linear search, in binary search, we need to apply the divide and conquer searching technique. First, we need to arrange the data in one order, and then we need to find the middle element of the array and compare it with the target element. Let’s say you have the elements in this form,
so first, you need to arrange them in ascending order. let’s see this in a program:
int main ()
{
int a[5]={10, 12, 30, 50, 89};
int lr=0, up=4, mid, item, f=0;
printf(“Enter the item that you want to search: ”);
scanf(“%d”, &item);
while (lr<=up)
{
mid=(lr+up)/2;
if (a[mid]==item)
{
f=1; break;
}
if(a [mid]<item)
{
else
{
up=mid-1;
}
}
if (f==1)
{
printf(“Found the item that you were searching at %d”, mid);
}
else
{
printf(“Didn’t found the item that you were searching”);
return 0;
}
In the above program, we first arranged the elements in the ascending order in the array and wrote the program in binary search. Now, according to the input of the user, it will give the output. A binary search is used whenever there is tons of data in an array list. In contrast to linear search, it is much faster and is commonly used along with a linked list.
Must Read: Difference between SQL vs. NoSQL Database
2. Sorting: It is a technique to arrange the elements in ascending order or descending order. It is of five types; let’s have a look at it:
- Bubble Sort: Bubble sort is an algorithm to arrange n numbers of elements in the correct position. This type of algorithm works by evaluating whether each adjacent element is in proper order or not, and if not, it switches its positions. This algorithm goes on until the last two elements need to be swapped. The bubble sort algorithm looks like the following picture:
After sorting we got,
- Selection Sort: This type of sorting targets the smallest item in the array and arranges it in proper order. The selection sort looks like the following in the picture:
Finally, our selection sort looks like this:
- Insertion Sort: This type of sorting algorithm arranges the n-th array by transferring the right position one at a time. The array looks like this:
- Quick Sort: This type of algorithm separates the list of elements into two different parts and then sorts each set of elements repeatedly. Every partition of a list is performed based on a pivot element. So, the left side consists of all the smaller items, and the left side contains all the greater elements.
- Merge Sort: This type of algorithm uses the divide-and-conquer technique and then splits the algorithm into smaller chunks and then sorts it. Each group then merges and comes down to the final sorted sequences.

What is an Algorithm ?
An algorithm is a sequence of steps or sets of rules that tell a computer how to perform a specific task. While this data structure and algorithm work simultaneously, these two go hand in hand and make the computer process things efficiently. Data structure take care of organizing and managing tasks, and algorithmic step-by-step instructions guide the data structure to perform the particular task correctly. Before writing any program, a programmer always designs the algorithm first. To better understand the algorithm concept, we will show a simple algorithm of printing of natural numbers:
Step1: Start
Step 2: Set i=1.
Step 3: Repeat step 4 and 5 while i<=10
Step 4: print i
Step 5: Exit

What is the Key Role of Algorithm Complexity in Data Structure?
Algorithm Complexity analyzes the performance of an algorithm in data structure through time and space. Space complexity is to define the total memory that is required by one particular algorithm to complete its execution. Time complexity tells how much time one algorithm takes for its execution.

Final Thoughts - Why is Data Structure Important?
Data Structure facilitates efficient data storage and retrieval, enabling programmers to manage large volumes of data effectively while optimizing algorithms for tasks like searching and sorting. By choosing the appropriate data structure, developers can optimize resource utilization, minimizing memory and processing overhead. Moreover, understanding data structure enables programmers to analyze problems effectively, select suitable solution strategies, and build scalable software systems across various domains, laying the groundwork for advanced concepts like databases, network protocols, and artificial intelligence. In essence, data structures serve as the backbone of modern computing, enabling the creation of efficient and robust software solutions.
Take control of your career and land your dream job
sign up with us now and start applying for the best opportunities!
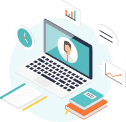

Frequently Asked Questions
If you have a good grasp of the concept of data structure, then you won’t have any difficulty arranging, modifying, accessing, and storing them in less time. Your capabilities in finding out and mapping the dots between the various data points will become strong.
It lets you store and manipulate data in various data structures for different tasks. Thus, the right selection of data structure plays a critical role in managing any software.
Some of the real-world examples of queues are a call center phone system printer and routers, or switches, to manage the packet flow.
Graphs are widely used by social networking sites and web pages.