Table of Contents
Toggle
Introduction
JSON is a file format used to exchange or share data across different systems or applications. Well, there are various ways in which we can transmit data, either by using XML or CSV, but JSON is gaining popularity and is way simpler than XML and CSV. So, if you’re venturing into the software development world and are unaware of where to use JSON, you have come to the right place. Today, this article will cover what JSON is.

What is JSON?
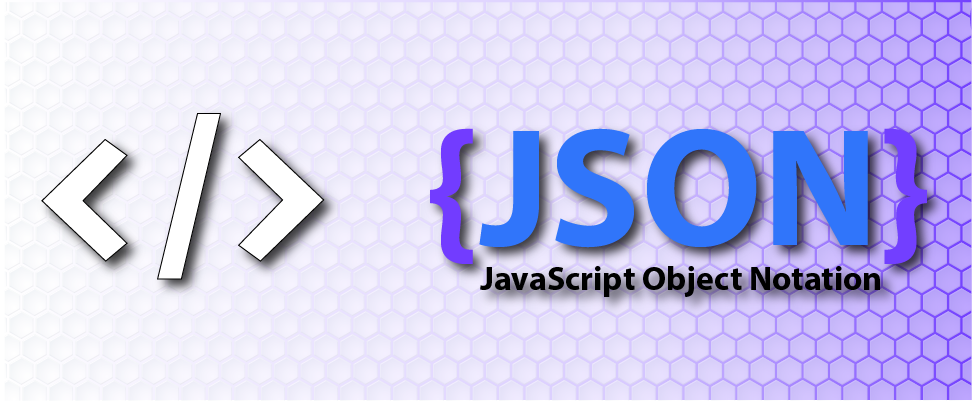
JSON stands for JavaScript Object Notation. It is popular for lightweight text-based formats to store structured data. This data is further assembled, parsed, and generated by JavaScript and other programming languages such as C, C++, C#, Java, JavaScript, Perl, and Python. Moreover, it’s one of the most common methods to send and receive data over the Web while using Web APIs.
This is how the JSON data looks like:
{
"name": Ansel,
"age": 29,
"married": false,
"hobbies": ["Singing," "Traveling," “Photography"]
}

What is the Role of API in JSON?
An API is a set of rules that enable seamless interaction between software. In simple words, it acts like a middleman between the computer and the JSON server. Let’s say you want to know about the weather. The JSON server will host the data you want to access, and the API will return it in JSON format. APIs minimize the burden of developing software by offloading some of the tasks to another server.

Why is JSON Important?
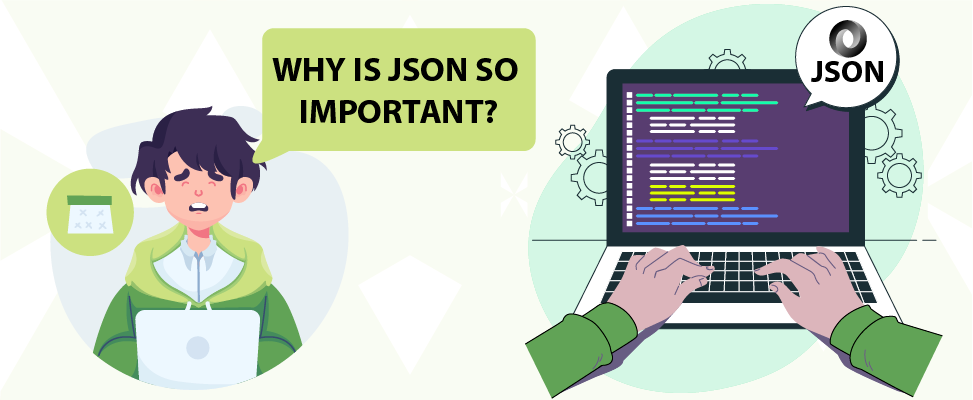
When AJAX was introduced, it offered the convenience of reading data right away once the web pages loaded on the web server. Also, updating the page was done without reloading it and sending data to a web server in the background. Therefore, it was essential for websites to load data more quickly and asynchronously without any delay in rendering. In this regard, JSON has everything from faster accessibility to memory optimization. Due to this, JSON is widely used and plays a pivotal role in modern-day web applications. Hence, it is often used with AJAX.

JSON Syntax
Its syntax is simple in a text format that is easy to read for both humans and machines. Before we delve into its syntax, we need to consider a few important rules of JSON syntax. First and foremost, while storing files in JSON format, it’s important to remember that the file extension always ends with “.json.”. Second, when creating JSON data, there are several rules for how data types need to be structured. JSON uses strings, numbers, Boolean values, arrays, null statements, and objects for data types. Let’s see the rules in detail below:
Key/value pair: A key is always a string enclosed in quotation marks, and the value must be one of the six JSON value types; those are
- A string that consists of characters in quotation marks.
- Number: It consists of 123, 6.789, and -2.
- Object: It is a collection of key-value pairs contained in curly brackets.
- Array: It consists of values in an ordered list that are separated by a comma.
- Boolean: It’s a true or false statement.
- Null: It is to denote empty and undefined data.
Key/value pairs must have double quotes around the string values.
"job role": "software developer"
It must be separated by a semicolon.
"name": "Sophie"
If you’re using multiple key/value pairs, those pairs must be separated by a comma at the end of the line.
"age":30,
"name":Sophie,
"job role": "software developer",
When using objects, they must be enclosed by curly brackets.
{
"color":"purple",
"food":"burger",
}
Arrays must be enclosed in square brackets, and the items inside the array must be separated by a comma.
"ice cream":["vanila","choclate","hazelnut"]
Another example of an array with square brackets;
"employees": [
{"firstName: "Harry", "lastName: "Smith"}
{"firstName: "Joseph, "lastName: "Paul"}
{"firstName: "Peter", "lastName: "Brown"}
{"firstName: "John", "lastName: "Green"}
]
Data is in name/value pairs; data is separated by commas, and curly braces hold the objects.
{"firstName: "Harry", "lastName: "Smith"}
Now let’s create one JSON file that contains different types of data:
{
"name" : "Ansel"
"age" : 29,
"isEmployee" : false,
"address" : {
"street" : "24 Avenue St",
"city" : "New York",
"postalCode": "10014"
),
"hobbies" : [" Singing, "traveling", "photography"],
"hasPets" : null
]
In this code above, we create a person named Ansel, with an age 29, whose boolean value of isEmployee is set to false. And we have the key/value pairs of streets, city, and postal with an address object. Similarly, we have created an array of hobbies and a key that is set to null because it’s unclear whether this person owns the pets or not.

How to Create a JSON File?
You can give any name of your choice and name the file, and then save it with this file extension, “.json.”. For example, “myfile.json” should be the file extension. Once done, start by creating the data of the object that you want directly in your newly created JSON file.
{
"firstName":"Abbey",
"LastName":"Brown",
"occupation": "UI Designer",
"Hobbies": ["Reading", "Coding", "Football", "Acting", "Singing"].
"married": false
"Age": 22
}
This file is to store the information of a person with a `first name` of `Abbey` together with his `last name` and other information. Similarly, we can do this in the array object syntax using square brackets. If you want to know how to open a JSON file on different operating systems, like Windows, iMac, and Linux, then have a look at the following:
For Windows:
- Microsoft Notepad
- Altova XMLSpy
- Mozilla Firefox
- Notepad
- File Viewer Plus
- Notepad++
- Microsoft WordPad
For Linux:
- Vim
- GNU Emacs
- GitHub Atom (cross-platform)
- Mozilla Firefox, and
- Google Chrome
For iMac:
- Apple TextEdit
- Mozilla Firefox
- Bare Bones Text Wrangler

Static Methods
JSON consists of two static methods, which are
- JSON.stringify()
- JSON.parse()
These two static methods are used based on the environment that the individual is working in. Sometimes, JSON files need to be parsed with functions in a new environment. This means it needs to be converted into a string or an object.
JSON.parse(): It is used in a JavaScript environment and converts the JSON object into a string. To do this, we can use a built-in method called parse, which parses and converts data into an object. Then, we can show this on the website, convert it further into another object, update some data, or delete something from it.
{
"name" : "Ansel"
"age" : 29,
"isEmployee" : false,
"address" : {
"street" : "24 Avenue St",
"city" : "New York",
"postalCode": "10014"
),
"hobbies" : [" Singing, "traveling", "photography"],
"hasPets" : null
]
const dataObject = JSON.parse(data);
console.log(dataObject);
In the above code example, we have used the JSON file for different data types earlier. Here, we convert it to a usable object. The data object returns a `prototype` of `Object`.
JSON.stringify():This static method is the reverse of `JSON.parse(). It converts the JSON string into an object. To convert this, we need to use another method, stringify, that converts an object to JSON. This is done whenever we want to send the data back to the API. Another use case is local Storage or session storage, where JSON is used. We will use the same code example and convert it to JSON.
{
"name" : "Ansel"
"age" : 29,
"isEmployee" : false,
"address" : {
"street" : "24 Avenue St",
"city" : "New York",
"postalCode": "10014"
),
"hobbies" : [" Singing, "traveling", "photography"],
"hasPets" : null
]
const data = JSON.stringify(dataObject);
console.log(data)
This will display the data of this person named Ansel and convert its object into a JSON string.
JSON with HTML: You need to create an index.html file and an app.js file. The index file will contain all the data, and the app files will display the functionality of this data.
<! DOCTYPE html>
<html lang=”en”>
<head>
<meta charset=”UTF-8”>
<meta name=”viewport” initial-scale=”1.0”>
<title> Display JSON</title>
</head>
<h1> Display JSON</h1>
<section id=”data-container”>
</section>
<script src-=”app.js”> </script>
</body>
</html>
app.js
const dataJSON = ‘ [
{
"name" : "Amber"
"age" : 20,
"isStudent" : true,
"address" : {
"street" : "Acorn Street",
"city" : "Boston",
"postalCode": "02112"
),
"hobbies" : [" Gardening, "Reading", "photography"],
"hasPets" : dog
},
{
"name" : "John"
"age" : 30,
"isStudent" : false,
"address" : {
"street" : "Hollywood Boulevard",
"city" : "Los Angelos",
"postalCode": "90009"
),
"hobbies" : [" Hiking, "Sports", "Gaming"],
"hasPets" : fish
}
] ‘ ;
const data =JSON.parse(dataJSON);
console.log(data);
As you can see, we have created two files, and in the app.js file, we have used the parse method to convert the JSON data into a JavaScript object so that we can easily work with it. Now we need to show this data in an HTML file. For that, we need to create some elements that can hold this data and display all of it in the section. To show this, we need to use the id of this element and, in the JavaScript, create a reference to this section.
cont sectionElement = document.getElementById(“data-container”);
console.log(sectionElement);
const ul = document.createElement(“ul”);
data.forEach(person => {
const li =document.createElement(‘’li”);
for (const key in person){
….
}
})
data.forEach (person=>
{
const li =document.createElement(“li”);
for (const key in person)
{
if (Array. isArray(person[key]))
{
li.innerHTML +=’$(key): ${person [key].joint (‘,’)}
}
<br>’;
}
else if typeof person[key]== “object”)
{
for (const subkey in person[key])
li.innerHTML +=’$(key): ${person [key] [subKey]}
}
<br>
}
}
else {
li.innerHTML += ‘${key} - ${person[key]}
<br>’;
}
}
ul.appenChild(li)
})
In the above example code, we use an id for the element and create a reference in JavaScript. Then we create elements for each person’s data in the JSON in an unordered list. We used the array using for Each, and then we will use the for loop to iterate over the keys of an object for each person. To display the data properly, for each key, we will have to work with some values differently. Finally, we have set the values using innerHTML, so now the HTML page will display a list of all the keys and values.

JSON vs. XML
XML, which stands for Extensible Markup Language, is another popular data interchange format. Its syntax is like HTML, follows hierarchy, and includes many complex nested tags. Whereas JSON syntax refers to JavaScript objects, while working with objects, arrays, APIs, and configuration files, JSON is mostly used. XML is used for complex data interchange, structure, and scientific data. However, XML sync is more complex because for every opening tag, there need to be extra closing tags. Thus, it makes the text long and hard to read. Whereas JSON is considered readable and simple for both humans and machines.

JSON vs. CSV
CSV stands for comma-separated values and is another popular data interchange format. In contrast to JSON, CSV is considered more plain and uses plain text with several lines. CSV is not used for objects and arrays; it is rather more suitable for flat data. Most commonly, CSV is used for tabular data that is organized in rows and columns. It is not the best choice for complex and nested data. Though CSV may seem complex to some, when there are a lot of rows and columns, it might be a bit harder to read.
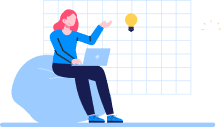
Don't miss out on your chance to work with the best!
Apply for top job opportunities today!

Why use JSON ?
JSON shares similarities with JavaScript object literals, but it is more like a text format. It is a good choice for absolute beginners who have just started their software development journey. Learning JSON methods like parsing and syntax can help you achieve effective data exchange and manipulation. As a language, JSON is independent and can parse and generate JSON data in other programming languages. Another compelling feature is its lightweight format, which allows users to share, store, and work with data. These days, JSON is getting support in APIs, such as Twitter API. It is a perfect alternative to XML, easier to parse, and quicker to read and write. All in all, JSON is easy for data interchanging, and creating APIs is much easier than XML.
FAQs
JSON is used for transmitting data between the server and the client. It is also used to store unstructured data and exchange data between systems.
No, it’s not. It is a data-interchange format and a subset of JavaScript. JSON can execute logic and operate like other programming languages.
JSON can seamlessly handle unstructured, complex data which is not possible using CSV format. Also, representing the hierarchical data using JSON is pretty simple and more versatile than XML and CSV formats.
It doesn’t have the date element to record calendar-style entries. Also, JSON cannot distinguish between floating-point fractional numbers and decimals, which can be a problem for some. There are security issues with the parser.
JSON Path Finder helps navigate the JSON query in JSON documents. It is extremely beneficial when dealing with large and complex data objects.
Yes, we can convert JSON to Dart objects using the tool called Quicktype. It’s simple interface allows users to convert JSON to Dart easily. By going to its web site, simply paste the JSON data in the JSON input box and select the ‘Dart’ option. That’s it, and then your JSON data will be converted into the Dart code.
Take control of your career and land your dream job!
Sign up and start applying for the best opportunities!
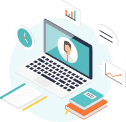