Table of Contents
Toggle
Introduction
In computer programming, data structures help you organize, store, and manipulate data efficiently. Data structures such as arrays, lists, graphs, queues, and stacks are the fundamental components of computer programming. Programmers who seek to acquire expertise in their field must know these data structures well so that they can build on their knowledge. In this blog, we will understand arrays and their types, advantages and disadvantages of arrays, and their real-life applications.

What is an Array?
In computer programming, an array is a data structure that stores equivalent data elements at adjacent memory locations. Arrays are data structures that hold values and can be accessed by indexes.
Here is how an array looks in a code.
int[] numbers = {1, 2, 3, 4, 5};
It is an array called ‘numbers’ and holds five integers. The first integer ‘1’ is at position or index 0, the second integer ‘2’ at index 1, and so on.
An array stores its elements in contiguous memory locations. In simple words, an array helps you locate and identify each stored piece of data or element. This is done by adding an offset to each value. Arrays are used in almost every program and are one of the oldest and most important data structures. They are also used to implement other data structures, like strings and lists.
Basic Operations Supported by Arrays
Traversal: Print the array elements one by one
Insertion: Add an element at the specified index
Deletion: Delete an element at the specified index
Searching: Use the given index or value to search for an element
Sorting: Maintain the order of elements in an array

Types of Arrays
One-Dimensional
A one-dimensional array contains a single row of elements. It is generally indexed from 0 to n-1, where ‘n’ is the size of the array. Each element in an array can be accessed easily by using the index number assigned to it.
The syntax of a one-dimensional array in C programming language is as follows:
dataType arrayName[arraySize];
Example of a simple one-dimensional array in C:
Input
#include <stdio.h>
int main() {
int numbers[5] = {10, 20, 30, 40, 50};
for(int i=0; i<5; i++) {
printf("numbers[%d] = %d\n", i, numbers[i]);
}
return 0;
}
Output
numbers[0] = 10
numbers[1] = 20
numbers[2] = 30
numbers[3] = 40
numbers[4] = 50
Two-Dimensional
Two-dimensional arrays are also known as matrix arrays, and they contain arrays of elements. Think of them as a grid that lays out the elements into rows and columns. You can access each element within the two-dimensional array individually by its row and column location. Two-dimensional arrays are used for storing data where each element may have multiple associated values, for example, tables or pictures.
The syntax to declare a 2D Array in C programming language is as follows:
data_type array_name[row_size][column_size];
Example of a two-dimensional array in C
Input
#include<stdio.h>
int main(){
int i=0,j=0;
int arr[4][3]={{1,2,3},{2,3,4},{3,4,5},{4,5,6}};
//traversing 2D array
for(i=0;i<4;i++){
for(j=0;j<3;j++){
printf("arr[%d] [%d] = %d \n",i,j,arr[i][j]);
}//end of j
}//end of i
return 0;
}
Output
arr[0][0] = 1
arr[0][1] = 2
arr[0][2] = 3
arr[1][0] = 2
arr[1][1] = 3
arr[1][2] = 4
arr[2][0] = 3
arr[2][1] = 4
arr[2][2] = 5
arr[3][0] = 4
arr[3][1] = 5
arr[3][2] = 6
Multi-Dimensional
Multi-Dimensional arrays, also referred to as N-dimensional arrays, contain more than one array arranged hierarchically. They are used to store and manage data organizationally. Multi-dimensional arrays can have many dimensions. Rows and columns are the most common, although three or more dimensions can also be used.
Here is the syntax for a multi-dimensional array in C:
data_type array_name[size1][size2]....[sizeN];
Here is an example of using multi-dimensional arrays in C, where the program stores the grades of 5 students in 4 different subjects.
Input
#include <stdio.h>
int main() {
int grades[5][4];
// Prompt user to enter grades
for (int i = 0; i< 5; i++) {
printf("Enter grades for student %d:\n", i+1);
for (int j = 0; j < 4; j++) {
printf("Subject %d: ", j+1);
scanf("%d", &grades[i][j]);
}
}
// Calculate average grade for each student
printf("\nAverage grade for each student:\n");
for (int i = 0; i< 5; i++) {
float sum = 0;
for (int j = 0; j < 4; j++) {
sum += grades[i][j];
}
float avg = sum / 4;
printf("Student %d: %.2f\n", i+1, avg);
}
// Calculate average grade for each subject
printf("\nAverage grade for each subject:\n");
for (int j = 0; j < 4; j++) {
float sum = 0;
for (int i = 0; i< 5; i++) {
sum += grades[i][j];
}
float avg = sum / 5;
printf("Subject %d: %.2f\n", j+1, avg);
}
return 0;
}
Output
Enter grades for student 1:
Subject 1: 80
Subject 2: 75
Subject 3: 90
Subject 4: 85
Enter grades for student 2:
Subject 1: 70
Subject 2: 85
Subject 3: 80
Subject 4: 75
Enter grades for student 3:
Subject 1: 90
Subject 2: 80
Subject 3: 85
Subject 4: 95
Enter grades for student 4:
Subject 1: 75
Subject 2: 90
Subject 3: 75
Subject 4: 80
Enter grades for student 5:
Subject 1: 85
Subject 2: 70
Subject 3: 80
Subject 4: 90
Average grade for each student:
Student 1: 82.50
Student 2: 77.50
Student 3: 87.50
Student 4: 80.00
Student 5: 81.25
Average grade for each subject:
Subject 1: 80.00
Subject 2: 80.00
Subject 3: 82.00
Subject 4: 85.00

Advantages of Arrays
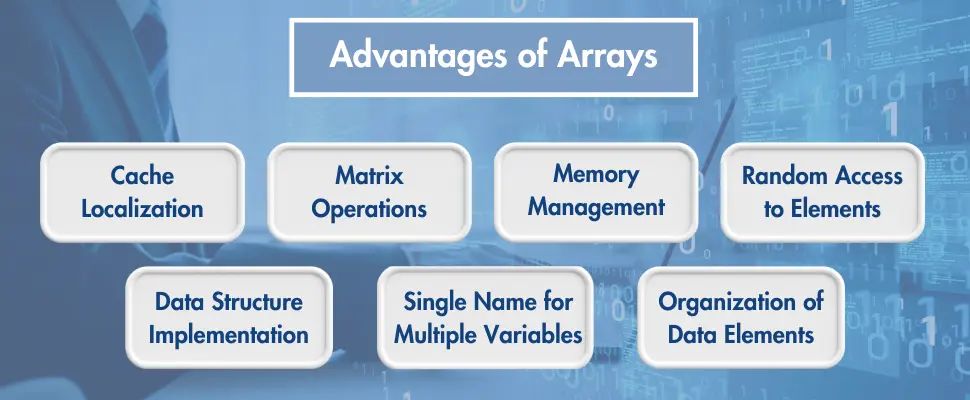
Cache Localization: Arrays can speed up your work as they can provide specific descriptions of reference locations within the data. This offers programmers more efficient cache localities.
Matrix Operations: Arrays can be used by small and large databases to perform matrix operations like addition and multiplication.
Memory Management: Variables are sorted under a single name by arrays, which optimizes memory management. For example, a program can create a new variable for each element entered. However, it would take time to locate items. So, search engines often use arrays to store and sort data.
Random Access to Elements: Data structures have sequential access, as a result of which you can only access the values in a specific order. With arrays, it is possible to access the elements randomly, which means position elements can be accessed more efficiently compared to data structures.
Data Structure Implementation: Arrays can be used by programmers and software developers to implement other data structures, such as hash tables, heaps, stacks, and queues.
Single Name for Multiple Variables: An array makes it easy for a software designer to organize multiple variables. Arrays make it easier to maintain large sets of data under a single variable name. With this, you can avoid any confusion that can come with multiple variables.
Organization of Data Elements: Arrays offer different algorithms that help with the organization of various data elements. For example, bubble sort, selection sort and insertion sort help programmers organize data efficiently and clearly.

Disadvantages of Arrays
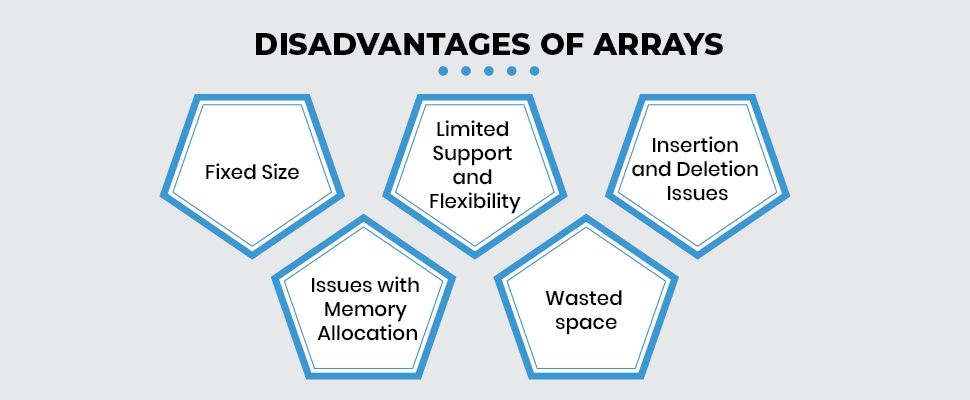
Fixed Size: The sizes of arrays are determined during their creation. If the size needs to be increased, you have to create a new array where the data is copied from the old array to the new one. This is a time-consuming and memory-intensive process.
Limited Support and Flexibility: Arrays must be of the same data type. Complex data types like objects and structures get limited support from arrays. As a result, compared to linked lists and trees, arrays come across as inflexible.
Insertion and deletion issues: When you insert or delete an element from an array, all the elements have to be shifted to accommodate the change. This can lead to poor efficiency as it is time-consuming.
Issues with Memory Allocation: Large arrays can cause your program to crash, particularly if you are using a system with limited memory. Allocating a large array can cause the system to run out of memory, causing the program to crash.
Wasted space: This could particularly be a concern for systems with limited memory. In case an array is not fully populated, it leads to wasted space in the memory allocated for the array.

Real-Time Applications of Arrays
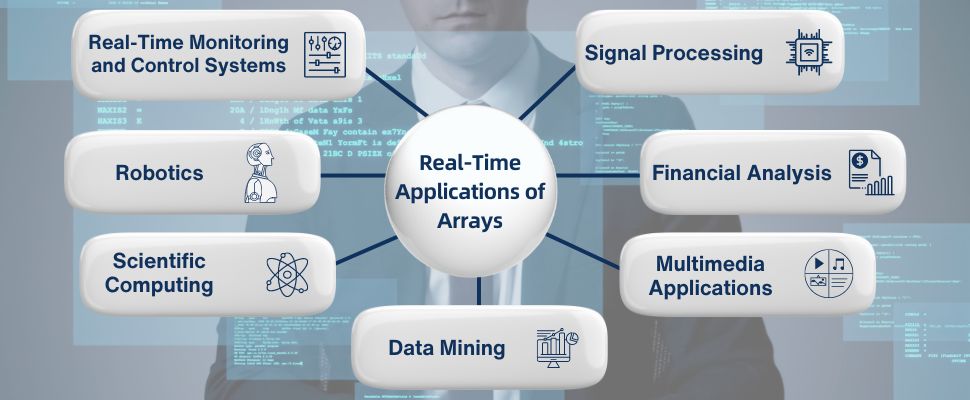
Signal Processing
Arrays can be used to represent samples collected over time. This is useful in signal processing and can be utilized in image processing, radar systems, and speech recognition.
Financial Analysis
Financial applications store financial data like historical stock prices and more. Arrays can help store such data and facilitate data access and analysis in real-time trading systems.
Multimedia Applications
Arrays can be used in multimedia applications to process video and audio. For example, the RGB values of an image can be stored using an array.
Data Mining
Arrays are used to represent large datasets in data mining applications. This makes it possible to access and process data efficiently, which has great benefits for real-time applications.
Scientific Computing
Measurements from experiments and simulations can be efficiently shown as numerical data in scientific computing with the help of arrays. It makes efficient data processing and visualization possible, which is crucial in real-time scientific analysis and experimentation.
Robotics
Arrays are used in motion planning, object recognition and other applications to show the position and orientation of objects in 3D space.
Real-Time Monitoring and Control Systems
Applications for industrial automation and aerospace systems need real-time processing and decision-making. With the help of arrays, you can build real-time monitoring and control systems that store sensor data and control signals.
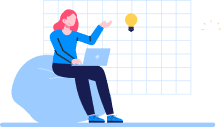
Don't miss out on your chance to work with the best!
Apply for top job opportunities today!

Conclusion
Mastering data structures is one of the humble beginnings in your journey as an expert programmer and developer. Arrays are among the oldest data structures used in programs that help build robust software solutions and versatile applications. Whether one-dimensional, two-dimensional or multi-dimensional, arrays provide a systematic and contiguous means to organize and access data. Although they have some disadvantages, the benefits of using arrays make them a vital part of representing and processing data efficiently. As a result, the real-time applications of arrays showcase their advantages across diverse domains. A deep understanding of arrays is crucial to making informed decisions when tackling complex problems in computer programming.
FAQs
There are two types of arrays in C++: single-dimensional and multi-dimensional.
In programming and coding, arrays are used to store multiple pieces of data of the same type together.
No. Arrays are common in object-oriented programming languages like C, Java, and Python.
Plain C arrays are immutable because the structure itself cannot change once it has been created. However, objects and arrays are mutable in JavaScript.
Take control of your career and land your dream job!
Sign up and start applying to the best opportunities!
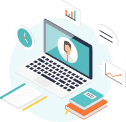