Table of Contents
Toggle
Introduction
In software programming, data refers to the value given to a variable and what functions (relational, logical, mathematical) can be applied through it. Python data types categorize various data items stored inside a variable. In this blog, let’s understand what Python data types are and look at the different data types in Python.
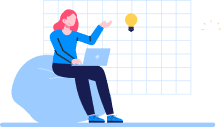
Don't miss out on your chance to work with the best
apply for top global job opportunities today!

What Is a Data Type in Python?
Data types are an important concept in computer programming. They categorize the various knowledge terms and tell us what operation a certain data can perform. In Python, every variable holds a value. A Python data type tells us what value each variable holds. Python is a dynamically typed language, so the interpreter automatically assigns data types to variables based on the values they hold. Each data type in Python has its characteristics and methods, which allow versatile and expressive programming.
Must read: Top 14 Trending Python Applications in 2024

Data Types in Python
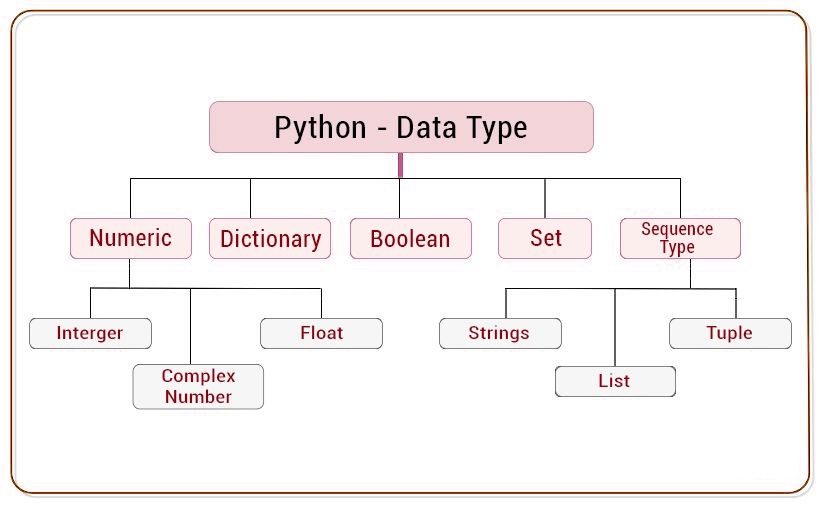
In Python programming, everything is an object. Data types are classes, and variables are instances or objects of these classes. Here are the standard data types in Python:
- Numeric
- Sequence
- Boolean
- Set
- Dictionary
- Binary
We need to define the values of various data types and check their data types. To do this, the type() function is used.
x = “Hello World”
x = 50
x = 60.5
x = 3j
x = [“python”, “data”, “types”]
x = (“python”, “data”, “types”)
x = range(10)
x = {“name”: “Gautam”, “age”: 24}
x = {“python”, “data”, “types”}
x = frozenset({“python”, “data”, “types”})
x = True
x = b”Python”
x = bytearray(4)
x = memoryview(bytes(6))
x = None
The variable “x” has been assigned to different values of various data types in Python. It covers string, integer, float, complex, list, tuple, range, dictionary, set, frozenset, boolean, bytes, bytearray, memoryview, and the special value ‘None’. As you can see, each assignment replaces the previous value, where ‘x’ takes on the data type and value of the most recent assignment.

Numeric Data Types in Python
Data that has numeric value is represented by numeric data types in Python. The different numeric values that can be assigned are:
- Integer: It is defined as Python int and is represented by the int class. Integers can have positive or negative whole numbers. Python can have long integer values.
- Floating number: It is defined as Python float and is represented by the float class. It is a real number that represents a floating point specified by a decimal point. Optionally, you can append the character e or E followed by a positive or negative integer to specify scientific notation.
- Complex number: It is defined as Python complex. A complex class represents a complex number. It is specified as (real part) + (imaginary part)j. For example – 4+5j
Let’s look at an example of how the type() function code determines the data type of variables in Python. It prints the data types of three variables: a (integer), b (float), and c (complex). The output is the respective data types for each variable.

Numeric Data Types in Python
a = 5
print(“Type of a: “, type(a))
b = 5.0
print(“\nType of b: “, type(b))
c = 2 + 4j
print(“\nType of c: “, type(c))
Output
Type of a: <class ‘int’>
Type of b: <class ‘float’>
Type of c: <class ‘complex’>

Sequence Data Type in Python
It is the ordered collection of similar or different data types. With sequences, you can store multiple values efficiently.
Sequence Types in Python
- Python String
- Python List
- Python Tuple

Python String
Arrays of bytes representing Unicode characters are called strings in Python. A collection of one or more characters put in a single quote, double-quote, or triple-quote is referred to as string. There is no character data type in Python, but a character is a string of length one and is represented by str class.
Let’s look at an example of a Python code with various string creation methods. We can see single quotes, double quotes, and triple quotes to create strings with different content and includes a multiline string. The code also shows printing the strings and checking their data types.
String1 = ‘Welcome to the Tech World’
print(“String with the use of Single Quotes: “)
print(String1)
String1 = “I’m a Techie”
print(“\nString with the use of Double Quotes: “)
print(String1)
print(type(String1))
String1 = ”’I’m a Techie and I live in a world of “Nerds””’
print(“\nString with the use of Triple Quotes: “)
print(String1)
print(type(String1))
String1 = ”’Techie
For
Life”’
print(“\nCreating a multiline String: “)
print(String1)
Output
String with the use of Single Quotes:
Welcome to the Tech World
String with the use of Double Quotes:
I’m a Techie
<class ‘str’>
String with the use of Triple Quotes:
I’m a Techie and I live in a world of “Nerds”
<class ‘str’>
Creating a multiline String:
Techie
For
Life

Accessing String Elements
Python uses indexing as a method to access individual characters of a String. Negative address references can access characters from the back of the String with the help of negative indexing. For instance, -1 refers to the last character, -2 refers to the second last character, and so on.
Example:
The following Python code demonstrates how to work with a string named ‘String1′. It initializes the string with “JobsForYou” and prints it. It then showcases how to access the first character (“J”) using an index of 0 and the last character (“u”) using a negative index of -1.
String1 = “JobsForYou”
print(“Initial String: “)
print(String1)
print(“\nFirst character of String is: “)
print(String1[0])
print(“\nLast character of String is: “)
print(String1[-1])
Output
Initial String:
JobsForYou
First character of String is:
J
Last character of String is:
u

Python List
Python lists are a collection of ordered data, just like arrays, declared in other languages. Lists are flexible because the items in a list don’t have to be of the same type.
Lists in Python are created by placing the sequence inside the square brackets[].
Here is an example of how a Python code creates and manipulates a list.
List = []
print(“Initial blank List: “)
print(List)
List = [‘JobsForYou’]
print(“\nList with the use of String: “)
print(List)
List = [“Jobs”, “For”, “You”]
print(“\nList containing multiple values: “)
print(List[0])
print(List[2])
List = [[‘Jobs’, ‘For’], [‘You’]]
print(“\nMulti-Dimensional List: “)
print(List)
Output
It starts with an empty list and prints it. Then a list containing a single string element is created and printed. Following this, a list with multiple string elements is created, and selected elements are printed from the list. It also prints and creates a multi-dimensional list (a list of lists). The code showcases various ways to work with lists, including single and multi-dimensional lists.
Initial blank List:
[]
List with the use of String:
[‘JobsForYou’]
List containing multiple values:
Jobs
You
Multi-Dimensional List:
[[‘Jobs’, ‘For’], [‘You’]]

Python Access List Items
The index number helps in accessing the list items, and this can be done using the index operator [ ] to access an item in a list. Negative sequence indexes represent positions from the end of the array in Python. One can just mention List[-3] instead of computing the offset as in List[len(List)-3].
Negative indexing means beginning from the end, -1 refers to the last item, -2 refers to the second-last item, etc.
List = [“Jobs”, “For”, “You”]
print(“Accessing element from the list”)
print(List[0])
print(List[2])
print(“Accessing element using negative indexing”)
print(List[-1])
print(List[-3])
Output
Accessing element from the list
Jobs
You
Accessing element using negative indexing
Jobs
You

Python Tuple
Tuples are similar to lists, except that tuples are immutable. A tuple is represented by a tuple class.
To create tuples in Python, one must use commas to separate the sequence of values placed. This can be with or without the use of parentheses for grouping the data sequence. Tuples can have unlimited elements of any datatype.
It is possible to create tuples with a single element, but it must have a trailing ‘comma’ to make it a tuple.
Tuple1 = ()
print(“Initial empty Tuple: “)
print(Tuple1)
Tuple1 = (‘Jobs’, ‘For’)
print(“\nTuple with the use of String: “)
print(Tuple1)
list1 = [1, 2, 4, 5, 6]
print(“\nTuple using List: “)
print(tuple(list1))
Tuple1 = tuple(‘Jobs’)
print(“\nTuple with the use of function: “)
print(Tuple1)
Tuple1 = (0, 1, 2, 3)
Tuple2 = (‘python’, ‘job’)
Tuple3 = (Tuple1, Tuple2)
print(“\nTuple with nested tuples: “)
print(Tuple3)
Output
Initial empty Tuple:
()
Tuple with the use of String:
(‘Jobs’, ‘For’)
Tuple using List:
(1, 2, 4, 5, 6)
Tuple with the use of function:
(‘G’, ‘e’, ‘e’, ‘k’, ‘s’)
Tuple with nested tuples:
((0, 1, 2, 3), (‘python’, ‘jobs’))

Accessing Tuple Items
- Refer to the index number.
- Use the index operator [ ] to access an item in a tuple.
- Remember that the index must be an integer, and one must use nested indexing to access nested tuples.
The code creates a tuple named ‘tuple1′ with five elements: 1, 2, 3, 4, and 5. Then, it prints the first, last, and third-last elements of the tuple using indexing.
tuple1 = tuple([1, 2, 3, 4, 5])
print(“First element of tuple”)
print(tuple1[0])
print(“\nLast element of tuple”)
print(tuple1[-1])
print(“\nThird last element of tuple”)
print(tuple1[-3])
Output
First element of tuple
1
Last element of tuple
5
Third last element of tuple
3

Boolean Data Type in Python
It is a data type that represents the truth values, True or False. Boolean objects equal to True are truthy (true), and those equal to False are falsy (false). The class bool is used to denote non-Boolean objects as true or false.
Let’s look at an example of a Boolean data type.
print(type(True))
print(type(False))
print(type(true))
Output
The first two lines will print the type of the Boolean values True and False, which is <class ‘bool’>.
<class ‘bool’>
<class ‘bool’>
There will be an error in the third line as true is not a valid keyword in Python. Python distinguishes between uppercase and lowercase letters as it is a case-sensitive language. The first letter of true must be capitalized to make it a Boolean value.
Traceback (most recent call last):
File “/home/7e8862763fb66153d70824099d4f5fb7.py”, line 8, in
print(type(true))
NameError: name ‘true’ is not defined

Set Data Type in Python
A Set is an unordered collection of data types that is iterable and mutable with no duplicate elements. The order of elements in a set may have various elements but is undefined.
The built-in set() function with an iterable object or a sequence helps in creating sets in Python. The sequence has to be placed inside curly braces, separated by a ‘comma.’ The type of elements in a set can be different, or mixed-up data type values can also be passed to the set.
Here is an example of how different types of values can be used to create sets.
set1 = set()
print(“Initial blank Set: “)
print(set1)
set1 = set(“JobsForYou)
print(“\nSet with the use of String: “)
print(set1)
set1 = set([“Jobs”, “For”, “You”])
print(“\nSet with the use of List: “)
print(set1)
set1 = set([1, 2, ‘Jobs’, 4, ‘For’, 6, ‘You’])
print(“\nSet with the use of Mixed Values”)
print(set1)
Output
Initial blank Set:
set()
Set with the use of String:
{‘F’, ‘o’, ‘J’, ‘u’, ‘r’, ‘b’, ‘s’}
Set with the use of List:
{‘Jobs’, ‘For’}
Set with the use of Mixed Values
{1, 2, 4, 6, ‘Jobs’, ‘For’}

Accessing Set Items
Sets are unordered, which means the items have no index and cannot be accessed by referring to an index. However, one can use a for loop or use the in the keyword to check if a specified value is present in a set to loop through the set items. Here is an example of a code that creates a set named set1 using the values “Jobs”, “For” and “You”.
set1 = set([“Jobs”, “For”, “You”])
print(“\nInitial set”)
print(set1)
print(“\nElements of set: “)
for i in set1:
print(i, end=” “)
print(“Geeks” in set1)
Output
Initial set:
{‘Jobs’, ‘For’}
Elements of set:
Jobs For
True

Dictionary Data Type in Python
In Python, a dictionary data type is an unordered collection of data values that store data values like a map. Other data types hold only a single value as an element, but a Dictionary holds a key known as a value pair. Key-values make the dictionary more optimized. To separate each key-value pair in a Dictionary, one must use a : and a , to separate each key.
To create a dictionary in Python, the sequence of elements is placed within curly {} braces, separated by ‘comma.’ Keys in a dictionary data type have to be immutable and cannot be repeated. However, values in a dictionary can be of any datatype and can be duplicated. The built-in function dict() in Python can also be used to create a dictionary. To create an empty dictionary, place it in curly braces{}. One must remember that dictionary keys are case-sensitive, which means that different cases of Key with the same name will be treated distinctly.
Here is an example that shows how a code creates and prints a variety of dictionaries.
Dict = {}
print(“Empty Dictionary: “)
print(Dict)
Dict = {1: ‘Jobs’, 2: ‘For’, 3: ‘You’}
print(“\nDictionary with the use of Integer Keys: “)
print(Dict)
Dict = {‘Name’: ‘Jobs’, 1: [1, 2, 3, 4]}
print(“\nDictionary with the use of Mixed Keys: “)
print(Dict)
Dict = dict({1: ‘Jobs’, 2: ‘For’, 3: ‘You’})
print(“\nDictionary with the use of dict(): “)
print(Dict)
Dict = dict([(1, ‘Jobs’), (2, ‘For’)])
print(“\nDictionary with each item as a pair: “)
print(Dict)
Output
Empty Dictionary:
{}
Dictionary with the use of Integer Keys:
{1: ‘Jobs’, 2: ‘For’, 3: ‘You’}
Dictionary with the use of Mixed Keys:
{1: [1, 2, 3, 4], ‘Name’: ‘Jobs’}
Dictionary with the use of dict():
{1: ‘Jobs’, 2: ‘For’, 3: ‘You’}
Dictionary with each item as a pair:
{1: ‘Jobs’, 2: ‘For’}

Accessing Key-value in Dictionary
One must refer to the key name to access the items of a dictionary. Key value can be used inside square brackets. Another way of accessing the element from a dictionary is to use the get() method.
Here is an example that shows how to access elements in a dictionary.
Dict = {1: ‘Jobs’, ‘name’: ‘For’, 3: ‘For’}
print(“Accessing a element using key:”)
print(Dict[‘name’])
print(“Accessing a element using get:”)
print(Dict.get(3))
Output
Accessing a element using key:
For
Accessing a element using get:
Jobs

Conclusion
Data types in Python are ways of classifying knowledge items. In this article, we have covered the six built-in data types in Python. A good understanding of basic concepts, such as data types, is one of the easiest ways to acquire proficiency in Python. One must remember that no data type is better than another. One must ensure that it is not used for a task that it is not designed for. If you are a Python developer looking for top job opportunities then sign up with Olibr now!!
Take control of your career and land your dream job
sign up with us now and start applying for the best opportunities!
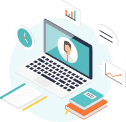
FAQs
In Python, data types help the computer system understand how to interpret the value of a piece of data.
Although type hints are not needed in Python, they can be used to improve code clarity and maintainability.
Python is an object-oriented, interpreted, high-level programming language that has dynamic semantics.
Python is used for a variety of tasks, such as developing websites and software, automating tasks, analyzing data, building programs for IoT-enabled apps, and also in game development.