Table of Contents
Toggle
Introduction
Cross-platform development skills are in high demand both for iOS and Android. Companies today seek to hire React Native developers who possess advanced skills. As the demand for skilled React Native developers continues to grow, mastering advanced React Native concepts is crucial. Our blogs on basic and intermediate React Native interview questions have already covered top-tier interview questions for beginners and experienced professionals. In this blog, you will find answers to complex React Native questions to help you explore the depths of React Native expertise.
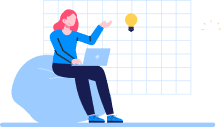
Don't miss out on your chance to work with the best
Apply for top global job opportunities today!

Top React Native Advanced Interview Questions
1. What causes performance issues in React Native?
Performance issues in Native React are caused when too many components pass through the threads unnecessarily. For example:
- JavaScript Thread: Heavy computations or blocking operations on the main JavaScript thread can slow down the UI.
- Excessive Re-renders: Frequent re-renders due to state changes can impact performance.
- Inefficient Components: Poorly optimized components or excessive use of forceUpdate can lead to bottlenecks.
- Large Images: Loading large images can cause memory and performance issues.
2. How to optimize applications in React Native?
Here are some ways of optimizing a React Native app:
- Use FlatList or SectionList: For efficient rendering of lists.
- Memoization: Use React.memo or useMemo to prevent unnecessary re-renders.
- Code Splitting: Split your code into smaller chunks using dynamic imports.
- Performance Profiling: Use tools like React DevTools or Flipper to identify bottlenecks.
3. How can you handle the dynamic linking of libraries in a React Native project?
To handle dynamic linking of libraries in a React Native project:
- Use react-native link to link native modules automatically.
- For custom linking, modify the native project files (e.g., AndroidManifest.xml, Info.plist).
4. What is Memory Leak issue in React Native?
Memory leak issues in React Native occur when an application unintentionally retains memory that it no longer needs, leading to gradual memory consumption over time. This can happen due to unclosed resources, circular references, or uncollected objects. Identifying and resolving memory leaks is crucial to maintain app performance and prevent crashes.
5. How can you detect memory leak issues in React Native?
Detecting and handling memory leaks in React Native apps is crucial for maintaining performance and stability. Some effective approaches to detect the issues are:
For iOS
- Go to XCode → Product → Profile (⌘ + i)
It will show you all the templates and you can choose the leaks.
For Android
- Run the React Native app normally (react-native run-android)
Run Android Studio
On the menu:
click Tools → Android → Enable ADB Integration
Click Tools → Android → Android Device Monitor
When Android Device Monitor shows up, click Monitor → Preferences
You can also use Perf Monitor (Performance Monitor) to detect memory leaks in Android:
Import PerfMonitor from 'react-native/Libraries/Performance/RCTRenderingPerf';
PerfMonitor.toggle();
PerfMonitor.start();
setTimeout(() => {
PerfMonitor.stop();
}, 20000);
}, 5000);
Must Read: REACT NATIVE BASIC INTERVIEW QUESTIONS
6. Write an example code to resolve a memory leak issue in React Native.
import React, { useEffect } from 'react';
import { DeviceEventEmitter } from 'react-native';
const ExampleComponent = () => {
useEffect(() => {
DeviceEventEmitter.addListener('exampleEvent', handleExampleEvent);
return () => {
// Missing clean-up function
};
}, []);
const handleExampleEvent = (eventData) => {
// Handle the event
};
return (
// Render component
);
};
7. How to store sensitive data in React?
React Native does not have built-in tools to store sensitive data. Here are ways to handle sensitive data storage in iOS and Android:
iOS – Keychain Services
- Keychain Services is an essential iOS feature that helps store sensitive securely.
- It allows you to store small pieces of user data (such as tokens, passwords, or encryption keys) in an encrypted format.
- Keychain data is the most secure way to store sensitive information on iOS, as it is protected by the device’s secure enclave.
Android – Secure Shared Preferences
- On Android, you can use Secure Shared Preferences to store sensitive data.
- It provides a secure storage mechanism for key-value pairs.
- Android Keystore is used under the hood to encrypt and protect the data
Android – Keystore
When working with React Native, consider using the following libraries for secure storage:
- expo-secure-store: Wraps Keychain on iOS and uses EncryptedSharedPreferences on Android.
- react-native-encrypted-storage: Provides a unified API for secure storage across platforms.
- react-native-keychain: Integrates with native keychain services (iOS Keychain, Android Keystore)
8. What is SSL Pinning in React Native?
SSL pinning is a crucial security technique used in React Native (and other mobile platforms) to enhance the security of network communication. SSL pinning involves embedding a public key, hash, or certificate directly into your app’s code.
There are several ways to implement SSL pinning in React Native:
Using Libraries
- react-native-ssl-pinning: A library that supports SSL pinning using OkHttp on Android and AFNetworking on iOS1.
- react-native-ssl-public-key-pinning: Another library that supports SSL public key pinning using the base64-encoded SHA-256 hash of a certificate’s Subject Public Key Info23.
Manual Implementation
- Extract the public key or hash from the server’s certificate.
- Compare it with the certificate received during network requests.
- Reject requests if they don’t match.
9. Discuss the use of ErrorUtils in error handling within a React Native app.
ErrorUtils is a global utility that intercepts unhandled JavaScript exceptions and provides a way to handle them gracefully. It’s especially useful for capturing and logging errors during development and production.
Follow these steps to import ErrorUtils in React Native.
import { ErrorUtils } from 'react-native';
// Set a global error handler
ErrorUtils.setGlobalHandler((error, isFatal) => {
// Handle the error (e.g., log it, show a custom error screen)
console.error('Unhandled error:', error);
if (isFatal) {
// Perform any necessary cleanup or crash the app
// (e.g., close resources, notify the user)
// Note: In production, you might want to restart the app.
}
});
// Example: Trigger an unhandled error
const divideByZero = () => {
const result = 1 / 0; // This will throw an exception
console.log('Result:', result);
};
divideByZero();
10. What is setNativeProps and how is it used?
setNativeProps is used in React Native to manipulate components directly. It allows you to directly modify properties of a native component (e.g., a view or text) without triggering a full re-render of the entire component tree. It is like setting properties directly on a DOM node in web development. However, Composite components (not backed by a native view) cannot use setNativeProps. If you try to call it on a composite component, you’ll encounter an error. It should only be used on native components (e.g., View, Text, etc.)
Example usage: Suppose you have a TouchableOpacity that changes opacity on press. The setNativeProps updates the opacity of the child view without triggering a re-render.
import { View, Text, TouchableOpacity } from 'react-native';
const MyComponent = () => {
const viewRef = useRef<View>();
const setOpacityTo = useCallback((value) => {
viewRef.current.setNativeProps({ opacity: value });
}, []);
return (
<TouchableOpacity onPress={() => setOpacityTo(0.5)}>
<View ref={viewRef}>
<Text>Press me!</Text>
</View>
</TouchableOpacity>
);
};
11. How is InteractionManager used for smooth animation?
InteractionManager ensures that your code runs when the app is idle, improving performance and responsiveness. InteractionManager.runAfterInteractions() is used to schedule a function to run after any ongoing interactions (such as animations or gestures) have been completed.
Example:
InteractionManager.runAfterInteractions(() => {
// ...long-running synchronous task...
});
You can replace the comment with the actual long-running synchronous task you want to execute.
12. How to use transitionConfig to create a custom transition animation between screens using 'react-navigation?
To create a custom transition animation between screens using createStackNavigator in React Navigation, you can use the transitionConfig option. This allows you to define your own animation logic.
Here is an example of a custom transition:
const customTransitionConfig = () => ({
transitionSpec: {
duration: 500, // Set your desired duration
easing: Easing.out(Easing.poly(4)), // Set your easing function
timing: Animated.timing,
},
screenInterpolator: (sceneProps) => {
const { layout, position, scene } = sceneProps;
const { index } = scene;
const width = layout.initWidth;
const translateX = position.interpolate({
inputRange: [index - 1, index, index + 1],
outputRange: [width, 0, -width],
});
return { transform: [{ translateX }] };
},
});
13. How to set up stack navigator with the desired screens?
To use stack navigator, import it from @react-navigation/stack:
import { createStackNavigator } from '@react-navigation/stack';
const Stack = createStackNavigator();
function MyStack() {
return (
<Stack.Navigator>
<Stack.Screen name="Home" component={Home} />
<Stack.Screen name="Notifications" component={Notifications} />
<Stack.Screen name="Profile" component={Profile} />
<Stack.Screen name="Settings" component={Settings} />
</Stack.Navigator>
);
}
14. What role does Babel play in React Native development?
Babel is a JavaScript compiler that transforms modern JavaScript (ES6+) code into a compatible format for older environments (such as browsers or Node.js). It allows developers to use the latest language features while ensuring compatibility with older platforms. React Native uses Babel to convert React syntax and newer ES5+ syntax into code that can run in a JavaScript environment that doesn’t natively support those features. Babel parses your code and generates equivalent code that works across different platforms.
Must Read: React Native Intermediate Questions
15. How would you implement a custom loading spinner in React Native?
You can create a component that uses the ActivityIndicator component from the React Native library. This will help you implement a custom loading spinner in React Native. You can also style it according to your design specifications. Moreover, it is also possible to create a reusable wrapper component that encapsulates the ActivityIndicator along with additional UI elements like text or icons. This allows you to easily reuse the loading spinner throughout your app with consistent styling.
16. How do you handle version updates and migrations in a React Native project?
Version updates and migrations in React Native project can be handled systematically.
- Maintain a version control system to track changes.
- Document your codebase and dependencies.
- Keep the project’s dependencies updated.
You can use tools like react-native-git-upgrade to update the React Native version while managing compatibility issues. Most importantly, it is important to follow platform-specific guidelines for handling version updates, especially for native modules. With thorough testing and continuous integration, you can ensure a smooth transition during updates.
17. What is the importance of code signing in React Native app deployment?
Code signing is a crucial step in preparing your React Native apps for deployment on mobile devices. It is a security practice used in app development to ensure the integrity and authenticity of the app. Code signing creates a unique digital signature for your iOS or Android app using cryptographic signatures. It prevents unauthorized modifications to the app’s code and is an essential step for in-app security and app store approval processes.
18. Discuss the role of PureComponent in React Native and when to use it.
In React and React Native, PureComponent automatically implements a shallow comparison of the props and state. It is a base class that optimizes rendering performance. When the data is unchanged, PureComponent helps prevent unnecessary re-renders. PureComponent is used when a component’s output does not need any additional complex logic or side effects. The component is determined by its props and state and can lead to performance improvements in components that render frequently.
19. Explain the purpose of AccessibilityRole and AccessibilityState in React Native.
AccessibilityRole and AccessibilityState can be used to make apps more inclusive and usable for users with disabilities. They are props used to improve the accessibility of React Native components. AccessibilityRole defines the role of a component in the app’s user interface. AccessibilityState is used to define additional accessibility-related properties like checked, disabled, or selected.
20. Discuss the benefits of using TypeScript with React Native.
There are many advantages of using TypeScript with React Native:
Type Safety and Static Typing
TypeScript adds type definitions to JavaScript, which helps catch errors at compile time. Type annotations enhance code quality and prevent runtime issues.
Improved Developer Experience
TypeScript provides better tooling support, including autocompletion and type inference. Accurate type information makes refactoring easy.
Enhanced Code Maintainability
Type annotations describe expected values as they allow self-documentation of codes.
In addition, TypeScript simplifies debugging by highlighting type mismatches.
Early Error Detection
Because of compile-time checks, errors can be detected before production. This also ensures a more robust application.
Integration with Existing JavaScript Code
It is possible for TypeScript and JavaScript modules to coexist. Also, developers can transition smoothly by adding TypeScript files alongside existing JavaScript files.
21. How can you implement a parallax effect in a React Native app?
Implementing a parallax effect in a React Native app can add depth and interactivity to your UI.
Here is how you can implement a parallax effect in a React Native app:
- Install react-native-reanimated for animations.
- Import necessary modules like interpolate, useAnimatedScrollHandler, useSharedValue, useAnimatedStyle, ScrollView, View, and Text.
- Create a shared value to track scroll position (scrollY).
- Define a scroll handler with useAnimatedScrollHandler to update scrollY as the user scrolls.
- Animate elements using useAnimatedStyle and interpolate, mapping scroll position to animation properties (e.g., translateY for vertical parallax).
- Use Animated.Extrapolate.CLAMP to constrain animation values within a defined range.
22. Explain the role of requestAnimationFrame in managing animations.
requestAnimationFrame is an API available in both web browsers and React Native. Its primary purpose is to optimize animations by synchronizing them with the device’s display refresh cycle. Here’s how it works:
- When you use requestAnimationFrame, your animation callback is executed just before the browser or app repaints the screen.
- By aligning animations with the display refresh rate, it significantly reduces the risk of jank (jerky movements) and stuttering.
- In React Native, the Animated library often leverages requestAnimationFrame internally to manage animations effectively.
In summary, requestAnimationFrame ensures smoother and more efficient animations across different platforms.
23. How can you ensure data consistency and integrity when syncing large datasets in a React Native app?
- Store data locally using SQLite or AsyncStorage.
- Implement differential sync.
- Handle conflicts with resolution strategies.
- Use batched updates and an offline queue for network failures.
24. How is react-native-webview used to embed web content in a React Native app?
React Native WebView is a component that allows you to embed web content, such as websites or web applications, within your React Native app. It is like a connection between native code and web code, which enables you to seamlessly display web-based features. It can be used to show external websites, web-based authentication, and integrate third-party web services. However, web views can introduce potential vulnerabilities and impact app performance. So, it is important to use it carefully.
25. How do you handle orientation changes in a React Native app?
There are a few ways of handling orientation changes in a React Native app. You can use the built-in Dimensions API or the react-native-orientation library. The Dimensions API gives information about the screen dimensions. You can update UI by subscribing to orientation change events. In addition, you can also use responsive design techniques, such as Flexbox or percentage-based dimensions. This makes sure that your UI elements adapt correctly to different orientations and screen sizes.
26. Explain the purpose of the ImageBackground component and its benefits.
You can use the ImageBackground component to display an image as the background of a container when using React Native. It simplifies creating visually appealing UIs with background images. To control aspects like image source, image style, and content alignment, ImageBackground offers props. ImageBackground also streamlines the design process and contributes to a more polished app interface. It can be used to add images behind other UI elements while maintaining proper sizing and positioning.
27. What is ActivityIndicator in a React Native app?
ActivityIndicator is used to display a spinning indicator to signify a loading or processing state. It is a built-in component in React Native that acts as a visual hint to inform users that something is happening in the background. The color, size, and visibility of the ActivityIndicator can be controlled based on the loading status of your app. ActivityIndicator improves user experience as it gives feedback and prevents user confusion during asynchronous operations.
28. How to handle global app state without Redux or Context API?
You can handle global app state without Redux or Context API. To do this, you have to create a module that exports a function to manipulate the state and listeners to subscribe to state changes. It can function as a simple custom global state manager. You can also use a state management library like MobX or Zustand which provides more structured solutions for managing a global state.
29. How is LayoutDebugger used to identify layout issues in a React Native app?
LayoutDebugger is a part of the React Native package. It is a tool that can be used to identify layout problems in an app. When you enable LayoutDebugger, you can use it to overlay colored borders on components and highlight their boundaries and dimensions. It helps in taking care of incorrect sizing, unexpected spacing, and alignment problems. LayoutDebugger helps developers a lot during the development and debugging phases, as it allows them to fine-tune the UI layout for consistency and visual appeal.
30. How to use react-native-svg to render vector graphics in a React Native app?
React-native-svg in React Native is a library that allows the rendering of scalable vector graphics (SVG) in React Native applications. It has components that can be used to create SVG-based UI elements such as shapes, paths, and text. React-native-svg helps create visually rich and scalable designs, icons, and illustrations within an app while maintaining a small memory footprint.
31. How to integrate React Native with existing native code in an app?
You need to create a bridge between the JavaScript code of React Native and the native code (Java for Android, Objective-C/Swift for iOS). Native modules can be set up to expose native functionality to JavaScript. You can also use RCT_EXPORT_METHOD or annotations to define methods accessible from React Native. ReactRootView can be used to embed React Native components into native views for advanced integrations.
32. What is deep linking in a React Native application?
Deep linking in a React Native application allows users to navigate directly to specific screens or content within the app. It enables seamless redirection from external sources (such as URLs or other apps) to relevant parts of your React Native app. Intercepting and processing URLs that point to specific sections of your app form a part of deep linking in a React Native application.
The React-native-linking library is used to handle deep links. You should register URL schemes or universal links (for iOS) in the app’s configuration. The library triggers an event containing the URL when the app is launched through a deep link. The URL can then be parsed and navigated to the appropriate screen or perform the desired action based on the link’s data.
33. Explain the purpose of FlatList and SectionList for efficient data rendering.
FlatList and SectionList are components in React Native that efficiently render large lists of data. FlatList renders single-column layout while SectionLista adds sections and headers. Both components use the virtualization technique where only the visible items are rendered, which improves performance and memory usage. Lazy loading, pull-to-refresh, and customizable rendering are a few features offered by these components through props like renderItem and renderSectionHeader.
34. What role does Geolocation play in getting the user's current location in a React Native app?
In a React Native app, Geolocation is an API that plays a crucial role in determining the user’s current location. It helps build location-based apps, mapping features, and provides location-specific content. You can request the user’s permission to access location services and retrieve latitude and longitude coordinates by using the navigator.geolocation object.
35. How can you implement a sliding menu (drawer) navigation in a React Native app?
In React Native, the createDrawerNavigator function from the React Navigation library sets up a navigation drawer on the side of the screen. You can open and close the drawer via gestures, which allows users to access different screens or sections of your app.
36. Explain the concept of 'Imperative vs Declarative' animations in React Native.
Imperative animations involve explicitly specifying each step of the animation process. Here, you provide instructions to the system on how to perform the animation. Example:
// Imperative animation using Animated API
Animated.timing(myValue, {
toValue: 1,
duration: 500,
useNativeDriver: true,
}).start();
Imperative animations offer fine-grained control over animation details. They are useful for complex, custom animations. However, they are wordy and prone to error and require managing the animation state manually.
Declarative animations focus on describing what the end result should look like. Here, you define the desired outcome, and the system handles the animation details. Example:
// Declarative animation using Animated API
<Animated.View style={{ opacity: myValue }}>
{/* Content */}
</Animated.View>
Declarative animations are concise and expressive. However, there are limited customizations for intricate animations.
37. Discuss the concept of Bridgeless architecture in React Native (Hermes engine).
The Bridgeless architecture is an alternative runtime for React Native apps. It was introduced in the Hermes JavaScript engine and reduces the communication overhead between JavaScript and native code by optimizing the execution of JavaScript code on the native side. Bridgeless improves app startup performance and reduces memory consumption.
38. How do you implement smooth transitions when navigating between screens using react-navigation?
You can use transition animations by the React Native library to implement smooth transitions between screens using react-navigation. You can define custom transition animations by configuring the following options
- transitionSpec
- screenInterpolator
- createStackNavigator
These options help you create visually pleasing transition effects by letting you control how the old screen fades out and the new screen fades in, slides, or scales.
39. How does VirtualizedList improve performance in long lists?
VirtualizedList is a component in React Native used by FlatList and SectionList. It optimizes rendering performance for long lists of data. Instead of rendering all items at once, VirtualizedList renders only the items visible within the viewport, recycling components as the user scrolls. This reduces memory consumption significantly and rendering time, which makes the UI more responsive and efficient. VirtualizedList helps with large datasets and ensures smooth scrolling and a better user experience.
40. How does NetInfo manage network connectivity in React Native?
NetInfo is a module in React Native that allows developers to monitor the network state, detect changes in connection status, and respond accordingly. It can be used to adapt the app’s behavior based on whether the device is online, offline, or transitioning between states. NetInfo helps create consistent user experience and robust apps that can efficiently handle different network conditions and provide.
41. How to implement GraphQL with a React Native app?
The Apollo Client library helps implement GraphQL in a React Native app. It has tools for making GraphQL queries, mutations, and subscriptions. It also manages data caching and state management. Apollo Client helps with data management and synchronization and enhances the app’s performance and responsiveness.
42. How does PanResponder handle touch gestures?
PanResponder is a built-in React Native module that helps with touch gestures and user interactions. It can be used to create complex touch-driven interactions like dragging, swiping, and zooming. PanResponder also helps you define callbacks for different touch events like onStartShouldSetPanResponder, onMoveShouldSetPanResponder, and onPanResponderMove.
43. How to use CodePush for over-the-air updates in React Native?
CodePush enables over-the-air updates for React Native apps without the need to download a new version from the app store. With it, updates can be directly pushed to users’ devices. CodePush also ensures quick bug fixes, feature enhancements, and other changes. It is best to use this tool for non-critical updates that can do without a new app store release.
44. How would you implement biometric authentication in a React Native app?
You can implement biometric authentication in a React Native app by using native modules or third-party libraries like react-native-biometrics or react-native-touch-id. These libraries have APIs that can be used to interact with the device’s biometric authentication features. Before granting access to sensitive parts of your app, the APIs prompt users for biometric authentication ensuring security and convenience.
45. What is the purpose of InteractionManager module in React Native?
InteractionManager can be used to manage interactions and prioritize tasks based on user interactions. It is a module in React Native with methods like runAfterInteractions and createInteractionHandle that allow the scheduling of tasks to be executed after the current interaction cycle is complete. This helps with less critical tasks such as data fetching or animations, until the user interactions and UI updates are finished.
46. Discuss the concept of React Native Fabric and its advantages.
Fabric is React Native’s new rendering system, designed as a conceptual evolution of the legacy render system. The advantages of React Native Fabric are:
Performance Boost: Fabric significantly improves performance by providing a more efficient UI layer. Synchronous rendering ensures smoother interactions and eliminates layout “jumps” when embedding React Native views in host components.
Code Quality and Consistency: Fabric promotes type safety through code generation, ensuring consistency across JavaScript and host platforms. Its shared C++ core simplifies cross-platform development, making it easier to maintain and extend React Native to new platforms.
Enhanced Interoperability: With synchronous and thread-safe layout calculations, Fabric seamlessly integrates with host platform frameworks. It also offers better overall performance and reliability as it reduces data serialization between JavaScript and the host platform.
47. How can you implement real-time synchronization in a React Native app?
You can use WebSocket, Firebase Realtime Database, or GraphQL subscriptions to implement real-time synchronization in a React Native. These tools allow instant updates when data changes on either side by allowing real-time communication between clients and servers. Real-time synchronization is useful for chat applications, live notifications, and collaborative editing. It is important to handle network disconnections and gracefully manage state changes to provide a seamless and reliable real-time experience.
48. Explain the purpose of BackHandler in handling Android back button events.
BackHandler is a module in React Native that provides methods to register event listeners for back button presses. It is used to handle the Android hardware back button. It also allows you to define custom behavior when the back button is pressed. This helps in managing navigation, modals, and dialog boxes. BackHandler ensures consistent and intuitive navigation in the React Native app, enhancing user experience and preventing unexpected app behavior.
49. What role do Error Boundaries play in React Native?
A class component becomes an error boundary if it defines either (or both) of the lifecycle methods static getDerivedStateFromError() or componentDidCatch(). Error Boundaries are components in React (and React Native) that catch and handle errors occurring within their child components. These components prevent crashing of the entire app. The Error Boundary displays a fallback UI when an error occurs. This makes the app more stable by isolating errors and allows users to continue using the app even when certain components encounter issues.
50. How do you handle dynamic data rendering using FlatList and SectionList?
When you provide FlatList and SectionList with a data array they render the items based on the data automatically. You can set the data prop and the renderItem or renderSectionHeader callback and ensure that the UI updates whenever the data changes. The components also support keyExtractor for efficient re-rendering and getItemLayout for better performance with large datasets.
51. How are DeviceEventEmitter and NativeEventEmitter used in event handling?
DeviceEventEmitter and NativeEventEmitter help in handling events between JavaScript and native code in React Native. DeviceEventEmitter is a part of the core ‘react-native’ package. It is used for emitting and listening to events from the native side in JavaScript. NativeEventEmitter is a wrapper around the native platform’s event emitter. It provides a more consistent API.
52. How would you implement a custom animated transition between screens?
- Use the react-navigation library’s TransitionPresets or createStackNavigator options.
- Use TransitionSpecs and screenInterpolator to define a custom animation configuration. Animated library can be used for more advanced animations.
- Create unique and visually appealing transitions by adjusting timing, opacity transforms, and other properties.
53. How do the props in TextInput work in React Native?
The TextInput component in React Native serves as a foundational input element for capturing text via a keyboard.
Configurable Props
Auto-Correction: Enables or disables auto-correction of text input.
Auto-Capitalization: Controls how text is capitalized (e.g., first letter of each word, all uppercase, etc.).
Placeholder Text: Displays a hint or example text when the input is empty.
Keyboard Types: Supports various keyboard layouts (numeric keypad, email, phone, etc.).
54. Discuss the role of useMemo and useCallback hooks in React Native.
You can utilize useMemo and useCallback to improve performance by memorizing values and functions. useMemo prevents expensive recalculations when dependencies remain unchanged while memorizing values. useCallback memorizes functions so that they are not recreated on every render. Both help in optimizing components that rely on computationally intensive operations.
55. How would you implement background tasks in a React Native app?
Use libraries like react-native-background-task or native modules like HeadlessJS to implement background tasks in a React Native. The libraries help developers run tasks in the background even when the app is not active. You can use platform-specific APIs like BackgroundFetch on iOS or JobScheduler on Android for periodic tasks.
56. Discuss the role of NavigationContainer and its significance.
To manage the navigation tree for an app, you can use NavigationContainer component in the react-navigation library. The component is a container for all the navigation components like StackNavigator, DrawerNavigator, and TabNavigator, to work seamlessly.

Conclusion
A good grasp of the advanced React Native concepts increases your chance of cracking interviews for React Native jobs. This blog explores interview questions specifically tailored for React Native developers with advanced skills. Whether you’re preparing for a job interview or simply aiming to deepen your expertise, these questions cover a wide spectrum of topics. For instance, you can improve your knowledge of performance optimization, React Native architecture, modules, gesture handling, and more. However, remember that these questions are primarily intended to push the boundaries of your knowledge and encourage a continuous learning process. As an advanced React Native developer, if you are looking for opportunities that match your skills, sign up with Olibr now!
Take control of your career and land your dream job
Sign up with us now and start applying for the best opportunities!
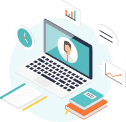
FAQs
React Native is an open-source UI software framework by Meta. It allows developers to create native apps and doesn’t compromise your users’ experiences.
React Native jobs offer good pay packages as cross-platform developers are in high demand. A React developer earns $117,770 per year in the United States, while a React Native developer earns an average annual salary of ₹ 5.4 lacs in India.
You should combine several complementary skills with React Native, including a good understanding of HTML, CSS, JavaScript, mobile development concepts, and networking and APIs.
Both Flutter and React Native are strong contenders for cross-platform mobile app development. Flutter offers a faster development process, better performance, and a consistent user interface. However, React Native has larger community support and a more versatile codebase.