Table of Contents
Toggle
Introduction
Cross-platform mobile applications are in high demand in today’s digital landscape. React Native is among the top frameworks and libraries used by developers to build these apps. if you are a developer looking forward to your first job interview, you have come to the right place! The basic interview questions covered in this blog give a clear picture of the basics of React Native. Whether you are learning React Native or getting ready to crack your first job interview, our simple explanations will help you grasp the basics of React Native easily. Read on to learn more!
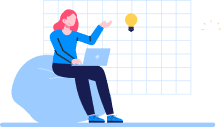
Don't miss out on your chance to work with the best
Apply for top global job opportunities today!

Top React Native Basic Interview Questions and Answers
1. What is React Native and how is it different from React?
React Native is a framework used by developers to build native mobile applications. React is a JavaScript library used to build responsive user interfaces for web applications.
In terms of syntax, both React and React Native use JSX (JavaScript XML), but React uses HTML tags like <div>, <h1>, and <p>, while React Native uses <view> and <text>.
2. How to install React Native?
There are two ways to set up a React Native development environment.
Using Expo (Recommended for Beginners):
There are two prerequisites for this:
Node.js: Ensure you have a recent version of Node.js installed.
Phone or Emulator: You’ll need a phone or emulator to run your app.
- Create a new React Native project
npx create-expo-app AwesomeProject
cd AwesomeProject
npx expo start
2. Install the Expo Go app on your iOS or Android phone and connect it to the same wireless network as your computer.
3. On Android, use the Expo Go app to scan the QR code from your terminal to open your project. On iOS, use the built-in QR code scanner in the default iOS Camera app.
4. Modify your app by editing App.js. The application will automatically reload when you save changes.
Using React Native CLI (For Experienced Developers)
For this you need Xcode (for iOS) or Android Studio (for Android)
1. Create a new React Native project
npx react-native init AwesomeProject
cd AwesomeProject
npx react-native start
2. Install the Expo Go app on your phone (iOS or Android) and connect it to the same network as your computer.
3. To run on an Android Virtual Device, use: npm run android
4. To run on the iOS Simulator (macOS only), use: npm run ios
3. Explain the architecture of a React Native app.
React Native apps have a component-based architecture. A component-based has reusable UI components and data management that use state and props. Moreover, it uses libraries like React Navigation for navigation and Redux for global state management.
4. What is Flexbox and what are some of its most used properties?
Flexbox is a layout model that allows elements to align and distribute space within a container. Below are the properties of Flexbox.
flexDirection: It is used to specify whether elements will be aligned vertically or horizontally. It uses the values column and row.
justifyContent: it is used to determine how elements should be distributed inside the container. The values used are center, flex-start, flex-end, space-around, and space-between.
alignItems: It is used to determine how elements should be distributed inside the container along the secondary axis. The values used are center, flex-start, flex-end, and stretched.
5. What is JSX in React Native?
JavaScript XML (JSX) is a syntax extension in React Native. It allows embedding HTML-like elements within JavaScript code. JSX allows developers to write components using a familiar HTML structure, which simplifies UI rendering. By getting transpiled into JavaScript, JSX enables React Native components to be expressed in a readable and intuitive format.
6. How to create a component in React Native?
You can create a component in React Native by defining a JavaScript function or class that returns JSX elements. Here is an example:
import React from 'react'; import { View, Text } from 'react-native'; const MyComponent = 0) => {
return ( <View> <Text>Hello, React Native!</Text> <View>): }; export default MyComponent;
7. What are React Native vector icons used for?
React Native vector icons are customizable icons that can integrate seamlessly into a project. They are used for embellishing buttons, logos, and navigation or tab bars.
8. What are the advantages of using React Native?
The advantages of using React Native are:
Large Community: React Native is a community driven open-source framework. Developers can find solutions by getting online assistance from fellow developers.
Code Reusability: Developers can reuse written code for both iOS and Android. This helps maintain the code and makes it easier to debug complex applications.
Live and Hot Reloading: Live reloading makes it possible to reload the entire app when a file changes. Hot reloading only refreshes the files that were changed without losing the state of the app.
Additional Third-Party Plugins: React Native allows third-party plugins which can help speed up the development process.
9. Explain Threads and their use in React Native.
A thread is a sequence of instructions that the CPU executes. It is a single path of execution within a program. In React Native, threads control the single sequential flow of control within a program. There are 3 threads used by React Native.
MAIN/UI Thread: This is the main application thread that runs an Android/iOS app. The main thread has access to the UI of the application and can change it.
Shadow Thread: It is a layout created using the React library in React Native. It handles UI layout and measurements by calculating the dimensions. The shadow thread collaborates with the main thread to ensure the smooth rendering of UI during layout calculations.
JavaScript Thread: It executes the main JavaScript code. This thread performs DOM manipulation asynchronously and handles user events, scroll events, etc.
10. Why is the render method important in React Native components?
The render method in React Native returns the JSX representation of the component’s UI. Based on the current state and props of the screen, it defines what the component should render on the screen. React Native automatically updates the UI when the state or props change, re-invoking the ‘render’ method to reflect those changes visually.
11. Explain how to style a React Native component.
You can use the StyleSheet component to style React Native components. The StyleSheet component offers an interface like CSS for defining styles. You can use properties like ‘flex’, ‘margin’, ‘padding’, ‘color’, and more.
import { StyleSheet} from 'react-native'; const styles = StyleSheet.create({ container: {flex: 1,
justifyContent: 'center', alignitems: 'center', backgroundColor: 'lightblue', }, text: { fontSize: 18, fontWeight: 'bold', color: 'white', }, });
12. How are default props used in React Native?
Here’s how default props are used in React Native to import React, {Component} from ‘react’
import {View, Text} from 'react-native';
class DefaultPropComponent extends Component {
render() {
return (
<View>
<Text>
{this.props.name}
</Text>
</View>
)
}
}
Demo.defaultProps = {
name: 'BOB'
}
export default DefaultPropComponent;
13. What is 'state' in React Native and how is it different from 'props'?
State in React Native is used to control the components. It stores variable data. A state is mutable, that is, it can change value at any time. Here is how we can create Text component with state data:
import React, {Component} from 'react';
import { Text, View } from 'react-native';
export default class App extends Component {
state = {
myState: 'State of Text Component'
}
updateState = () => this.setState({myState: 'The state is updated'})
render() {
return (
<View>
<Text onPress={this.updateState}> {this.state.myState} </Text>
</View>
); } }
14. How is user input handled in React Native?
In React Native, user input is handled with a component called TextInput. This component allows users to input text via the keyboard. It provides props like onChangeText, onSubmitEditing, and onFocus to handle user interactions.
Example code
import React, { useState } from 'react';
import { TextInput, View, Button } from 'react-native';
const MyForm = () => {
const [text, setText] = useState('');
const handleInputChange = (value) => {
// Handle changes to the input value
setText(value);
};
const handleFormSubmit = () => {
// Handle form submission (e.g., send data to a server)
console.log('User input:', text);
};
return (
<View>
<TextInput
placeholder="Enter something..."
value={text}
onChangeText={handleInputChange}
onSubmitEditing={handleFormSubmit}
/>
<Button title="Submit" onPress={handleFormSubmit} />
</View>
);
};
export default MyForm;
15. Explain the React Native component lifecycle.
The React Native component lifecycle consists of the following phases.
Mounting Phase
- Creation: When a component is first created, it enters the mounting phase.
- Constructor: The component’s constructor is called, where you can initialize state and bind methods.
- Render: The render( ) method is invoked, returning the initial UI representation.
- ComponentDidMount: After rendering, componentDidMount( ) is called. It’s a good place for side effects (e.g., data fetching) and setting up subscriptions.
Updating Phase
- Props or State Change: When a component’s props or state changes, it re-enters the updating phase.
- ShouldComponentUpdate: React checks if the component should update by calling shouldComponentUpdate( ). You can optimize performance here.
- Render: If the component should update, it re-renders using the updated props and state.
- ComponentDidUpdate: After rendering, componentDidUpdate( ) is called. Useful for handling side effects after an update.
Unmounting Phase
- Removal: When a component is removed from the DOM, it enters the unmounting phase.
- ComponentWillUnmount: Before unmounting, componentWillUnmount( ) is called. Clean up resources (e.g., cancel subscriptions).
Error Handling
- If an error occurs during rendering or in lifecycle methods, the error handling phase is triggered.
- Use componentDidCatch( ) to handle errors gracefully.
16. How to debug a React Native application?
Debugging in React Native is different for iOS and Android.
Dev Menu
- Access the Dev Menu by shaking your device or using keyboard shortcuts:
- iOS Simulator: Cmd ⌘ + D (or Device > Shake)
- Android emulators: Cmd ⌘ + M (macOS) or Ctrl + M (Windows and Linux)
From the Dev Menu, you can:
- Open the Debugger to understand how your JavaScript code runs.
- Use React DevTools to inspect the element tree, props, and state.
- View errors and warnings in LogBox (disabled in production builds).
React Native Debugger
- Install React Native Debugger, a standalone debugging tool.
- Run your app and start debug mode (shake your device or use keyboard shortcuts).
- React Native Debugger provides an experience like Android Studio or Xcode, allowing you to write code and debug within the same app
Console Errors and Warnings
- Errors and warnings appear as on-screen notifications.
- Tap a notification to see an expanded view and paginate through other logs.
- Use LogBox.ignoreLogs( ) to selectively disable notifications for specific logs.
Read More: 10 BEST FRAMEWORKS FOR BACKEND DEVELOPMENT
17. What is Redux and what components of Redux are used in a React Native app?
Redux is a powerful state management library in React Native. It helps to manage the state of applications efficiently. Some key components of Redux commonly used in React Native applications are:
Store
- The store is the central place where the entire application state is stored.
- It holds the data for your app, and each component can access this state.
- To create a store, use the createStore function from Redux.
Actions
- Actions describe what happened in your app. They are plain JavaScript objects.
- An action typically has a type (a string) and additional data (payload).
- Example: const incrementCounter = { type: ‘INCREMENT’, payload: 1 };
Reducers
- Reducers specify how the application’s state changes in response to actions.
- They are pure functions that take the current state and an action and return a new state.
Example
const counterReducer = (state = 0, action) => {
switch (action.type) {
case 'INCREMENT':
return state + action.payload;
default:
return state;
}
};
Dispatch
- The dispatch function sends an action to the store.
- It triggers the reducer to update the state based on the action.
- Example: store.dispatch(incrementCounter);
Middleware
- Middleware sits between dispatching an action and the reducer.
- It allows you to add custom logic, handle asynchronous actions, or modify actions.
- Popular middleware includes redux-thunk (for async actions) and redux-logger (for logging).
Selectors
- Selectors are functions that extract specific data from the state.
- They help components access relevant parts of the state.
- Example: const selectCounter = (state) => state.counter;
18. What are Timers in React Native Application?
In React Native, timers play a crucial role in managing asynchronous tasks and scheduling events. The different types of timers available in React Native are:
Timeouts: setTimeout allows you to execute a function after a specified delay (in milliseconds). Example:
setTimeout(() => {
console.log('Delayed execution after 1000ms');
}, 1000);
Intervals: setInterval repeatedly calls a function at a specified interval.
const intervalId = setInterval(() => {
console.log('Repeated execution every 2000ms');
}, 2000);
// To stop the interval:
// clearInterval(intervalId);
Immediate Execution: setImmediate executes a function at the end of the current JavaScript execution block. It’s useful for tasks that need to run immediately after other synchronous code.
Example:
setImmediate(() => {
console.log('Executed immediately after current block');
});
Animation Frames: requestAnimationFrame schedules a function to run before the next browser frame repaint. It is useful for smooth animations and avoiding layout thrashing. Example:
const animate = () => {
// Animation logic here
requestAnimationFrame(animate);
};
animate();
19. What is Props Drilling and how can we avoid it?
Props drilling happens when props are passed from a top-level component to multiple nested components. Although some intermediate components do not use props, it can lead to cluttered code and reduced maintainability.
Steps to avoid props drilling:
- React Context API
- Composition
- Render props
- HOC
- Redux or MobX
20. How to make AJAX network calls in React Native using Fetch API?
The Fetch API allows you to send HTTP requests with various configurations (such as headers, methods, and payloads).
Example:
fetch('https://api.example.com/data')
.then((response) => response.json())
.then((data) => {
console.log('Received data:', data);
})
.catch((error) => {
console.error('Error fetching data:', error);
});
21. How to make AJAX network calls in React Native using AJAX Libraries?
- There are alternative libraries like SuperAgent and Wretch that you can use for AJAX requests.
- These libraries offer additional features and customization options.
- Example using SuperAgent (install SuperAgent first):
import request from 'superagent';
request.get('https://api.example.com/data')
.end((error, response) => {
if (error) {
console.error('Error fetching data:', error);
} else {
console.log('Received data:', response.body);
}
});
22. How to integrate React Native into an existing Android mobile application?
You can integrate Redux in React Native by creating a store to hold the global state. You also have to use components like ‘Provider’ to make the state available to the app. Actions, reducers, and middleware are used to update and manage the state.
23. How to handle navigation between screens in React Native?
You can use libraries like React Navigation to manage navigation between screens in React Native. First, define a navigator component and configure the screens you want to navigate to. Then use navigation methods like ‘navigate’, ‘push’, ‘goBack‘, etc., to move between screens.
24. What are 'keys' in React Native and why are they important in lists?
Keys in React Native are special attributes used to identify elements in a list. Keys help React Native optimize re-rendering when the list changes. This improves performance and prevents rendering glitches.
25. Describe the purpose of 'AsyncStorage' in React Native.
AsyncStorage in React Native is an API in React Native for asynchronous, unencrypted, and persistent storage of small amounts of data. You can use it to store settings, preferences, or authentication tokens locally on the user’s device.
26. How do you optimize performance in a React Native application?
You can use PureComponent for performance optimization in React Native. You can also optimize render methods, avoid unnecessary re-renders, and implement code splitting. Additionally, profiling tools like React DevTools can help identify performance bottlenecks.
27. Explain the concept of 'HOC' (higher-order component) in React Native.
A HOC is a design pattern in React Native. It can be used to wrap a component with additional functionality. HOC is used for cross-cutting concerns like authentication, data fetching, and logging, making code more modular and reusable.
28. How can you integrate third-party libraries in a React Native app?
You can use package managers like npm or yarn to integrate third-party libraries. The library can be installed and imported into your code. You can also use its components or APIs. You can also use the react-native link command to link native modules.
29. What is the role of 'navigator' in React Navigation?
Navigator is a core component in React Navigation that manages the navigation flow between screens in a React Native app. It defines the app’s navigation structure, handles transitions, and maintains the navigation stack.
30. What are Touchable components in React Native and how do they work?
Touchable components in React Native offer touch interactions for UI elements. TouchableOpacity and TouchableHighlight are examples of touchable components. These components respond to touch events like onPress and onLongPress and provide visual feedback, which makes apps feel more interactive.
31. How do you handle form validation in React Native?
State and event handling are used for form validation in React Native. Components like TextInput are used to capture user input. Then, you validate the input based on your criteria and display error messages accordingly.
32. How do you handle platform-specific code in React Native?
The Platform module in React Native is used to handle platform-specific code. It is used to render components conditionally or apply styles based on the iOS/Android platform. You can also create separate platform-specific files using naming conventions like ‘MyComponent.ios.js’ and ‘MyComponent.android.js’).

Conclusion
The React Native basic interview questions in this blog explore the key fundamentals of React Native that every developer should be familiar with. The topics and concepts covered lay the groundwork for building robust mobile applications. However, it is important to understand there is no template when appearing for interviews. Apart from understanding the concepts that test your React Native knowledge, you must also practice coding for real-world scenarios. If you are a React Native developer looking for opportunities that match your talent, sign up with Olibr now to learn more.
Take control of your career and land your dream job
Sign up with us now and start applying for the best opportunities!
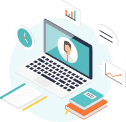
FAQs
- Good Command of HTML, CSS & JavaScript, and Basics of React
- Experience With Platforms (Windows, iOS)
- Knowledge of Third-Party Dependencies and APIs
- Testing and Debugging skills
- Performance Optimization skills
A good understanding of JavaScript, including ES6 and ES7 features, and proficiency in React principles, such as JSX, components, state, and props, is crucial to becoming a React Native expert.
Yes. A React developer earns $117,770 per year in the United States, while a React Native developer earns an average annual salary of ₹ 5.4 lacs in India.
The growing demand for cross-platform mobile apps has led to an increase in the demand for developers who can use React Native and other related technologies. In fact, the number of developers specializing in React Native has increased, as it efficiently caters to both iOS and Android platforms.