Table of Contents
Toggle
Introduction
If you are looking for advanced level JavaScript interview questions to prepare for your next interview, we have curated the most frequently asked in interviews. After you have gone through the JavaScript tutorial and revised the basic JavaScript concepts, look at these advanced JavaScript questions and answers.

JavaScript Interview Questions for Experienced Developers
1. What is the ‘Strict’ mode in JavaScript and how can it be enabled?
Strict mode is a feature in JavaScript that was introduced in ECMAScript 5. When enabled, it enforces additional constraints on your code, making it follow stricter rules. This feature prevents certain actions from being taken and throws more exceptions. To enable strict mode, you need to add the following expression at the beginning of your script or function:
"use strict";
2. How to get the status of a CheckBox?
To set or return the checked status of a checkbox field, you can use the DOM Input Checkbox Property. It can also be used to reflect the HTML Checked attribute.
document.getElementById("GFG").checked;
The CheckBox returns True if it is checked.
3. How to explain closures in JavaScript and when to use it?
A closure is a feature in JavaScript where an inner function has access to the outer (enclosing) function’s variables. This includes the outer function’s variables, parameters, and even the variables from the global scope.
// Explanation of closure
function foo() {
let b = 1;
function inner() {
return b;
}
return inner;
}
let get_func_inner = foo();
console.log(get_func_inner());
console.log(get_func_inner());
console.log(get_func_inner());
Closures can be used in the following cases:
- Data Privacy: To create private variables.
- Function Factories: To generate functions dynamically.
- Event Handlers: To maintain state between function calls.
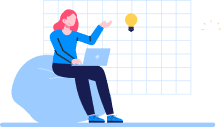
Don't miss out on your chance to work with the best
Apply for top global job opportunities today!
4. What is the difference between call() and apply() methods?
Both call() and apply() are used to invoke functions and set this inside the function.
- call(): Invokes the function with the specified this value and arguments provided individually.
- apply(): Invokes the function with the specified this value and arguments provided as an array.
Must Read: JAVASCRIPT INTERVIEW QUESTIONS FOR BEGINNERS
5. How to target a particular frame from a hyperlink in JavaScript?
You can use the target attribute in the hyperlink to target a particular frame from a hyperlink in JavaScript:
<a href="/OlibrBlogs.htm" target="newframe">New Page</a>
6. What are the errors shown in JavaScript?
Types of Errors in JavaScript
- Syntax Errors: Errors in the syntax of the code.
- Reference Errors: Accessing variables that don’t exist.
- Type Errors: Operations on incompatible types.
- Range Errors: Numbers out of range.
- Eval Errors: Errors related to the eval() function.
- URI Errors: Errors in URI handling functions.
7. What is the difference between JavaScript and Jscript?
- JavaScript: Developed by Netscape, it is the standard scripting language for web browsers. It is used to design client and server-side applications. It is completely independent of Java language.
- JScript: It is a scripting language by Microsoft. It is an implementation of the ECMAScript standard, used to design active online content for the World Wide Web.
8. What does the var myArray = [[]]; statement declare?
In JavaScript, var myArray = [[]]; is used to declare a two-dimensional array.
9. How many ways can an HTML element be accessed in JavaScript code?
- By ID: document.getElementById(‘id’)
- By Class Name: document.getElementsByClassName(‘className’)
- By Tag Name: document.getElementsByTagName(‘tagName’)
- By Name: document.getElementsByName(‘name’)
- Query Selector: document.querySelector(‘selector’)
- Query Selector All: document.querySelectorAll(‘selector’)
10. What is the difference between innerHTML & innerText?
innerHTML: Gets or sets the HTML content inside an element. It parses and renders HTML tags.
<div id="content">Hello <b>World</b></div>
<script>
let content = document.getElementById('content').innerHTML;
console.log(content); // Output: Hello <b>World</b>
</script>
innerText: Gets or sets the text content inside an element. It ignores HTML tags and returns only the text.
<div id="content">Hello <b>World</b></div>
<script>
let content = document.getElementById('content').innerText;
console.log(content); // Output: Hello World
</script>
11. What is an event bubbling in JavaScript?
Event bubbling is a type of event propagation in the HTML DOM API. When an event is triggered on an element, it first runs the handlers on that element, then on its parent, and then all the way up the DOM tree until it reaches the document object. This means that the event is first captured and handled by the innermost element and then propagated to the outer elements.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Event Bubbling Example</title>
</head>
<body>
<div id="parent" style="padding: 50px; border: 1px solid black;">
Parent
<div id="child" style="padding: 20px; border: 1px solid red;">
Child
</div>
</div>
<script>
document.getElementById('parent').addEventListener('click', function() {
alert('Parent clicked!');
});
document.getElementById('child').addEventListener('click', function(event) {
alert('Child clicked!');
// event.stopPropagation(); // Uncomment to stop bubbling
});
</script>
</body>
</html>
12. What will be the output of the following code?
let X = { Olibr: 1 };
let Output = (function () {
delete X.Olibr;
return X.Olibr;
})();
console.log(output);
The ‘delete’ will delete the property of the object. X is the object with the Olibr’s property and it is a self-invoking function that will delete the Olibr’s property from object X so the result will be undefined.
Read More: JAVASCRIPT INTERMEDIATE INTERVIEW QUESTIONS
13. How are JavaScript and ECMA Script related?
JavaScript is the main language that maintains some rules and regulations, which is ECMA Script. The rules also bring new features to the JavaScript language.
14. How to hide JavaScript code from old browsers that don’t support JavaScript?
To hide the JavaScript codes from the old browsers that don’t support JavaScript you can use:
<!-- before <script> tag and another //--> after </script> tag
By using the above code, all the old browsers will take ‘that’ as a long comment of HTML. New browsers that support JavaScript will take ‘that’ as an online comment.
15. What will be the output of the following code?
let output = (function(x) {
delete x;
return x;
})(0);
document.write(output);
The output will be 0. The delete operator is used to delete the operator of the object but the X is not the object here it is a local variable. The delete operator doesn’t affect local variables.
16. Answer if the following expressions result in true or false in JavaScript.
"0" == 0 // true or false ?
"" == 0 // true or false ?
"" == "0" // true or false ?
“0” == 0 results in true.
“” == 0 results in true.
“” == “0” results in false.
17. How to use any browser for debugging?
Most modern browsers have built-in developer tools for debugging JavaScript. These tools include:
Chrome: Developer Tools (F12 or Ctrl+Shift+I)
Firefox: Developer Tools (F12 or Ctrl+Shift+I)
Edge: Developer Tools (F12 or Ctrl+Shift+I)
Safari: Developer Tools (Develop > Show Web Inspector or Command+Option+I)
18. How to use an external JavaScript file in another JavaScript file?
This code can be used to use external JavaScript code in another JavaScript file.
let script = document.createElement('script');
script.src = "external javascript file";
document.head.appendChild(script)
19. What is the design prototype pattern in JavaScript?
The prototype pattern is a creational design pattern used to create new objects by cloning an existing object, known as the prototype. This pattern is particularly useful when the creation of new objects is resource intensive. Instead of creating new instances from scratch, new objects are created by copying the prototype.
20. How is the Temporal Dead Zone defined in JavaScript?
The Temporal Dead Zone refers to the time period between entering a scope where a variable is declared using let or const and the actual declaration of the variable. During this period, any reference to the variable will result in a ReferenceError.
21. What is the difference between the methods .map() and .forEach() in JavaScript?
- .map(): Creates a new array with the results of calling a provided function on every element in the array. It does not modify the original array.
let arr = [1, 2, 3];
let doubled = arr.map(num => num * 2);
console.log(doubled); // Output: [2, 4, 6]
- .forEach(): Executes a provided function once for each array element. It does not return a new array and does not modify the original array by itself.
let arr = [1, 2, 3];
arr.forEach((num, index, array) => {
array[index] = num * 2;
});
console.log(arr); // Output: [2, 4, 6]
22. How to hide JavaScript code from old browsers that don't support JavaScript?
After opening the <script> tag, we will use a one-line HTML style comment without any closing character ( <! – ). We will then write the JavaScript code that is to be hidden from older browsers. We will use the closing character with comment ( //–> ) before we close the script with the </script> tag.
Syntax:
<script>
<!--
// Your JavaScript code
// that is hidden from older browser
console.log("Hello Geeks");
//-->
</script>
23. Write a sample code for a JavaScript multiplication table.
function generateMultiplicationTable(number) {
for (let i = 1; i <= 10; i++) {
console.log(`${number} x ${i} = ${number * i}`);
}
}
generateMultiplicationTable(5);
24. Write a code to explain how unshift() works.
var name = [ "johnny" ];
name.unshift ( "charles" ) ; name. unshift "jose", "Jane" ) ; console. log (name) ;
Output:
[" jose,"," Jane,", " charles", " johnny "]
25. What are screen objects?
The ‘screen’ object contains information about the user’s screen. It can be used to determine properties like screen width, height, color depth, and more.
Properties:
screen.width: Returns the width of the screen.
screen.height: Returns the height of the screen.
screen.availWidth: Returns the width of the screen (excluding the taskbar).
screen.availHeight: Returns the height of the screen (excluding the taskbar).
screen.colorDepth: Returns the color depth of the screen.
26. Write the code to find the vowels
const findVowels = str => {
let count = 0
const vowels = ['a', 'e', 'i', 'o', 'u']
for(let char of str.toLowerCase()) {
if(vowels.includes(char)) {
count++
}
}
return count
}
27. How do you turn an Object into an Array [] in JavaScript?
let obj = { id: "1", name: "user22", age: "26", work: "programmer" };
//Method 1: Convert the keys to Array using - Object.keys()
console.log(Object.keys(obj));
// ["id", "name", "age", "work"]
// Method 2 Converts the Values to Array using - Object.values()
console.log(Object.values(obj));
// ["1", "user22r", "26", "programmer"]
// Method 3 Converts both keys and values using - Object.entries()
console.log(Object.entries(obj));
//[["id", "1"],["name", "user22"],["age", "26"],["work", “programmer"]]
28. What is the output of the following code?
const b = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10];
for (let i = 0; i < 10; i++) {
setTimeout(() => console.log(b[i]), 1000);
}
for (var i = 0; i < 10; i++) {
setTimeout(() => console.log(b[i]), 1000);
}
The output for the given code is:
1
2
3
4
5
6
7
8
9
10
undefined
undefined
undefined
undefined
undefined
undefined
undefined
undefined
undefined
undefined

Conclusion
As a JavaScript developer with advanced JS skills, you must remember that many JavaScript interviews are free in terms of structure. This also applies to JavaScript coding tests, irrespective of whether you appear for a live coding test or otherwise. The questions and answers in this blog should help you plan your JavaScript interview like an experienced professional would. Having said that, remember to stay updated with the current JavaScript trends and practice coding as much as possible. To find the best jobs to match your JavaScript developer skills, sign up with Olibr now!
Take control of your career and land your dream job
Sign up with us now and start applying for the best opportunities!
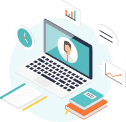
FAQs
Java and JavaScript are fundamentally different languages designed for different tasks. Java is a robust, general-purpose language commonly used in large-scale applications, whereas JavaScript is a versatile scripting language mainly used for web development.
Lexical scope in JavaScript means that the accessibility of variables is determined by the location of the variables within the nested function scopes in the source code.
As per Glassdoor estimates, the average salary for a JavaScript Developer is ₹ 6,35,000 per year in India. The average JavaScript developer salary in the USA is $117,000 per year or $56.25 per hour, as per talent.com.
Yes, a JavaScript developer can work remotely on building SaaS apps or work in frontend development to create user interfaces at scale. Developers who can implement JavaScript applications with an emphasis on optimization, API design, and architecture are in demand.