Table of Contents
Toggle
Introduction
JavaScript developers with intermediate programming skills are a great asset to a development team. Intermediate JavaScript skills are built upon the basics and delve deeper into the language. JavaScript intermediate interviews evaluate your deeper understanding of the JavaScript language beyond the basics. Let’s look at the important JavaScript intermediate interview questions to help you crack your interview.

JavaScript Intermediate Interview Questions
1. What are all the looping structures in JavaScript?
JavaScript provides several looping structures for iterating over data.
while loop: It is a control flow statement where code can be executed repeatedly based on a given Boolean condition. It is like a repeating if statement. It continues to execute as long as the condition is true.
for loop: It is a structure that provides a concise way of writing the loop structure. Unlike a while loop, the ‘for’ loop is a traditional loop that iterates a specified number of times. A ‘for’ statement consumes the initialization, condition, and increment/decrement in one line, providing a shorter, easy-to-debug structure of looping.
do while: A ‘do…while’ loop is like a ‘to…while’ loop but the only difference is that it checks the condition after executing the statements. This makes it an example of an Exit Control Loop.
2. How can the style/class of an element be changed?
The document.getElementByID method is used to change the style/class of an element in two ways:
document.getElementById("myText").style.fontSize = "16px;
document.getElementById("myText").className = "class";
3. Explain how to read and write a file using JavaScript?
Use the readFile() function for reading operation.
readFile( Path, Options, Callback)
Use the writeFile() function for writing operation.
writeFile( Path, Data, Callback)
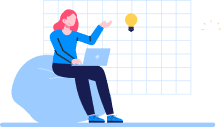
Don't miss out on your chance to work with the best
Apply for top global job opportunities today!
4. What is called Variable typing in JavaScript?
When you want to store a number and use that same variable to assign a string, use variable typing.
Olibr = 42;
Olibr = “JobsForYou";
5. How to convert the string of any base to an integer in JavaScript?
You can use the parseInt() function in JavaScript to convert the string to an integer. This function returns an integer of a specified base in the second argument of parseInt() function. The parseInt() function returns Nan (not a number) when the string doesn’t contain a number.
6. How to detect the operating system on the client machine?
You can use the navigator.userAgent property to detect the operating system.
let userAgent = navigator.userAgent;
if (userAgent.indexOf('Win') !== -1) {
console.log('Windows OS');
} else if (userAgent.indexOf('Mac') !== -1) {
console.log('Mac OS');
} else if (userAgent.indexOf('Linux') !== -1) {
console.log('Linux OS');
} else {
console.log('Unknown OS');
}
7. What are the types of Pop up boxes available in JavaScript?
Alert: Displays a message and an OK button.
alert('This is an alert box!');
Confirm: Displays a message, an OK button, and a Cancel button.
let result = confirm('Do you want to proceed?');
if (result) {
console.log('User pressed OK');
} else {
console.log('User pressed Cancel');
}
Prompt: Displays a message, a text input field, an OK button, and a Cancel button.
let userInput = prompt('Please enter your name:');
if (userInput !== null) {
console.log('User entered: ' + userInput);
}
Must Read: JavaScript Interview Questions for Beginners
8. What is the difference between an alert box and a confirmation box?
Alert Box: Only has an OK button and is used to display information to the user.
Confirmation Box: Has both OK and Cancel buttons and is used to get confirmation from the user.
9. What is the disadvantage of using innerHTML in JavaScript?
Security Risks: Using innerHTML can make your application vulnerable to Cross-Site Scripting (XSS) attacks if you are inserting untrusted data.
Performance: Setting innerHTML causes the browser to reparse and recreate the entire DOM subtree, which can be inefficient.
Loss of Event Listeners: When you use innerHTML, any event listeners attached to elements within that HTML will be lost.
10. What is the use of void(0)?
The void(0) expression evaluates to undefined and is often used in hyperlinks to prevent the default action of the link, while still allowing an event handler to be executed.
<a href="javascript:void(0)" onclick="alert('Link clicked!')">Click me</a>
This approach ensures that the link does not navigate to a new page or cause any other side effects.
11. How to create a cookie using JavaScript?
JavaScript cookies are small files stored on a user’s computer. JS cookies hold data specific to a particular client and website. They and can be accessed either by the web server or by the client’s computer. To create a cookie by using JavaScript, you must assign a string value to the document.cookie object.
document.cookie = "key1 = value1; key2 = value2; expires = date";
12. How to read a cookie using JavaScript?
To read a cookie, you can access document.cookie and parse it to find the value:
function getCookie(name) {
let nameEQ = name + "=";
let ca = document.cookie.split(';');
for(let i = 0; i < ca.length; i++) {
let c = ca[i];
while (c.charAt(0) === ' ') c = c.substring(1, c.length);
if (c.indexOf(nameEQ) === 0) return c.substring(nameEQ.length, c.length);
}
return null;
}
// Example usage
let username = getCookie('username'); // Retrieves the value of the 'username' cookie
console.log(username);
13. How to delete a cookie using JavaScript?
To delete a cookie, you set its expiration date to a past date:
function deleteCookie(name) {
document.cookie = name + '=; Max-Age=-99999999;';
}
// Example usage
deleteCookie('username'); // Deletes the 'username' cookie
14. What are escape characters and escape() functions?
Escape characters are needed to work with some special characters like single and double quotes, apostrophes, and ampersands. Special characters play an important role in JavaScript. To ignore or to print that special character, you can use the escape character backslash “\”. It will normally ignore and behave like a normal character.
// Need escape character
document.write("GeeksforGeeks: A Computer Science Portal "for Geeks" ")
document.write("GeeksforGeeks: A Computer Science Portal \"for Geeks\" ")
The escape() function takes a string as a parameter and encodes it so that it can be transmitted to any computer in any network that supports ASCII characters.
15. Does JavaScript have a concept-level scope?
JavaScript is not concept-level scope, the variables declared inside any function have scope inside the function. JavaScript uses function-level scope, meaning that variables declared within a function are only accessible within that function.
16. How to create generic objects in JavaScript?
To create a generic object in JavaScript use:
var I = new object();
17. Which keywords are used to handle exceptions?
Errors commonly occur when executing JavaScript code. These errors can occur due to wrong input by the programmer or faulty logic of the program. All errors can be solved by using these commands.
- The try statement lets you test a block of code to check for errors.
- The catch statement lets you handle the error if any are present.
- The throw statement lets you make your own errors.
18. What is the use of the blur function?
The blur function is used to remove focus from an element.
document.getElementById('myInput').blur();
19. What is the unshift method in JavaScript?
The unshift method adds one or more elements to the beginning of an array and returns the new length of the array.
let arr = [2, 3, 4];
arr.unshift(1); // Adds 1 to the beginning
console.log(arr); // [1, 2, 3, 4]
20. How to print screen in JavaScript?
By calling the window.print() method in the browser, we can print the content of the current window in JavaScript.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Print Screen Example</title>
</head>
<body>
<h1>Welcome to My Page</h1>
<p>This is an example of printing the screen using JavaScript.</p>
<button onclick="printPage()">Print this page</button>
<script>
function printPage() {
window.print();
}
</script>
</body>
</html>
HTML Structure
- A heading (<h1>), a paragraph (<p>), and a button (<button>).
- The button has an onclick attribute that calls the printPage function when clicked.
JavaScript Function
- The printPage function is defined in a <script> tag.
- Inside this function, the window.print() method is called to open the print dialog.
21. What is the main difference between Attributes and Property?
- Attributes provide more details on an element like id, type, value, etc.
- Property refers to the value assigned to the property such as type=”text” value=’Name’.
22. How is an HTML element accessible in JavaScript code?
An HTML element can be used in JavaScript code in the following ways:
getElementById(‘idname’): Gets an element by its ID name
getElementsByClass(‘classname’): Gets all the elements that have the given class name.
getElementsByTagName(‘tagname’): Gets all the elements with the given tag name.
querySelector(): It returns the first selected element using a CSS style selector.
23. How can a JavaScript code be involved in an HTML file?
JavaScript can be involved in an HTML file in several ways. These methods allow you to add interactivity, dynamic content, and other functionality to your web pages. Here are the main ways to include JavaScript in an HTML file:
Inline JavaScript: Inline JavaScript is placed directly within HTML elements using the onclick, onmouseover, or other event attributes.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Inline JavaScript Example</title>
</head>
<body>
<button onclick="alert('Hello, World!')">Click me</button>
</body>
</html>
Internal JavaScript: Internal JavaScript is placed within the <script> tags inside the <head> or <body> sections of the HTML file.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Internal JavaScript Example</title>
<script>
function showMessage() {
alert('Hello, World!');
}
</script>
</head>
<body>
<button onclick="showMessage()">Click me</button>
</body>
</html>
External JavaScript: External JavaScript is written in separate .js files and linked to the HTML document using the <script> tag with the src attribute. This method keeps the HTML and JavaScript code separate, making the code easier to maintain.
HTML file (index.html):
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>External JavaScript Example</title>
<script src="script.js" defer></script>
</head>
<body>
<button onclick="showMessage()">Click me</button>
</body>
</html>
JavaScript file (script.js):
function showMessage() {
alert('Hello, World!');
}
24. What is unshift method in JavaScript?
The unshift method in JavaScript is used to add one or more elements to the beginning of an array. This method modifies the original array and returns the new length of the array.
Syntax:
array.unshift(element1, element2, ..., elementN)
Example:
let arr = [2, 3, 4];
console.log(arr); // Output: [2, 3, 4]
let newLength = arr.unshift(0, 1);
console.log(arr); // Output: [0, 1, 2, 3, 4]
console.log(newLength); // Output: 5
25. What is the difference between Local storage and Session storage?
Local Storage: Data stored in local storage persists even after the browser is closed. Local storage data is accessible across different tabs/windows of the same browser. There is no expiration to local data; it remains until manually cleared by the user or the web app.
Session Storage: Data stored in session storage is cleared when the page session ends (i.e., when the browser is closed). Session storage data is specific to the current tab/window and is not shared across other tabs/windows. It is cleared automatically when the session ends (browser closure or tab/window closure).

Conclusion
As an intermediate JavaScript developer, recruiters test your deeper understanding of JavaScript and its applications. As a JavaScript developer, you need to demonstrate a good grasp of JS frameworks and libraries, error handling, performance optimization, and more, in your interviews. The questions listed here contain simple explanations to help you plan your interviews. However, remember, you also need to practice JavaScript coding questions and stay updated with what’s happening in the JavaScript community to ace your job interviews. Sign up with Olibr now for the best JavaScript jobs!
Take control of your career and land your dream job
Sign up with us now and start applying for the best opportunities!
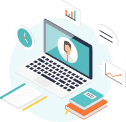
FAQs
The latest JavaScript update is ECMAScript 2023 (ES14), which came out in June 2023.
React, Angular, Vue.js, Ember.js, and Backbone are some of the most popular JavaScript frameworks.
To take user input in JavaScript, you can use the prompt() function. It displays a dialog box with a message, an input field, and “OK” and “Cancel” buttons. When the user clicks “OK,” the input value is returned as a string.
JavaScript is used in frontend and backend development, CLI development, mobile application development, desktop application development, game development, AI and Data Science, and even IoT and Robotics.