Table of Contents
Toggle
Introduction
Data structures help programming languages organize and store data efficiently. JavaScript (JS) is a dynamically typed scripting language where variables can receive different data types over time. In this blog, we will look at the different data types in JavaScript.

Why Are Data Types Important?
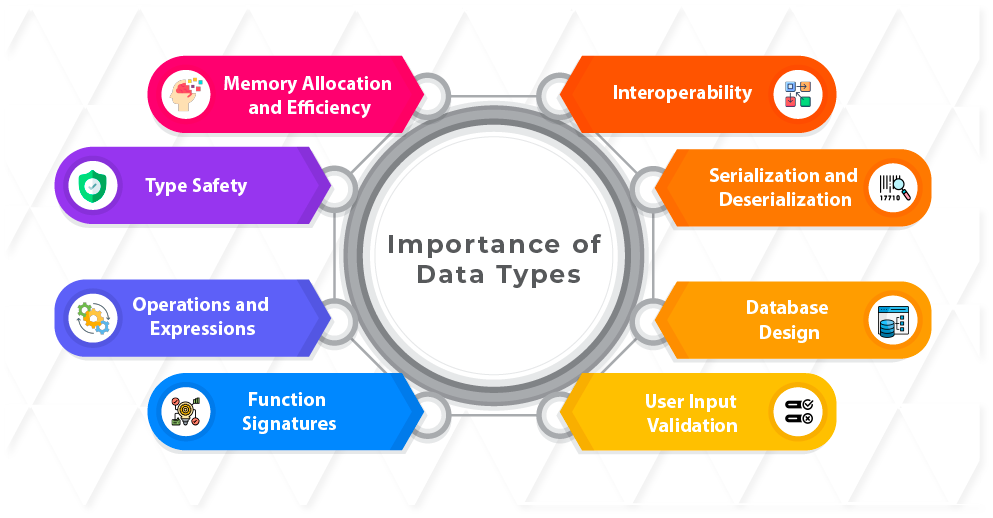
Programming languages have built-in data structures, and they play a crucial role in programming languages. Here are the top reasons why data structures are important:
Memory Allocation and Efficiency
For a programming language to offer optimal performance, it is important to use memory efficiently. The right data type ensures optimal memory allocation and reduces wastage. Data types determine how much memory is allocated to store a value. For example, an integer requires a fixed amount of memory, while a floating-point number needs more space.
Type Safety
Type safety improves code reliability and reduces runtime errors. For example, there are strict rules about data types with strongly typed languages. This prevents unintended operations like adding a string to an integer and catches errors at compile time.
Operations and Expressions
Data types support different operations in different programming languages. For instance, arithmetic operations work differently for integers and floating-point numbers. A good knowledge of data types allows the compiler or interpreter to handle expressions correctly.
Function Signatures
Functions have specific input and output data types known as signatures. These signatures lead to consistency and compatibility. Passing incorrect data types to a function can lead to unexpected behavior.
Interoperability
It is important that data types align with different components, like libraries, APIs, and databases, during integration. Consistent functioning between different parts of a system can happen when there is consistency in the data types.
Serialization and Deserialization
How you serialize and deserialize data depends on data types. For instance, JSON and XML are serialization formats that rely on data type information.
Database Design
Choosing the right data types ensures efficient storage and retrieval, as the structure of tables and columns is defined by data types.
User Input Validation
A good understanding of data types helps in validating user input. For instance, to prevent security vulnerabilities, you have to ensure that the input matches the expected type.
In summary, data types are essentially the foundation for writing correct, efficient, and reliable code. They guide how data is stored, manipulated, and processed within a program.
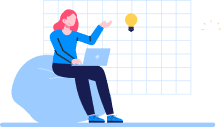
Don't miss out on your chance to work with the best
Apply for top global job opportunities today!

What Are the Data Types in JavaScript?
As per the latest ECMAScript standard, JavaScript data types can be divided into primitive and non-primitive data types. Let’s look at them in detail.
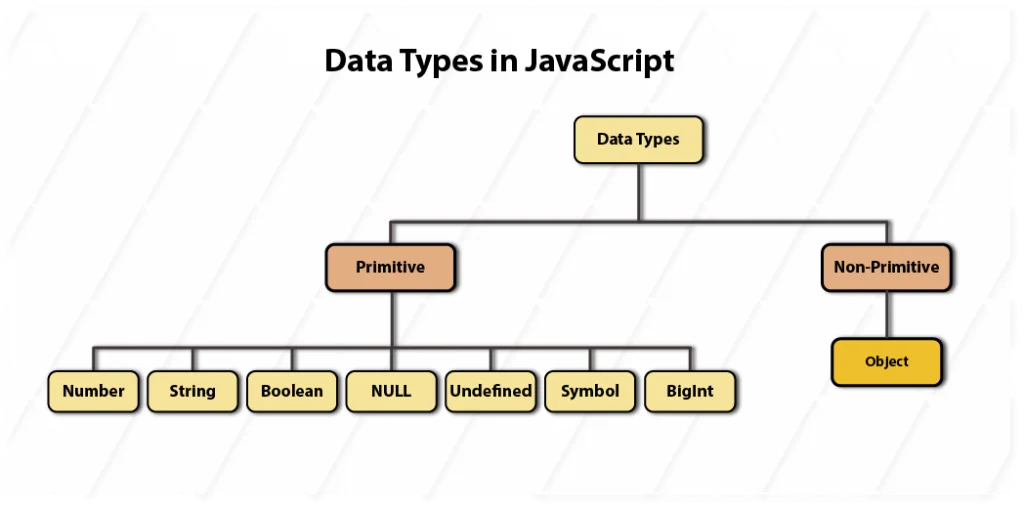

Primitive Data Types in JavaScript
There are seven primitive data types that are predefined and in-built into JavaScript.
Number
These data types represent numeric values (e.g., 42, 3.14). In JavaScript, you don’t need int, float, etc to declare different numeric values. Numbers are always stored in double-precision 64-bit binary format IEEE 754 in JS.
let num = 2; // Integer
let num2 = 1.3; // Floating point number
let num3 = Infinity; // Infinity
let num4 = 'something here too'/2; // NaN
String
A string data type is like a sentence. It consists of a list of characters that are essentially an array of characters. In other words, string data types a sequence of characters (e.g., “Hello, world!”). In JavaScript, Strings are values made up of text and can contain letters, numbers, symbols, punctuation, and even emojis. Strings in JavaScript are shown within a pair of either single quotation marks ” or double quotation marks “”.
let str = "Hello There";
let str2 = 'Single quotes works fine';
let phrase = `can embed ${str}`;
Boolean
It is a logical entity and can have two values: true or false. Boolean values are usually used for conditional operations, including ternary operators, if…else, and while.
let isCoding = true; // yes
let isOld = false; // no
Null
A null data type represents the absence of any value.
let age = null;
Undefined
An undefined data type represents an undefined variable or a variable that has not been assigned a value.
let x;
console.log(x); // undefined
Symbol
This is a data type introduced in ES6 and used for creating unique identifiers. Symbols help add unique property keys to an object that won’t collide with keys of any other code added to an object.
let symbol1 = Symbol("Olibr")
let symbol2 = Symbol("Olibr")
// Each time Symbol() method
// is used to create new global Symbol
console.log(symbol1 == symbol2); // False
BigInt
This is a built-in data object in JavaScript that represents whole numbers larger than 253-1.
let bigBin = BigInt("0b1010101001010101001111111111111111");
// 11430854655n
console.log(bigBin);
Also read: JAVASCRIPT INTERVIEW QUESTIONS FOR BEGINNERS

Non-Primitive Data Types in JavaScript
These are data types that are derived from primitive data types of JavaScript. Non-primitive data types are also known as derived data types or reference data types. There is one type of non-primitive data type:
Object
In computer science, an object is a value in memory that can be referenced by an identifier. Object data types are like the building blocks of modern JavaScript. They are the only mutable values.
Object constructor syntax:
let person = new Object();
Object literal syntax:
let person = {}; //

Conclusion
Data types are fundamental concepts in JavaScript and in programming in general. They determine how data is stored, accessed, and processed. Understanding these concepts as a JavaScript developer helps with writing efficient, maintainable, and error-free code. Sign up with Olibr now for more such insights from the tech world!
Take control of your career and land your dream job
Sign up with us now and start applying for the best opportunities!
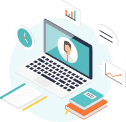
FAQs
To enable JavaScript in Google Chrome, follow these steps: Open Google Chrome on your computer. Click the three vertical dots icon at the top-right corner of the browser window. Select “Settings.” Scroll down and click “Advanced.” Under the “Privacy and Security” section, click “Site Settings.” Find “JavaScript” and ensure it is set to “Allowed.”
The most popular implementation of server-side JavaScript is Node.js. Node.js brings JavaScript to the server side, allowing developers to build scalable, high-performance, and event-driven applications.
JavaScript data structures are fundamental constructs that allow you to organize and store data efficiently within your programs. They include arrays, objects, linked lists, stacks, queues, sets, maps, and trees. These structures play a crucial role in solving various programming problems and optimizing algorithms.
The ‘typeof’ operator in JavaScript determines the data type of its operand. It returns the data type as a string. You can use it to identify the type of data you’re working with, whether it’s a variable, function, or object.