Table of Contents
Toggle
Introduction

What is a Data Structure

Classification of Data Structures
| Primitive Data Type | Non-Primitive Data Type | Abstract Data Type (ADT) |
Definition | Basic data types | Derived data types | Custom data types |
Storage Size | Fixed | Variable | Variable |
Memory Allocation | Stack | Heap | Heap |
Default Value | Assigned by default | null or undefined | N/A |
Examples | integer, float, character | Array, String, Object | List, Queue, Stack |
Mutability | Immutable | Mutable | Variable |
Operations | Simple operations | Complex operations | Custom operations |
Performance | Faster | Slower | Varies |
Direct Manipulation | Yes | Yes | No |
Also Read: Is Data Science an Overhyped Trend?

Top 10 Common Data Structures
Arrays
What is Array in data structures? An array is a fundamental data structure. It stores elements of the same type close to each other. They offer fast access to elements through their index. Arrays are ideal for situations where the collection size is known in advance.
Linked Lists
Your data is arranged in linear order and linked to one other in a linked list. It stops users from accessing random data. Users can only access data in the given sequence.
Stacks
In Stack also, items are arranged in a line. However, Stack works in a Last in, First Out (LIFO) order. So, it is also called a LIFO structure. LIFO structure allows you to access the last item in a structure first. The application of stack in data structure includes evaluating expressions consisting of operands and operators.
Queues
Queues again work like Stacks. However, Queues allow you to access the first element in a structure. It follows the First in First Out (FIFO) model.
Tuple
Tuple is a linear data structure. It stores the element in a particular sequence. Since it is indexed, users can access elements using an index or a slice operation.
Hash Tables
What is hashing in data structure? The hash table connects each item with a key and stores them. You can easily find a particular object from a set of similar objects.
Trees
Another critical basic data structure that a programmer should know is a Tree. In Trees, data is connected as in the Linked List. However, it is organized in ranking order – similar to a family tree.
Types of Trees include:
- Binary search tree (BST)Red-black tree
- B tree, treap
- Splay tree
- N-ary tree
- AVL tree
Note: Each of these Tree types is used for specific applications.
For instance, the Binary search tree stores data in grouped order.
BST Tree is mainly used in different types of search operations. Other Tree types are used in wireless networking and to create expression solvers.
Dictionary
A dictionary stores data as key-value pairs. Each value is connected to a unique key. We use keys to add, remove, or modify elements in the dictionary.
Sets
In Sets, the elements are not stored in a specific order. It does not allow duplicate elements. Users can perform set operations on two or more sets, such as Union, Intersection, and Difference.
Heaps
A heap is a dual tree data structure. In a heap, a parent node is compared to its child node and arranges values in the nodes accordingly.
There are two types of heaps:
Min heap: The parent’s key is equal to or less than the keys of its children.
Max heap: The parent’s key is greater than the keys of its children.
Heaps are generally used to find an array’s largest and smallest values. It is also used to create priority queues in algorithms.
Graphs
A graph is a non-linear data structure. The graph doesn’t store elements in a sequence. It represents objects connected in a network-like structure. The objects are linked through the edges. Graphs consist of vertices (nodes) and edges. Vertices contain information like Name, Age, etc. Edges are the lines connecting the nodes.

Importance of Data Structure
- Efficient Data Organization: Data structures provide efficient ways to store, organize, and manage data.
- Algorithm Design and Analysis: Data structures allow developers to choose the most appropriate structure for a specific problem.
- Memory Management: Data structures help allocate and deallocate memory dynamically. This reduces memory wastage. It also optimizes resource utilization. Developers can minimize memory expenses and improve program efficiency by choosing suitable data structures.
- Code Reusability: Well-defined data structures help you to reuse the codes. Once a data structure is implemented, it can be used in multiple programs and projects. This can save time and improve efficiency.
- Data Integrity and Consistency: Data structures provide procedures for enforcing data control, verifying inputs, etc. It makes sure that data is stored and retrieved accurately. Data structures contribute to reliable and error-free software systems by maintaining data consistency.
- Scalability and Flexibility: As data volumes increase, well-designed data structures can handle larger datasets without affecting performance. They allow for easy modifications, additions, and extensions to the data representation.
- Problem-Solving: Data structures help break down complex problems into smaller, manageable components. It helps analyze, design, and implement solutions quickly.
- Collaboration and Communication: Standardized data structures enable effective communication of ideas, algorithms, and data representations across teams. It makes it easier to share and understand code.
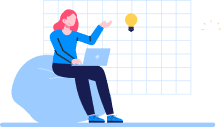
Don't miss out on your chance to work with the best!
Apply for top job opportunities today!

How can data structures be used in real-world applications?
Data structures play a crucial role in real-world applications by efficiently storing, organizing, and manipulating data. They are used in databases for fast data retrieval and management.
- Operating systems utilize data structures like queues and linked lists to manage processes and allocate memory.
- Networking applications rely on data structures such as graphs for efficient data transmission and routing.
- Compilers use data structures like symbol tables and abstract syntax trees to process program code.
- AI and machine learning algorithms utilize matrices and graphs to handle large datasets.
- Financial systems use data structures for order book management and real-time analytics.
- Gaming applications rely on grids, trees, and graphs for tasks like collision detection and pathfinding.
- Web development uses data structures for data storage and retrieval.

What resources are available to learn data structures?

www.hackerrank.com
- Beginner-friendly coding problem website with easy, medium, and hard questions.
- Categorized questions by difficulty and topics (Arrays, Strings, etc.).
- Language-specific tracks available for practice.

www.geeksforgeeks.org
- A comprehensive resource with tutorials and explanations for various tech topics.
- Dedicated practice platform with problem sorting by company and difficulty.
- Interview experiences section for preparation.

leetcode.com
- A popular platform for coding interviews with increasing difficulty levels (Easy to Hard).
- A discussion forum and interview experiences section are available.
- Regular coding challenges to participate in.

www.codechef.com
- A platform for DSA and competitive programming.
- Tutorials, forums, and practice sections for learning and improvement.
- A star rating system based on contest participation and performance.

codeforces.com
- Active community and discussion forums for competitive programming.
- Problem Set section with previous contest questions sorted by difficulty.
- Divisions (Div1 to Div4) for contests based on ratings, allowing progression.

Conclusion: Why should programmers learn Data Structures?

FAQs
Python data structures are collections of data elements organized and stored in memory, allowing for efficient manipulation and retrieval of data. Common data structures in Python include lists, tuples, dictionaries, sets, and arrays, each with its own characteristics and use cases.
Sorting in data structures refers to the process of rearranging a collection of elements into a specific order. The goal is to make the data easier to search, retrieve, or manipulate efficiently.
Traversing in data structures refers to the process of systematically visiting every element within a data structure. When we traverse a data structure, we access each element at least once. This operation is essential for various purposes, such as displaying elements or performing specific operations on them.
Take control of your career and land your dream job!
Sign up and start applying to the best opportunities!
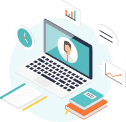