Table of Contents
Toggle
Introduction
If you are reading this blog, you have been successful as an intermediate Python programmer and are ready to take on new challenges in your career. Congratulations! You started from building your basics and strived to become a part of a thriving, global Python community. In this blog, we have compiled some of the most common interview questions for advanced Python candidates. These questions, along with our questions for basic and intermediate Python programmers, should give you a great headstart at different phases of your career. Read on to know more!

Top Python Advanced Interview Questions
1. What are the trending Python applications in 2024?
Python is used to build web apps and in software development. There are also a few modern technology fields where we can see an increase in Python applications in 2024:
- Data science, Analytics, and Visualization
- AI and Machine learning
- Blockchain and Smart Contracts
- Game development
- IoT & Embedded Systems
- DevOps & Automation
2. What does ‘self’ mean in Python?
An object or an instance of a class is called self. While it is optional in Java, it is included as the first parameter in Python explicitly. Self is used to distinguish between the methods and attributes of a class with local variables.
Syntax:
Class A:
def func(self):
print("Hi")
In the init method, the self variable refers to the newly created object. It refers to the object whose method was called in other methods.
3. How to access a module written in Python from C?
This method can be used to access the module written in Python from C.
Module == PyImport_ImportModule("<modulename>");
4. What are the different uses of the append() and extend() methods?
Both methods help add elements at the end of a list. However:
- To add a single element to the end of a list, use the append() method.
- To add elements from an iterable (e.g., another list) to the end of the list, use the extend().
5. How does Python Flask handle database requests?
To create or initiate the database in Flask, you need to install the sqlite3 command. There are three ways in which Flask handles database requests:
- The before_request() command is used to request database before only without passing arguments.
- The after_request() command is called after requesting the database and to send the response to the client.
- The teardown_request() command is used where the exception is raised for the responses not guaranteed. There is no access to modify the request.
6. What is docstring in Python?
A docstring in Python is a string literal appearing as the first statement in a module, function, class, or method definition. It documents the purpose, usage, and behavior of the module, function, or class. Docstrings can be identified by the Python interpreter by their triple quotes.
Syntax:
""" Using docstring as a comment. This code add two numbers """ x=7 y=9 z=x+y print(z)
7. How is multi-threading achieved in Python?
The multi-threading package in Python increases the time taken to execute a code. Instead, the GIL constructor ensures that only a single thread is executed at one time. Because of this process, a thread works with the acquired GIL and then passes the GIL onto the next thread. This is an instant process, which gives the illusion of parallel execution to the human observer. The threads, however, take turns utilizing the same CPU core as they execute sequentially.
8. What is functional programming? Does Python follow a functional programming style? If yes, list a few methods to implement functionally oriented programming in Python.
If the main source of logic in a program comes from functions, it is referred to as functioning coding style. Python follows functional programming. Using functional programming involves writing pure functions, which cause little or no changes outside the scope of the function. The changes, also known as side effects, are easy to test and debug.
Examples of functional programming in Python:
filter(): Filter lets us filter some values based on a
conditional logic.
list(filter(lambda x:x>6,range(9))) [7, 8]
map(): Map applies a function to every element in an iterable.
list(map(lambda x:x**2,range(5))) [0, 1, 4, 9, 16, 25]
reduce(): Reduce repeatedly reduces a sequence pair-wise until it reaches a single value.
from functools import reduce >>> reduce(lambda x,y:x-y,[1,2,3,4,5]) -13
9. Which one of the following is not the correct syntax for creating a set in Python?
- set([[1,2],[3,4],[4,5]])
- set([1,2,2,3,4,5])
- {1,2,3,4}
- set((1,2,3,4))
set([[1,2],[3,4],[4,5]]) is incorrect because the argument given for the set must be iterable.
10. Explain monkey patching in Python.
The changes made to a class or a module during runtime are called monkey patches. This is possible in Python, as we can change the program while it is being executed.
Example of monkey patching in Python:
# monkeyy.py
class X:
def func(self):
print("func() is being called")
Here is how the behavior of a function changes at runtime with monkey patching:
import monkeyy def monkey_f(self): print("monkey_f() is being called") # Replacing the address of "func" with "monkey_f" monkeyy.X.func = monkey_f obj = monkeyy.X() # Calling the function "func" whose address was replaced with the function "monkey_f()" obj.func()
11. What are dataframes? What is its syntax?
Dataframes are two-dimensional mutable data structures or data aligned in the tabular form with labeled axes(rows and column).
Syntax:
pandas.DataFrame( data, index, columns, dtype)
12. What is the difference between series and dataframes?
In pandas, a Series is a one-dimensional array-like object that can hold any data type.
A Dataframe is a two-dimensional, table-like structure with columns that are potentially typed heterogeneously. DataFrames are a collection of Series objects with the same index.
13. What is the process for merging dataframes in Pandas?
The following functions allow the merging of dataframes in Pandas:
Append() for horizontal stacking:
data_frame1.append(data_frame2)
Concat() for vertical stacking and when the dataframes that have to be merged have the same column and similar fields:
pd.concat([data_frame1, data_frame2])
Join() to extract data from different dataframes with one or more columns in common:
data_frame1.join(data_frame2)
14. How do you identify missing values and deal with missing values in Dataframe?
To identify missing values in a dataframe, we use the isnull() and isna() functions.
missing_count=data_frame1.isnull().sum()
To handle missing values, we can either replace the missing values with 0:
df[‘col_name’].fillna(0)
or replace the missing values with the mean value of that column:
df[‘col_name’] = df[‘col_name’].fillna((df[‘col_name’].mean()))
15. What is regression?
It is a supervised machine learning algorithm technique that helps programmers find the correlation between variables. Based on the independent variable (x), it can also predict the value of the dependent variable(y). It is used for time series modeling, forecasting, prediction, and determining the causal-effect relationship between variables.
Types of regression:
- Linear Regression: It is used for continuous and numeric variables.
- Logistic Regression: It is used for continuous and categorical variables.
16. What is classification?
To predict a class label for a given example of input data, we use the predictive modeling process. Classification can be used to categorize the provided input into a label with similar features. It can be used to classify an email as spam. A few classification algorithms used in ML are:
- Random forest classifier
- Decision tree
- Support vector machine
17. How do you split the data in train and test dataset in Python?
We can use the scikit machine learning library along with the train_test_split function in Python to do this.
from sklearn.model_selection import train_test_split # test size = 30% and train = 70% X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.3, random_state=0)
18. Why do we refer to Set as unordered? Also, is it mutable or immutable?
The items in a set are a collection that cannot be accessed by their positions. They are referred to as ‘unordered’ as they don’t have a specific order or sequence like a list.
Since we can remove or add items from a set after it’s created, sets in Python are mutable. The items within the sets are immutable, as we cannot change them.
We can’t modify the elements themselves once they’re in the set, but we can add or remove elements from a set.
19. What is the difference between percentile and quantiles in Python?
Both are measures used to describe the distribution of a dataset by dividing it into equal portions. Percentiles are expressed as percentages, ranging from 0% to 100%. A quantile refers to dividing a dataset into equal portions, such as quartiles (four parts) or quintiles (five parts).
20. What is SVM?
Support Vector Machine (SVM) is a machine learning algorithm used for classification and regression tasks. It works by finding a hyperplane in a high-dimensional space that separates data points of different classes with a maximum margin. SVM is effective for both linear and non-linear data and is widely used in various applications, including image classification, text categorization, and pattern recognition.
21. Write a code to get the indices of N maximum values from a NumPy array.
import numpy as np
ar = np.array([1, 3, 2, 4, 5, 6])
print(ar.argsort()[-3:][::-1])
22. What is the easiest way to calculate percentiles when using Python?
We can use NumPy arrays and their functions for this. For example:
import numpy as np
a = np.array([1,2,3,4,5,6,7])
p = np.percentile(a, 50) #Returns the 50th percentile which is also the median
print(p)
23. Write a Python program to check if a given string is a palindrome in Python without using an iterative method.
Here is an example of a palindrome in Python.
def fun(string): s1 = string s = string[::-1] if s1 == s: return True else: return False print(fun("madam"))
24. Write a Python program to calculate the sum of a list of numbers.
Use the pop() method to remove the last object from a list:
def sum(num): if len(num) == 1: return num[0] # With only one element in the list, the sum result will be equal to the element. else: return num[0] + sum(num[1:]) print(sum([2, 4, 5, 6, 7]))
25. Write a program in Python to execute the Bubble sort algorithm.
def bubbleSort(x): n = len(x) # Traverse through all array elements for i in range(n-1): for j in range(0, n-i-1): if x[j] > x[j+1]: x[j], x[j+1] = x[j+1], x[j] # Driver code to test above arr = [25, 34, 47, 21, 22, 11, 37] bubbleSort(arr) print("Sorted array is:") for i in range(len(arr)): print(arr[i])
Output:
11,21,22,25,34,37,47
26. Write a program in Python to produce a Star triangle.
def Star_triangle(n): for x in range(n): print(' '*(n-x-1)+'*'*(2*x+1)) Star_triangle(9)
Output:
*
***
*****
*******
*********
***********
*************
***************
*****************
27. Write a program to produce the Fibonacci series in Python.
Here is a program to produce the Fibonacci series in Python.
n = int(input("number of terms? "))
n1, n2 = 0, 1
count = 0
if n <= 0:
print("Please enter a positive integer")
elif n == 1:
print("Fibonacci sequence upto", n, ":")
print(n1)
else:
print("Fibonacci sequence:")
while count < n:
print(n1)
nth = n1 + n2
n1 = n2
n2 = nth
count += 1
28. Write a program in Python to check if a number is prime.
num = 13 if num > 1: for i in range(2, int(num/2)+1): if (num % i) == 0: print(num, "is not a prime number") break else: print(num, "is a prime number") else: print(num, "is not a prime number")
Output:
13 is a prime number
29. Create a Python sorting algorithm for a dataset of numbers.
my_list = ["8", "4", "3", "6", "2"]
my_list = [int(i) for i in list]
my_list.sort()
print (my_list)
Output:
2,3,4,6,8
30. Write a Program to print ASCII Value of a character in Python.
x= 'a' # print the ASCII value of assigned character stored in x print(" ASCII value of '" + x + "' is", ord(x))
Output: 65
31. How can you access this in Python?
Dataset in Csv format on Google Spreadsheet shared publicly.
We can use the following code:
>>link = https://docs.google.com/spreadsheets/d/…
>>source = StringIO.StringIO(requests.get(link).content))
>>data = pd.read_csv(source)
32. What is the difference between these two data series:
df[‘Name’] and df.loc[:, ‘Name’], where:
df = pd.DataFrame([‘aa’, ‘bb’, ‘xx’, ‘uu’], [21, 16, 50, 33], columns = [‘Name’, ‘Age’])
The difference is that both are copies of the original dataframe.
33. How will you correct this error:
Traceback (most recent call last): File “<input>”, line 1, in<module> UnicodeEncodeError:
‘ascii’ codec can’t encode character.
The error is about the difference between utf-8 coding and a Unicode. The following can fix it:
pd.read_csv(“temp.csv”, encoding=’utf-8′)
34. Explain the use of this code: In line two, write plt.plot([1,2,3,4], lw=3)
It can be used to create a simple line width in a given plot.
35. What does this code do: df.reindex_like(new_index,)
It can be used to reset the index of a dataframe to a given list.
36. Mention any five benefits of Flask.
- It offers incorporated unit testing support.
- It allows the use of cookies.
- It has a built-in development server as well as a rapid debugger.
- It has an HTTP request processing function.
- It has WSGI 1.0 interface that is compatible with jinja2.
37. How are Django, Flask, and Pyramid different?
Django | Flask | Pyramid |
---|---|---|
Python framework | Micro framework | Python framework |
Includes an ORM | Does not require external libraries | Offers flexibility and the right tools |
Used to develop large applications | Also used to build large applications | Also used to create small applications |
38. What is the use of sessions in the Django framework?
In Django, sessions are used to store and retrieve user-specific data between different requests. They are used to store user preferences, for user authentication, or to track user activity on a website. Additionally, Django’s session framework abstracts away the underlying storage mechanism, making it easy to switch between different session engines without changing the application code.
39. Write a program that combines two dictionaries. If you locate the same keys when combining them, you can sum the values of these similar keys. Create a new dictionary.
from collections import Counter
d1 = {'key1': 50, 'key2': 100, 'key3':200}
d2 = {'key1': 200, 'key2': 100, 'key4':300}
new_dict = Counter(d1) + Counter(d2)
print(new_dict)
40. How many inheritance styles does Django have?
There are three inheritance styles:
- Abstract base class: It is used when we need only the parent’s class to hold information that we don’t want to type out for each child model.
- Multi-table inheritance: It is used to sub-class an existing model when we need each model to have its own database table.
- Proxy model: It changes the Python level behavior of the model without modifying the model’s fields.
41. What are Keywords in Python?
In Python, keywords are fixed words that have special meaning. Keywords cannot be used for variable or function names but are used to define types of variables. There are 33 keywords in Python:
false | none | class | true | and | as |
assert | break | continue | def | del | elif |
else | except | finally | for | from | global |
if | import | in | is | lambda | nonlocal |
not | nor | pass | raise | return | try |
while | with | yield |
42. How to access the first five entries of a dataframe?
We can use the head(5) function to access the first five entries of a dataframe.
df.head() returns the top 5 rows by default.
df.head(n) is used to get the top n rows.
43. How to access the last five entries of a dataframe?
Use the tail(5) function to get the top five entries of a dataframe.
df.tail() returns the top 5 rows by default.
Use df.tail(n) to get the last n rows.
44. Write a code that fetches a data entry from a Pandas dataframe using a given value in index.
import pandas as pd bikes=["bajaj","tvs","herohonda","kawasaki","bmw"] cars=["lamborghini","masserati","ferrari","hyundai","ford"] d={"cars":cars,"bikes":bikes} df=pd.DataFrame(d) a=[10,20,30,40,50] df.index=a df.loc[10]
45. Write a code to create a new column in Pandas by using values from other columns.
import pandas as pd a=[1,2,3] b=[2,3,5] d={"col1":a,"col2":b} df=pd.DataFrame(d) df["Sum"]=df["col1"]+df["col2"] df["Difference"]=df["col1"]-df["col2"] df
46. Write a code to delete a column or group of columns in Pandas.
d={"col1":[1,2,3],"col2":["A","B","C"]} df=pd.DataFrame(d) df=df.drop(["col1"],axis=1) df
47. Build a decision tree classification model on an iris dataset. The dependent variable =“Species” and the independent variable = “Sepal.Length”.
y = iris[[‘Species’]] x = iris[[‘Sepal.Length’]] from sklearn.model_selection import train_test_split x_train,x_test,y_train,y_test=train_test_split(x,y,test_size=0.4) from sklearn.tree import DecisionTreeClassifier dtc = DecisionTreeClassifier() dtc.fit(x_train,y_train) y_pred=dtc.predict(x_test) from sklearn.metrics import confusion_matrix confusion_matrix(y_test,y_pred) (22+7+9)/(22+2+0+7+7+11+1+1+9)
48. How will you scrape data from the website “Cricbuzz”?
import sys import time from bs4 import BeautifulSoup import requests import pandas as pd try: #use the browser to get the url. This is suspicious command that might blow up. page=requests.get(‘cricbuzz.com’) # this might throw an exception if something goes wrong. except Exception as e: # this describes what to do if an exception is thrown error_type, error_obj, error_info = sys.exc_info() # get the exception information print (‘ERROR FOR LINK:’,url) #print the link that cause the problem print (error_type, ‘Line:’, error_info.tb_lineno) #print error info and line that threw the exception #ignore this page. Abandon this and go back. time.sleep(2) soup=BeautifulSoup(page.text,’html.parser’) links=soup.find_all(‘span’,attrs={‘class’:’w_tle’}) links for i in links: print(i.text) print(“\n”)
49. Write code to perform sentiment analysis on Amazon reviews.
import pandas as pd import numpy as np import matplotlib.pyplot as plt from tensorflow.python.keras import models, layers, optimizers import tensorflow from tensorflow.keras.preprocessing.text import Tokenizer, text_to_word_sequence from tensorflow.keras.preprocessing.sequence import pad_sequences import bz2 from sklearn.metrics import f1_score, roc_auc_score, accuracy_score import re %matplotlib inline def get_labels_and_texts(file): labels = [] texts = [] for line in bz2.BZ2File(file): x line.decode(“utf-8”) labels.append(int(x[9]) – 1) texts.append(x[10:].strip()) return np.array(labels), texts train_labels, train_texts = get_labels_and_texts(‘train.ft.txt.bz2’) test_labels, test_texts = get_labels_and_texts(‘test.ft.txt.bz2’) Train_labels[0] Train_texts[0] train_labels=train_labels[0:500] train_texts=train_texts[0:500] import re NON_ALPHANUM = re.compile(r'[\W]’) NON_ASCII = re.compile(r'[^a-z0-1\s]’) def normalize_texts(texts): normalized_texts = [] for text in texts: lower = text.lower() no_punctuation = NON_ALPHANUM.sub(r’ ‘, lower) no_non_ascii = NON_ASCII.sub(r”, no_punctuation) normalized_texts.append(no_non_ascii) return normalized_texts train_texts = normalize_texts(train_texts) test_texts = normalize_texts(test_texts) from sklearn.feature_extraction.text import CountVectorizer cv = CountVectorizer(binary=True) cv.fit(train_texts) X = cv.transform(train_texts) X_test = cv.transform(test_texts) from sklearn.linear_model import LogisticRegression from sklearn.metrics import accuracy_score from sklearn.model_selection import train_test_split X_train, X_val, y_train, y_val = train_test_split( X, train_labels, train_size = 0.75) for c in [0.01, 0.05, 0.25, 0.5, 1]: lr = LogisticRegression(C=c) lr.fit(X_train, y_train) print (“Accuracy for C=%s: %s” % (c, accuracy_score(y_val, lr.predict(X_val)))) lr.predict(X_test[29])
50. How will you Implement multiple linear regression on this iris dataset?
import numpy as np import pylab import scipy.stats as stats from matplotlib import pyplot as plt n1=np.random.normal(loc=0,scale=1,size=1000) np.percentile(n1,100) n1=np.random.normal(loc=20,scale=3,size=100) stats.probplot(n1,dist=”norm”,plot=pylab) plt.show()
51. Write a code to add a new column to Pandas data frame
Independent variables = “Sepal.Width”, “Petal.Length”, “Petal.Width”
Dependent variable = “Sepal.Length”
Solution:
import pandas as pd iris = pd.read_csv(“iris.csv”) iris.head() x = iris[[‘Sepal.Width’,’Petal.Length’,’Petal.Width’]] y = iris[[‘Sepal.Length’]] from sklearn.model_selection import train_test_split x_train, x_test, y_train, y_test = train_test_split(x, y, test_size = 0.35) from sklearn.linear_model import LinearRegression lr = LinearRegression() lr.fit(x_train, y_train) y_pred = lr.predict(x_test) from sklearn.metrics import mean_squared_error mean_squared_error(y_test, y_pred)
Code solution:
Import the required libraries:
import pandas as pd iris = pd.read_csv(“iris.csv”) iris.head()
Extract the independent variables and dependent variable:
x = iris[[‘Sepal.Width’,’Petal.Length’,’Petal.Width’]] y = iris[[‘Sepal.Length’]]
Divide the data into train and test sets:
from sklearn.model_selection import train_test_split x_train, x_test, y_train, y_test = train_test_split(x, y, test_size = 0.35)
Build the model:
from sklearn.linear_model import LinearRegression lr = LinearRegression() lr.fit(x_train, y_train) y_pred = lr.predict(x_test)
Find out the mean squared error:
from sklearn.metrics import mean_squared_error mean_squared_error(y_test, y_pred)
52. How will you access the 2nd, 4th and 5th elements from this list: [1,”a”,2,”b”,3,”c”]
First, create a tuple with the indices of elements to be accessed. Then, check the index values with the help of a for loop and print them out.
Code:
indices = (1,3,4)
for i in indices:
print(a[i])
53. Write a code to print the factorial of a number.
factorial = 1 #check if the number is negative, positive or zero if num<0: print("Sorry, factorial does not exist for negative numbers") elif num==0: print("The factorial of 0 is 1") else for i in range(1,num+1): factorial = factorial*i print("The factorial of",num,"is",factorial)
54. How can you set up the Database in Django?
The settings.py file will have all the database connection information and the settings of the project. As we know, Django works with SQLite database by default but can be configured to work with other databases as well.
We need to establish a connection between the application and the database by using the django.db.backends.mysql driver. This will connect to MySQL. We must remember to include all connection information in the settings file. Here is a code for the database:
DATABASES = { 'default': { 'ENGINE': 'django.db.backends.mysql', 'NAME': 'djangoApp', 'USER':'root', 'PASSWORD':'mysql', 'HOST':'localhost', 'PORT':'3306' } }
This code will build tables for admin, auth, content types, and sessions. We can also connect to the MySQL database by selecting it from the database drop-down menu.
55. Give an example of how you can write a VIEW in Django.
The view function in Python takes a web request and returns a web response. The responses can range from anything, such as the HTML contents of a web page, a redirect, or a 404 error. As long as the code is on your Python path, it can live anywhere, but the convention is to put views in a file called views.py, placed in your project or application directory.
Here is a code for a view that returns the current date and time, as an HTML document:
from django.http import HttpResponse import datetime def current_datetime(request): now = datetime.datetime.now() html = "<html><body>It is now %s.</body></html>" % now return HttpResponse(html)
56. Write a code to convert a list to a string.
fruits = [ ‘apple’, ‘orange’, ‘mango’, ‘papaya’, ‘guava’] listAsString = ‘ ‘.join(fruits) print(listAsString)
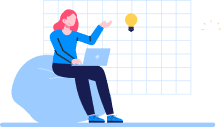
Don't miss out on your chance to work with the best!
Apply for top job opportunities today!

Conclusion
As an experienced Python programmer, it is obvious that you need to be proficient in all levels of Python. But more importantly, it is crucial to showcase your ability to apply Python in the real world. You may be asked to write code for an IoT-enabled device function or debug a program written for a game development company. It is important to understand that there is no template when it comes to appearing for interviews as an experienced candidate. There can be questions that test your database knowledge and observe your problem-solving skills. Employers might want to evaluate you as a candidate who is confident in accepting challenges that drive innovation and efficiency. In this blog, we have included the common questions that can be expected in interviews. However, it is imperative that, as a candidate, you build on your knowledge by continuous learning. Also, if you are seasoned Python developer looking for exciting job opportunities then sign up with Olibr now! All the best with your interviews!
FAQs
Here are a few intermediate Python programming jobs:
- Ensure your resume is updated.
- Be well-versed with the frameworks, data structures, functions, and libraries in Python.
- Practice coding by solving problems, working on small projects, and writing sample programs.
- Explain your thought process clearly for technical questions.
- Prepare for soft skills questions about communication, leadership, and teamwork.
Clearing a Python coding interview round can be a challenging stage. This is because it evaluates your programming skills through real-world problems or business use cases. The best way to be well-prepared for this round is to practice data structures and algorithms for the coding challenges.
- Obtain proficiency in basic Python skills.
- Master the intermediate Python concepts.
- Stay connected with the Python community.
- Build a portfolio of projects.
- Keep working on projects.
Here are some job profiles found on the Python job board:
- Senior API Engineer – AI Solutions Development
- Senior Python Backend Engineer
- Python Django E-Commerce Full Stack Developer
- Senior Python Architect & Tech Lead
- Security Researcher
- Senior RPA Python Developer
Take control of your career and land your dream job!
Sign up and start applying to the best opportunities!
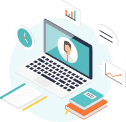