Table of Contents
Toggle
Introduction
Read in detail: WHAT IS GOLANG? WHAT IS IT USED FOR?
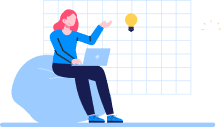
Don't miss out on your chance to work with the best
Apply for top global job opportunities today!

Top 50 Intermediate Interview Questions and Answers
1. What are Goroutines in Go?
Goroutines are lightweight threads managed by the Go runtime. They allow concurrent execution of functions.
2. How are Goroutines different from threads?
Goroutines are more efficient than OS threads because they use smaller stacks and multiplex onto fewer threads.
3. Explain the purpose and usage of the select statement in Go.
The select statement lets a goroutine wait on multiple communication operations. It blocks until one of its cases can run, then executes that case.
4. What is a nil pointer in Go, and how can it cause runtime errors?
A nil pointer points to no object, and dereferencing it leads to a runtime panic. Always check if a pointer is nil before dereferencing it.
5. How does Go handle errors, and what is the idiomatic way to return and handle errors?
Go uses error values to indicate errors. The idiomatic way is to return an error as the last return value and check it using if err != nil.
6. What is the difference between a slice and an array in Go?
7. How do you handle concurrency in Go?
8. What is a channel and how is it used in Go?
9. Explain the purpose of the defer statement.
10. How does Go's garbage collector work?
11. What is the use of sync.Mutex in Go?
sync.Mutex is used to prevent race conditions by providing mutual exclusion, ensuring only one goroutine accesses critical sections of code at a time.
12. Explain the difference between buffered and unbuffered channels.
13. What are Go interfaces, and how are they used?
Interfaces in Go are a way to specify the behavior of an object. They are satisfied implicitly by any type that implements their methods.
14. How do you implement dependency injection in Go?
15. Explain the use of context in Go.
16. What is a closure in Go?
17. How do you ensure a slice is not nil and has a default capacity?
Use make([]Type, 0, capacity) to create a non-nil slice with a default capacity.
18. What are variadic functions in Go?
19. How does Go handle memory allocation?
Go handles memory allocation using the built-in new and make functions. new allocates zeroed storage, while make is used for slices, maps, and channels.
20. Explain how Go’s type assertion works.
21. What are Go maps and how do you handle concurrent access to them?
22. What is a method set in Go?
A method set defines the set of methods attached to a type, which determines the interfaces the type implements.
23. How can you implement a thread-safe singleton in Go?
Use sync.Once to ensure a piece of code runs only once, making a thread-safe singleton pattern.
24. How do you convert a string to a byte slice and vice versa in Go?
25. What is the init function in Go?
The init function is called before the main function, used to initialize package-level variables.
26. Explain Go's build tags and their usage.
Build tags control which files are included in the package. They are set using // +build tagname comments at the top of the file.
27. How do you handle timeouts in Go?
Use the context package with context.WithTimeout or time.After for handling timeouts.
28. What are goroutine leaks and how can you avoid them?
29. Explain how to write a custom error type in Go.
Also Read: TOP 10 GOLANG FRAMEWORKS FOR WEB DEVELOPMENT
30. How do you perform JSON marshalling and unmarshalling in Go?
Use json.Marshal to convert Go structs to JSON and json.Unmarshal to convert JSON to Go structs.
31. What is the sync.WaitGroup used for?
32. How can you execute shell commands in Go?
33. Explain the purpose of Go modules.
34. How do you define and use generics in Go?
As of Go 1.18, generics are implemented using type parameters. Syntax: func Foo[T any](arg T) { … }
35. What is the panic and recover mechanism in Go?
36. Explain the difference between new and make.
37. How do you create and manage logs in Go?
Use the log package to create logs. You can customize output, add prefixes, and set flags for formatting.
38. What are Go’s testing tools and how do you use them?
39. Explain the use of iota in Go.
40. How can you handle configuration in a Go application?
41. How do you work with pointers to struct fields in Go?
42. What is Go’s race detector and how do you use it?
43. How do you manage dependencies in a Go project?
44. What is the purpose of the sync.Cond type?
45. How do you copy a slice in Go?
46. Explain Go’s approach to OOP principles.
47. How do you benchmark code in Go?
48. What are method receivers and how are they used?
49. How do you create a new Go project with modules?
50. What is a type switch in Go?

Conclusion
Preparing for an intermediate Golang interview requires a solid grasp of the language’s core concepts as well as practical experience with its more advanced features. The questions we’ve covered in this blog are designed to test your understanding and help you identify areas where you might need further study. By practising these questions and understanding the underlying principles, you can approach your interview with confidence. Remember, the key to excelling in any technical interview is not just about knowing the correct answers but also about demonstrating a clear, logical approach to problem-solving and a deep understanding of the tools at your disposal. Also, sign up with Olibr to find top opportunities for Golang Developers.
Take control of your career and land your dream job
Sign up with us now and start applying for the best opportunities!
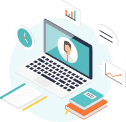
FAQs
Intermediate-level questions typically focus on deeper aspects of Golang, including concurrency, data structures, error handling, and more advanced language features. They often require a practical understanding and ability to apply concepts rather than just recalling syntax.
Start by reviewing fundamental concepts such as goroutines, channels, interfaces, and error handling. Practice coding exercises that involve these concepts to strengthen your understanding. Additionally, explore common design patterns and best practices in Go programming.
Topics usually include concurrency (goroutines, channels), data structures (maps, slices, structs), error handling (panic, recover, error interface), interfaces, packages, testing, and possibly advanced topics like reflection and concurrency patterns.
Both are important. While understanding theory is crucial for explaining concepts, practical coding skills are equally essential for solving problems efficiently during interviews. Practice coding exercises that simulate real-world scenarios.
Be prepared to discuss your thought process when solving problems, write clean and efficient code, and explain why you chose a particular approach. Show familiarity with Go’s idioms and conventions.
Don’t panic. Clarify the question with the interviewer if needed. If a topic is unfamiliar, admit it honestly and demonstrate your willingness to learn. Try to relate it to similar concepts you know.
Utilize online platforms like LeetCode, HackerRank, or Exercism for practicing coding problems. Refer to books such as “The Go Programming Language” by Alan Donovan and Brian Kernighan. Also, explore Go’s official documentation and community forums for insights and discussions.
Extremely important. Go’s concurrency features (goroutines and channels) are powerful and distinctive. Employers often assess candidates’ ability to manage concurrent tasks effectively and safely.