Table of Contents
Toggle
Introduction
The advancements in technology have increased cross-platform app development. As a result, many companies looking to hire React Native developers seek professionals with more than a beginner-level experience of various technologies. As you progress beyond the basics of React Native, it is important to be ready to take on more complex tasks as an intermediate React Native developer. This blog will help you evaluate yourself as an intermediate developer as you go through the React Native interview questions and answers. Read on to learn more about the tools and technologies used in React Native!
Must Read: REACT NATIVE BASIC INTERVIEW QUESTIONS
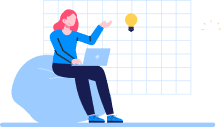
Don't miss out on your chance to work with the best
Apply for top global job opportunities today!

Top React Native Intermediate Questions and Answers
1. How to process an entire React Native code to show the final output on the screen?
- The main thread starts execution at the first start of the app and starts loading JS bundles.
- After the JavaScript code has been loaded successfully, the main thread sends it to another JS thread. This ensures that the UI thread will not be affected when JS does heavy calculations.
- The Reconciler starts diffing when React starts rendering. When a new virtual DOM (layout) is generated, it sends changes to another thread(Shadow thread).
- The Shadow thread calculates layout and sends layout parameters/objects to the main(UI) thread.
- Only the main thread can render something on the screen. So, the shadow thread sends the generated layout to the main thread and only then UI renders.
2. What is a bridge and why is it used in React Native?
The bridge serves as a communication channel between the JavaScript (JS) thread and the native side of a React Native app. A bridge allows interaction between the JavaScript code (where your React Native logic resides) and the native components (such as UI views, sensors, and device features). It is possible to have seamless data exchange and method calls between the two realms.
3. Explain how a bridge is used in both Android and iOS.
Android
In Android, the bridge facilitates the following interactions:
- Native Modules: Developers can create custom native modules (Java classes) that expose functionality to JavaScript. These modules can access native features (e.g., sensors, camera) or perform custom logic.
- Communication: JavaScript code communicates with native modules via the bridge. For example:
- JavaScript invokes methods from native modules using NativeModules.
- Native modules can send data back to JavaScript using callbacks or promises.
- UI Components: React Native components (e.g., View, Text, Image) are mapped to native Android views. The bridge ensures that UI rendering happens natively.
iOS
In iOS, the bridge serves a similar purpose:
- Native Modules: Developers create custom native modules (Objective-C/Swift classes) to expose functionality to JavaScript. These modules can access platform APIs or provide custom features.
- Bidirectional Communication: JavaScript communicates with native modules using the bridge. For instance:
- JavaScript invokes methods from native modules using NativeModules.
- Native modules can send events or data back to JavaScript using callbacks or promises.
- UI Rendering: React Native components map to native iOS views (e.g., UIView, UILabel). The bridge ensures that UI components render natively.
4. Name core components in React Native and the analogy of those components when compared with the web.
REACT Native UI Component | Android View | iOS View | Web Analog | Description |
---|---|---|---|---|
< View > | <ViewGroup> | < UIView > | A non-scrolling <div> | A container that supports layout with flexbox style, some touch handling, and accessibility controls. |
< Text > | < TextView > | <UITextView> | < p > | Displays, styles, and nests strings of text and even handles touch events. |
< Image > | <ImageView > | <UIImageView> | < img > | Displays different types of images |
< ScrollView > | <ScrollView > | <UIScrollView> | < div > | A generic scrolling container that can contain multiple components and views. |
< TextInput > | < EditText > | <UITextField> | <input type=”text”> | Allows the user to enter text |
5. What is ListView in React Native?
A List View in React Native is a view component that contains the list of items and displays it in a vertically scrollable list.
Example Code
export default class MyListComponent extends Component {
constructor() {
super();
const ds = new ListView.DataSource({rowHasChanged: (r1, r2) => r1 !== r2});
this.state = {
dataSource: ds.cloneWithRows(['Android','iOS', 'Java','Php', 'Hadoop', 'Sap', 'Python','Ajax', 'C++']),
};
}
render() {
return (
<ListView
dataSource={this.state.dataSource}
renderRow={
(rowData) =>
<Text style={{fontSize: 30}}>{rowData}</Text>} />
); }
}
6. How does React Native achieve native performance?
React Native achieves native performance through a combination of smart design choices and optimizations:
Native Components: React Native uses native components (e.g., UIView, UILabel on iOS) instead of web-based components. This ensures efficient rendering and responsiveness.
GPU Utilization: By leveraging the device’s Graphics Processing Unit (GPU), React Native achieves near-native performance. UI animations, transitions, and rendering benefit from GPU acceleration.
Differential Rendering: React Native employs a Virtual DOM and differential rendering. It compares the current Virtual DOM with the updated one, selectively updating only changed components. This minimizes costly reflows and repaints.
JavaScript Thread Optimization: React Native strives to keep the JavaScript thread responsive. If any operation takes longer than 100ms, it impacts user experience. By optimizing JavaScript execution, React Native ensures smooth animations and interactions.
7. How can you write different code for iOS and Android in the same code base?
The Platform Module in React Native detects the platform in which the app is running.
For example:
import { Platform, Stylesheet } from 'react-native';
const styles = Stylesheet.create({
height: Platform.OS === 'IOS' ? 200 : 400
})
The Platform.select method takes an object containing Platform.OS as keys and returns the value for the platform you are currently on.
For example:
import { Platform, StyleSheet } from 'react-native';
const styles = StyleSheet.create({
container: {
flex: 1,
...Platform.select({
ios: {
backgroundColor: 'red',
},
android: {
backgroundColor: 'green',
},
default: {
// other platforms, web for example
backgroundColor: 'blue',
}, }),
},
});
8. What are the key features of FlatList components in React Native? Give an example code.
The FlatList component is useful with large data lists where list items might change over time. It shows similarly structured data in a scrollable list. The FlatList does not show all elements of the list at once. It only shows the rendered elements currently displayed on the screen.
Example code:
import React, { Component } from 'react';
import { AppRegistry, FlatList,
StyleSheet, Text, View,Alert } from 'react-native';
export default class FlatListBasics extends Component {
renderSeparator = () => {
return (
<View
style={{
height: 1,
width: "100%",
backgroundColor: "#000",
}}
/>
);
};
//handling onPress action
getListViewItem = (item) => {
Alert.alert(item.key);
}
render() {
return (
<View style={styles.container}>
<FlatList
data={[
{key: 'Android'},{key: 'iOS'}, {key: 'Java'},{key: 'Swift'},
{key: 'Php'},{key: 'Hadoop'},{key: 'Sap'},
]}
renderItem={({item}) =>
<Text style={styles.item}
onPress={this.getListViewItem.bind(this, item)}>{item.key}</Text>}
ItemSeparatorComponent={this.renderSeparator}
/>
</View>
);
}
}
AppRegistry.registerComponent('AwesomeProject', () => FlatListBasics);
9. How to use Routing with React Navigation in React Native?
React Navigation is a library for routing and navigation in React Native applications. The library helps to navigate between multiple screens and share data between them. Here is a coding example of how routing is used with React Navigation in React Native.
import * as React from 'react';
import { NavigationContainer } from '@react-navigation/native';
import { createStackNavigator } from '@react-navigation/stack';
const Stack = createStackNavigator();
const MyStack = () => {
return (
<NavigationContainer>
<Stack.Navigator>
<Stack.Screen
name="Home"
component={HomeScreen}
options={{ title: 'Welcome' }}
/>
<Stack.Screen name="Profile" component={ProfileScreen} />
</Stack.Navigator>
</NavigationContainer>
);
};
10. How to use styled components in React Native application?
Styled components are used in React Native to write styles in normal CSS. It can be easily included in a project without any linking. All you need to do is run the following command in the root directory of your app to install it.
import React, {Component} from 'react';
import { StyleSheet,Text, View} from 'react-native';
import styled from 'styled-components'
const Container=styled.View`
flex:1;
padding:50px 0;
justify-content:center;
background-color:#f4f4f4;
align-items:center
`
const Title=styled.Text`
font-size:20px;
text-align:center;
color:red;
`
const Item=styled.View`
flex:1;
border:1px solid #ccc;
margin:2px 0;
border-radius:10px;
box-shadow:0 0 10px #ccc;
background-color:#fff;
width:80%;
padding:10px;
`
export default class App extends Component {
render() {
return (
<Container>
<Item >
<Title >Item number 1</Title>
</Item>
<Item >
<Title >Item number 2</Title>
</Item>
<Item >
<Title >Item number 3</Title>
</Item>
<Item >
<Title >Item number 4</Title>
</Item>
</Container>
);
}
11. What are the benefits of using Expo for React Native development?
- Expo simplifies setup by abstracting the complexity of native modules. This makes it easier for developers to build apps faster without worrying about platform-specific configuration.
- Expo’s ability to provide over-the-air updates upgrades apps without the need to download new versions from the app store.
- Users have access to several APIs to access device features like camera, location, and more
- The unified codebase of Expo allows developers to build for both iOS and Android using a single codebase.
12. What are the drawbacks of using Expo for React Native development?
- Expo is not a good choice if you need deep native integration. It has limited native module support as access to certain native modules is restricted.
- Since runtime is included in the Expo app package, it leads to larger compared to bare React Native projects.
- In case you change or discontinue Expo services it can impact the app’s functionality and maintenance.
- Expo offers limited customization as it does not have the tools complex where third-party native libraries must be integrated.
13. Where can you use bare workflows in Expo?
Bare workflows in Expo can be used in:
- Complex projects with specific requirements.
- Projects needing platform-specific features or extensive customization.
- Applications demanding optimized performance.
14. What is gesture handling in React Native?
Gesture handling in React Native refers to the ability to recognize and respond to user interactions such as taps, swipes, pinches, and scrolls. It allows developers to create interactive and intuitive mobile app interfaces by capturing touch gestures and translating them into specific actions or animations. React Native provides built-in components and libraries (such as react-native-gesture-handler) to facilitate efficient gesture recognition and handling. Whether it’s smooth scrolling, interactive animations, or custom gestures, mastering gesture handling ensures a delightful user experience in mobile apps.
15. How can you handle offline storage in a React Native app?
Offline storage in a React Native app can be handled using AsyncStorage.
Example code:
import AsyncStorage from '@react-native-async-storage/async-storage; // Save data const
saveData = async (key, value) => { try { await AsyncStorage.setltem(key, JSON.stringify(value));
} catch (error) { console.error ('Error saving data:", error); } }; // Retrieve data const getData =
async (key) => { try { const value = await AsyncStorage.getItem(key); return value ?
JSON.parse(value) : null; } catch (error) { console.error ('Error retrieving data:', error); } };
16. Give an example of shouldComponentUpdate in React Native.
class MyComponent extends React.Component { shouldComponentUpdate(ne*Props, nextState) {// Compare current props and state with nextProps and nextState // Return true to allow the component to update, or false to prevent it returning this.props.someValue !== nextProps.someValue; } render) { // Render component content } }
17. How can you achieve a responsive design in React Native with Flexbox and Dimension API?
Responsive design in React Native can be achieved by creating layouts that adapt effectively to different screen sizes and orientations. Here is how it can be done using Flexbox and Dimensions API:
Flexbox: React Native uses Flexbox for layout which allows components to dynamically adjust their size and position based on available space. It’s crucial for creating responsive designs.
- flexDirection: Determines the main axis direction (row or column).
- alignItems: Aligns items along the cross axis (e.g., vertically for a row layout).
- justifyContent: Positions items along the main axis (e.g., horizontally for a column layout).
Example:
const styles = StyleSheet.create({
container: {
flex: 1,
flexDirection: 'row',
alignItems: 'center',
justifyContent: 'space-between',
},
});
Dimensions API: It provides information about the device screen dimensions. It can be used to create responsive layouts based on the available screen space.
Example:
import { Dimensions } from 'react-native';
const screenWidth = Dimensions.get('window').width;
const screenHeight = Dimensions.get('window').height;
18. What is the purpose of the PixelRatio module in React Native?
Since mobile devices have different pixel densities, it affects the way content is displayed. PixelRatio is a module in React Native that is used to handle screen density differences across devices. The module helps create visually appealing yet consistent designs on screen irrespective of the varying pixel densities. The PixelRatio.get() method can be used to adjust font sizes, dimensions, and other visual elements as it returns the device’s pixel density as a number.
import { PixelRatio} from 'react-native'; const fontScale = PixelRatio.getFontScale); const
devicePixelDensity = PixelRatio.get); const adjustedFontSize = 16 * fontScale; // Adjust font
size based on device's font scale const adjustedWidth = Pixe|Ratio.roundToNearestPixel(100 *
devicePixelDensity); I/ Adjust width based on device's pixel density
19. Give an example code to handle push notifications in a React Native app.
Push notifications help with user engagement. Here are the steps to handle push notifications in a React Native app:
- Set up push notification services using FCM or APNs for iOS.
- Request permissions using the react-native-push-notification or @react-native-community/push-notification-ios library to request notification permissions from users.
- Use a push notification service to register the device token as it is necessary for sending notifications to the device.
- Handle notifications by configuring the app to manage the notifications. This step will help you define custom behaviors based on notification data.
- Display notifications through the notification library.
Example code:
import PushNotification from 'react-native-push-notification'; // Request permissions and set up handlers
PushNotification.configure{ onRegister: function (token) { // Send the token to your server }, onNotification:
function (notification) { // Handle incoming notification }, onAction: function (notification) {// Handle notification
actions (e.g., tapping on it) }, onRegistrationError: function (error) { console.error ('Push notification
registration error:", error); }, });
20. Explain the Virtual DOM and its relevance in React Native.
It is a great optimization technique at the core of React’s performance and efficiency. Here is how it works in the context of React Native:
Initial Render
- When your React Native app loads, it creates a Virtual DOM representation of the UI.
- This lightweight JavaScript object mirrors the actual UI structure.
Updating State
- When a change occurs (e.g., button click or data fetch), React Native updates the component state.
- It generates a new Virtual DOM representing the updated state.
Differential Rendering
- During reconciliation, React Native compares the current Virtual DOM with the new one.
- It identifies differences (often called “diffing”) and selectively updates only the changed components in the actual DOM.
- This process minimizes costly reflows and repaints, resulting in faster rendering.
21. What is NativeBase and how does it simplify React Native development?
NativeBase is an open-source UI component library for React Native. It has platform-specific components that are easily accessible, pre-designed, and can be customized. Some key features of NativeBase are:
- It offers many ready-to-use components like buttons, cards, headers, tabs, and more, which can be easily integrated into an app.
- Based on the platform, components adapt their appearance automatically allowing platform-specific styling and a native look for iOS and Android.
- The default style customization allows developers to use components that the app’s branding and design
- The components can be easily imported and used in code which reduces the need for manual styling.
- NativeBase components have a responsive design, which allows them to work across various screen sizes and orientations.
- The module also allows developers to create and apply themes consistently across different screens and components.
22. How can you implement bi-directional communication in React Native?
Bi-directional communication in React Native refers to the ability of data exchange between two entities (usually the client and the server) in both directions. Here are ways to achieve bi-directional communication in React Native:
- Use props to pass data from parent to child components. Child components can trigger events by using callbacks passed from the parent as they receive data as props.
- Use Context API to share data between components not directly connected in the component tree. With this, you can avoid passing props through intermediate components.
- Global state management is possible with libraries like Redux and MobX. The libraries also allow any component to access and modify the shared state.
- Child components can emit events or callbacks provided by the parent components to communicate changes or trigger actions.
- React hooks can be used to enclose the logic and share it across components, allowing developers to reuse the behavior.
- Native modules can be built for complex scenarios to expose native functionality to JavaScript and vice versa.
23. Discuss the role of SafeAreaView in React Native and why it's important.
SafeAreaView in React Native is a component designed to render content within the safe area boundaries of a device. It is particularly relevant for iOS devices with iOS version 11 or later. SafeAreaView ensures that your app’s content avoids overlapping with areas like the top status bar, bottom navigation bars, or notches (such as the sensor housing area on iPhone 13). Here is why SafeAreaView is important in React Native:
Safe insets: Status bars, notches, and navigation bars can be of different sizes and shapes across devices. SafeAreaView helps in preventing content obstruction as it calculates and applies insets to your content.
Consistent UI: SafeAreaView ensures that the crucial UI elements and content are always accessible and visible.
Platform specifics: SafeAreaView takes into account platform-specific guidelines and adjusts the layout automatically based on the device’s platform.
Ease of use: You can wrap the top-level components or screens with SafeAreaView so that insets can be handled efficiently.
Here is a basic code to help understand how to use SafeView:
import { SafeAreaView } from 'react-native'; function App() { return (<SafeAreaView style= flex:
1 }}> {/* Your app's content */ </SafeAreaView>); }
24. How to implement a custom font in a React Native app?
Here is an example of how you can implement a custom font:
import React from 'react; import { Text, View } from 'react-native"; import Icon from
'react-native-vector-icons/FontAwesome'; const App = () => { return ( <View> <Text style=(
fontFamily: 'CustomFont, fontSize: 20 }}> Custom Font Example </Text> <Icon name="rocket" size=(30} color="#900" /> </View>): }; export default App;
A custom font for a ReactNative app can be implemented in the following way:
Download the Custom Font
Find the custom font you want to use (e.g., from Google Fonts). Download the font files (usually in TTF or OTF format).
Create an Assets Folder
In your React Native project, create an assets folder in the root directory (if it doesn’t exist). Inside the assets folder, create a subfolder called fonts.
Add Font Files
Paste the downloaded font files (TTF or OTF) into the fonts folder. Ensure the font files have the correct names (e.g., MyCustomFont-Regular.ttf).
Configure react-native.config.js
Create a react-native.config.js file in the root directory (if it doesn’t exist). Add the following JS code to the file:
module.exports = {
project: {
ios: {},
android: {},
},
assets: ['./assets/fonts'],
};
Link the fonts
Link the fonts by running this command in your terminal: npx react-native link
Use the custom font
Reference the custom font using the fontFamily property in the styles or component:
const styles = StyleSheet.create({
customText: {
fontFamily: 'MyCustomFont-Regular',
fontSize: 16,
// Other styling properties...
},
});
Reload the app
Reload your app (either by restarting the Metro bundler or rebuilding the app).
25. Explain the purpose of the AppState module in React Native.
The AppState module in React Native provides information about the current state of the app (e.g., whether it’s in the foreground, background, or transitioning). It’s frequently used to handle scenarios like updating push notification behavior based on the app’s visibility. By monitoring changes in app state, developers can ensure proper behavior and responsiveness in their applications. It is used to trigger background tasks or pause ongoing tasks when the app is sent to the background.
Example:
import { AppState } from 'react-native'; class App extends React.Component {
componentDidMount) { AppState.addEventListener('change', this.handleAppStateChange); }
componentWillUnmount) { AppState.removeEventListener('change,
this.handleAppStateChange); } handleAppStateChange = (nextAppState) => { if (nextAppState
=== 'active") { // App is in the foreground} else if (nextAppState === 'background') { // App is in the background } }; render) {// ... } }
26. Describe bridge communication in React Native.
The Bridge in React Native serves as a crucial layer that enables communication between JavaScript and Native modules. The bridge allows JavaScript code and Native modules to interact bidirectionally. The bridge ensures that UI components render natively, resulting in better performance. Events are dispatched through the bridge. React Native components respond to these events, updating the UI as needed.
Il JavaScript side import { NativeModules } from 'react-native'; // Call a native method
NativeModules.MyNativeModule.doSomethingAsync(data) .then(result => {// Handle the result
}) catch(error => { // Handle errors });
// Native (Android) side @ReactMethod public void doSomethingAsync(String data, Promise promise) {// Perform native operations asynchronously // Resolve or reject the promise based
on the result if (success) { promise.resolve(resultData); } else { promise.reject("ERROR_CODE",
"Error message"); } }
27. Explain the use of native modules in React Native.
Native modules are sets of JavaScript functions that are implemented natively for each platform (iOS and Android). They come into play when you need to access native capabilities that React Native doesn’t provide out of the box.
They are used when:
- You want to interact with platform-specific APIs (e.g., accessing Apple or Google Pay).
- You need to reuse existing Objective-C, Swift, Java, or C++ libraries without reimplementing them in JavaScript.
- You’re writing high-performance, multi-threaded code (e.g., for image processing).
Example (iOS)
// NativeModuleExample.h #import < React/RCTBridgeModule.h>
@interface
NativeModuleExample: NSObject < RCTBridgeModule > @end
// NativeModuleExample.m #import "NativeModuleExample.h" @implementation
NativeModuleExample RCT_EXPORT_MODULE();
RCT_EXPORT_METHOD(doSomething: (NSString *)input
resolver: (RCTPromiseResolveBlock)resolve rejecter:(RCTPromiseRejectBlock)reject) < //
Perform native operation asynchronously if (success) { resolve(result); } else {
reject(@"ERROR_
_CODE", @"Error message", nil); } } @end
28. What are the limitations of React Native in the context of Native API access?
React Native is a powerful framework for building cross-platform mobile apps, but it does have some limitations. React Native provides access to a wide range of native APIs, but not all features are accessible. Some native functionalities may be inaccessible or not fully supported, potentially limiting app complexity. For instance, accessing specific hardware features or low-level system APIs might be challenging.
29. How would you handle state synchronization between React components using component communication patterns?
Here is how you can synchronize between React components using component communication patterns:
Props: Pass data from parent to child components via props.
Callbacks: Pass functions as props to child components to allow them to update the parent’s state.
Event Bus/Custom Events: Create an event bus (a central event emitter) to communicate between unrelated components.
Render Props: Pass a function as a prop to a child component, allowing it to render content based on that function’s result.
30. Discuss the role of LayoutAnimation in creating smooth transitions in React Native.
LayoutAnimation in React Native is a powerful API that automatically animates views to their new positions when the next layout occurs. It’s particularly useful for creating smooth transitions during UI updates. When you update the layout (e.g., change the size or position of components), LayoutAnimation ensures that the transition happens seamlessly. It works by automatically animating views to their new positions, making your app feel more dynamic and responsive.
Example:
import { LayoutAnimation, View, Text, TouchableOpacity } from 'react-native'; import React, {
Component } from 'react'; class App extends Component { state = { expanded: false };
toggleExpansion = () => { LayoutAnimation.configureNext(LayoutAnimation.Presets.spring);
this.setState{ expanded: /this.state.expanded 7); }; render { const { expanded } = this.state; return (<View> <TouchableOpacity onPress={this.toggleExpansion}> <Text> Toggle Expansion</Text> </TouchableOpacity> <View style={{ height: expanded ? 200 : 50,
background Color: 'blue' }} /> </View>); } } export default App;
31. How can you implement background tasks in a React Native app through background fetch?
In React Native, handling background tasks is essential for scenarios like data synchronization, notifications, or periodic updates. Here is how you can implement background tasks in a React Native app through background fetch:
- The react-native-background-fetch library simplifies background task scheduling.
- It allows you to define tasks that run periodically, even when the app is in the background or terminated.
Example usage:
import BackgroundFetch from 'react-native-background-fetch';
BackgroundFetch.configure({
minimumFetchInterval: 15, // Minimum interval in minutes
stopOnTerminate: false, // Continue running after app termination
startOnBoot: true, // Start when the device boots up
}, async (taskId) => {
// Perform your background task here
console.log(`Background task with ID ${taskId} executed`);
BackgroundFetch.finish(taskId); // Signal task completion
});
// Start the background task
BackgroundFetch.start();
32. How do you install React Navigation in your React Native project?
React Navigation is a powerful library for handling navigation in React Native apps. To install the necessary packages in your React Native project:
npm install @react-navigation/native
npm install react-native-screens react-native-safe-area-context
If you are using Expo, you can run:
npx expo install react-native-screens react-native-safe-area-context
33. Write a code to create a simple stack navigator with two screens.
import React from 'react';
import { NavigationContainer } from '@react-navigation/native';
import { createNativeStackNavigator } from 'react-native-screens/native-stack';
const Stack = createNativeStackNavigator();
const App = () => {
return (
<NavigationContainer>
<Stack.Navigator>
<Stack.Screen name="Home" component={HomeScreen} />
<Stack.Screen name="Details" component={DetailsScreen} />
</Stack.Navigator>
</NavigationContainer>
);
};
// Define HomeScreen and DetailsScreen components
// (These can be functional components or class components)
const HomeScreen = ({ navigation }) => {
return (
// Your Home screen UI here
);
};
const DetailsScreen = ({ navigation }) => {
return (
// Your Details screen UI here
);
};
export default App;
34. What is the difference between Hot Reloading and Live reloading?
Hot Reloading and Live Reloading are features in React Native that enhance the development experience by allowing developers to see immediate code changes without manual refreshes.
Hot Reloading
- Hot Reloading automatically refreshes only the modified code files while preserving the app’s current state.
- For instance, if you’re deep into your app’s navigation and save a change to styling, the state remains intact, and the new styles appear without navigating back to the same page.
Live Reloading
- Live Reloading reloads the entire app whenever any file changes occur.
- If you’re deep into navigation and save a change, the app restarts and loads back to the initial route.
- It discards the current state, making it less suitable for preserving complex app states during development.
35. What is the use of the ScrollView component?
The ScrollView component in React Native serves as a versatile scrolling container for multiple components and views. ScrollView allows you to create scrollable content, both vertically and horizontally (by setting the horizontal property). You can mix different types of components (text, images, custom views) within a single ScrollView.
By enabling the pagingEnabled prop, you can implement swiping gestures between views.
On Android, you can achieve horizontal swiping using the ViewPager component.

Conclusion
A good grasp of the intermediate React Native concepts increases your chance of cracking interviews for React Native jobs. In this blog, we have covered the major intermediate React Native concepts and added example codes that could give you a broad overview of how you need to prepare for an upcoming interview. Our clients are looking to hire React Native developers. If you are a react native developer looking for top job opportunities then sign up with Olibr now!
Take control of your career and land your dream job
Sign up with us now and start applying for the best opportunities!
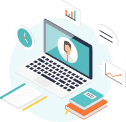
FAQs
React Native is not a programming language. It is an open-source UI software framework created by Meta. It helps developers to build native mobile applications using JavaScript.
Apart from React Native, some other JS frameworks for mobile apps are Sencha Ext Js, Vue JS, Ember JS, Node.js, and Meteor JS.
Node.js and React Native serve different purposes and are not directly comparable. Node.js is a back-end runtime environment, whereas React Native is a framework for building cross-platform mobile applications using JavaScript.
Yes, you can combine React Native and Node.js to create a full-stack development environment.
React interview questions for experienced developers often delve into advanced topics such as state management, performance optimization, component lifecycle methods, React hooks, server-side rendering, testing methodologies, architectural patterns, and integration with other libraries and frameworks.
React is a JavaScript library for building user interfaces for web applications, while React Native is a framework for building cross-platform mobile applications using JavaScript and React.