Table of Contents
Toggle
Introduction
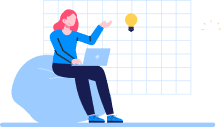
Don't miss out on your chance to work with the best
Apply for top global job opportunities today!

Top 50 Golang Interview Questions and Answers for Beginners
1. What is Golang?
Read in detail: WHAT IS GOLANG? WHAT IS IT USED FOR?
2. Who developed Golang and when?
3. What are the main features of Go?
4. How do you declare a variable in Go?
Variables can be declared using the var keyword or the shorthand :
= syntax.
var x int
y := 10
5. What is a goroutine?
6. How do you start a goroutine?
go func() {
fmt.Println("Hello, goroutine!")
}()
7. What is a channel in Go?
8. How do you declare and use a channel?
ch := make(chan int)
ch <- 1 // Send a value to the channel
value := <-ch // Receive a value from the channel
9. What is the purpose of the select statement in Go?
10. How do you handle errors in Go?
if err != nil {
fmt.Println("Error:", err)
}
11. What is a Go package?
12. How do you import a package in Go?
13. What is the init function in Go?
14. What is the difference between := and = in Go?
:= is used for short variable declaration and initialization, while = is used for assignment.
15. What are slices in Go?
Also Read: TOP 10 GOLANG FRAMEWORKS FOR WEB DEVELOPMENT
16. How do you create a slice in Go?
arr := [5]int{1, 2, 3, 4, 5}
s := arr[1:4]
17. What is a struct in Go?
A struct is a composite data type that groups together variables under a single name. Each variable in a struct is called a field.
18. How do you define and initialize a struct in Go?
type Person struct {
Name string
Age int
}
p := Person{Name: "Alice", Age: 30}
19. What are interfaces in Go?
Interfaces are types that specify a set of method signatures. A type implements an interface by implementing its methods.
20. How do you declare an interface in Go?
type Speaker interface {
Speak() string
}
21. How do you implement an interface in Go?
type Dog struct{}
func (d Dog) Speak() string {
return "Woof!"
}
22. What is the difference between a method and a function in Go?
func (p Person) Greet() {
fmt.Println("Hello, my name is", p.Name)
}
23. What is the purpose of the defer statement in Go?
The defer statement postpones the execution of a function until the surrounding function returns. It’s often used for cleanup tasks.
24. How do you create a new Go module?
25. What is the go fmt tool?
26. How do you run tests in Go?
You run tests using the ‘go test’ command. Test files should be named with _test.go suffix and contain functions starting with ‘Test’.
go test
27. What is a pointer in Go?
A pointer is a variable that holds the memory address of another variable.
28. How do you declare and use pointers in Go?
var p *int
x := 10
p = &x
fmt.Println(*p) // Outputs: 10
29. What is the zero value in Go?
30. What is the recover function in Go?
The recover function is used to regain control of a panicking goroutine. It is used in conjunction with defer to handle panic situations gracefully.
31. What are the built-in data types in Go?
32. How do you create a map in Go?
m := make(map[string]int)
m["key"] = 42
33. What is the range keyword used for in Go?
The range keyword is used to iterate over elements in arrays, slices, maps, and channels.
for index, value := range slice {
fmt.Println(index, value)
}
34. What is the difference between append and copy in Go?
append adds elements to the end of a slice, while copy copies elements from one slice to another.
slice = append(slice, 4, 5)
copy(destSlice, srcSlice)
35. How do you handle string concatenation in Go?
Strings can be concatenated using the + operator.
s := "Hello, " + "world!"
36. What is type assertion in Go?
var i interface{} = "hello" s := i.(string)
37. What is the go run command used for?
The go run command compiles and runs Go source files in one step.
go run main.go
38. How do you write a comment in Go?
Comments in Go are written using // for single-line comments and /* */ for multi-line comments.
// This is a single-line comment
/*
This is a multi-line comment
*/
39. What is the main function in Go?
func main() {
fmt.Println("Hello, World!")
}
40. What is a blank identifier _ in Go?
The blank identifier _ is used to ignore values in assignments and function returns.
_, err := someFunction()
41. How do you create constants in Go?
Constants are created using the const keyword.
const Pi = 3.14
42. What is the iota identifier in Go?
const (
A = iota
B
C
)
43. What is shadowing in Go?
Shadowing occurs when a variable declared within a certain scope (e.g., inside a function) has the same name as a variable declared in an outer scope, thus temporarily overriding the outer variable.
44. How do you convert between types in Go?
var x int = 42
var y float64 = float64(x)
45. What is the panic function in Go?
The panic function stops the normal execution of a program and begins panicking, which can be caught by a recover function to handle errors gracefully.
46. What is a rune in Go?
47. How do you check the length of a slice or string in Go?
length := len(slice)
48. How do you read and write files in Go?
The os and io/ioutil packages provide functions to read and write files.
data, err := ioutil.ReadFile("file.txt")
err = ioutil.WriteFile("file.txt", data, 0644)
49. What is the difference between make and new in Go?
make is used to create slices, maps, and channels, while new is used to allocate memory for a new variable and returns a pointer to it.
p := new(int)
m := make(map[string]int)
50. What is the import . syntax used for in Go?
import . "fmt"
func main() {
Println("Hello, World!")
}

Conclusion
Mastering Golang can open doors to exciting career opportunities in software development, particularly in fields requiring efficient and scalable solutions. By familiarizing yourself with these 50 beginner-level interview questions and answers, you can solidify your understanding of Go’s core concepts and be well-prepared for your next interview. Remember, the key to success lies in continuous practice and exploration of the language. Keep coding, keep learning, and you’ll soon find yourself proficient in one of the most robust programming languages available today. Also, sign up with Olibr to find top opportunities for Golang Developers.
Take control of your career and land your dream job
Sign up with us now and start applying for the best opportunities!
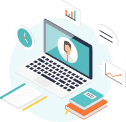
FAQs
Golang is widely used by developers in various industries, particularly those involved in backend development, cloud services, and infrastructure tools. Companies like Google, Uber, Dropbox, and Docker use Golang for its performance and reliability.
Some key features include:
- Concurrency: Golang has built-in support for concurrent programming.
- Simplicity: The syntax is clean and easy to learn.
- Performance: Compiled language with efficient execution.
- Garbage Collection: Automatic memory management.
- Standard Library: Extensive standard library for various needs.
Some recommended resources include:
- Official Go Documentation: Comprehensive and authoritative.
- Books: “The Go Programming Language” by Alan A. A. Donovan and Brian W. Kernighan.
- Online Courses: Platforms like Coursera, Udemy, and Pluralsight offer courses on Golang.
- Community: Join forums, Slack groups, or Reddit communities for support and networking.
Practicing for interviews can involve:
- Coding Challenges: Websites like LeetCode, HackerRank, and Exercism offer Golang challenges.
- Building Projects: Create small projects or contribute to open-source projects.
- Mock Interviews: Practice with peers or use platforms that offer mock interview services.
Projects that can highlight your skills include:
- Web Servers: Build a simple web server using net/http.
- CLI Tools: Develop command-line tools to automate tasks.
- APIs: Create RESTful or GraphQL APIs.
- Concurrent Programs: Implement programs that showcase Golang’s concurrency capabilities.
Yes, common pitfalls include:
- Ignoring Error Handling: Proper error handling is crucial in Golang.
- Misusing Goroutines: Understanding how to manage concurrency effectively is important.
- Overlooking Testing: Writing tests is an integral part of Go development.
- Neglecting Go Modules: Properly manage dependencies with Go modules.
While not crucial for beginners, having a basic understanding of the Go runtime and internals can be beneficial. As you advance, this knowledge will help you write more efficient and optimized code.
Golang is primarily used for backend development. However, it can be utilized in front-end development indirectly through WebAssembly or by generating static assets. Typically, front-end development is done using languages like JavaScript or frameworks like React.
Best practices include:
- Consistent Formatting: Use gofmt to format your code.
- Write Idiomatic Go: Follow Go conventions and idioms.
- Effective Error Handling: Handle errors gracefully and consistently.
- Documentation: Write clear and concise comments and documentation.
- Testing: Regularly write and run tests to ensure code quality.