Table of Contents
Toggle
Introduction
When it comes to front-end development, ReactJS stands out as a powerhouse. It’s like the Swiss Army knife for building user interfaces, making it a top choice for developers and companies alike. According to a 2024 Stack Overflow survey, ReactJS is used by over 40% of developers globally, showcasing its dominance in the web development world. As companies continue to seek skilled ReactJS developers, acing an interview can make all the difference. Here’s a collection of 100 essential ReactJS interview questions and answers to help you prepare like a pro. Whether you’re a seasoned developer or just starting, these questions cover a broad spectrum of topics to ensure you’re ready for anything that comes your way.
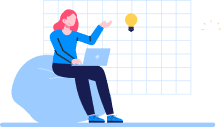
Don't miss out on your chance to work with the best
Apply for top global job opportunities today!

Top React.js Interview Questions and Answers
1. What is React, and why would you use it?
React is a JavaScript library developed by Facebook for building user interfaces, primarily for single-page applications. It helps create fast, scalable, and simple front-end applications by enabling developers to build reusable UI components. React follows a component-based architecture, which makes the development process modular and maintainable.
Read in detail: .NET Core vs .NET Framework
2. What are components in React?
Components are the building blocks of a React application. There are two types of components:
- Functional Components: These are JavaScript functions that accept props and return React elements.
- Class Components: These are ES6 classes that extend from React.Component and have lifecycle methods.
3. What is the Virtual DOM, and how does React use it?
The Virtual DOM (VDOM) is a lightweight representation of the real DOM. React creates an in-memory tree structure that mimics the DOM. When a component’s state changes, React updates the virtual DOM and compares it to the previous version using a process called “reconciliation.” React then updates only the parts of the real DOM that need changes, improving performance by minimizing direct DOM manipulations.
4. What is JSX?
JSX (JavaScript XML) is a syntax extension of JavaScript used in React to describe UI elements. It allows you to write HTML-like code inside JavaScript, which is later transpiled into regular JavaScript function calls. For example:
const element = <h1>Hello, world!</h1>;
This code will transpile to:
const element = React.createElement('h1', null, 'Hello, world!');
5. Explain the difference between state and props in React.
- State: It represents the internal data of a component that can change over time. The state is local to a component and can only be modified using setState() in class components or the useState() hook in functional components.
- Props: These are external inputs passed to components, which can be used to render dynamic content. Props are immutable and passed from parent to child components.
6. What are hooks in React?
Hooks are functions that allow functional components to use features like state and lifecycle methods. Common hooks include:
- useState: Manages state in a functional component.
- useEffect: Handles side effects like fetching data or subscribing to events.
- useContext: Provides access to the context API.
7. What is the purpose of useEffect hook?
useEffect is a hook in React that is used to handle side effects like data fetching, DOM manipulation, or subscribing/unsubscribing to services. It runs after the component renders and can also be configured to run only when specific values change.
Example
useEffect(() => {
// Effect logic here
}, [dependency]); // Only runs when dependency changes
8. How do you optimize performance in a React application?
Some common ways to optimize performance in React applications include:
- Memoization with React.memo(): Prevent unnecessary re-renders by memoizing components.
- Use useCallback and useMemo: To prevent unnecessary re-creation of functions and values in components.
- Lazy Loading Components: Using React.lazy() and Suspense for code-splitting and lazy loading components.
- Virtualization: Libraries like react-window can render only visible parts of a large list.
9. What is Context API, and when would you use it?
The Context API is a feature in React that allows you to share data across components without having to pass props through every level of the component tree. It’s useful for managing global data like user authentication, themes, and settings.
10. What is React.Fragment and why would you use it?
React.Fragment is a wrapper component used to group multiple children elements without adding extra nodes to the DOM. This is useful when you need to return multiple elements from a component without wrapping them in a div or another container element.
Example:
return (
<React.Fragment>
<h1>Title</h1>
<p>Description</p>
</React.Fragment>
);
Alternatively, you can use the shorthand syntax:
Example:
return (
<>
<h1>Title</h1>
<p>Description</p>
</>
);
11. What is the difference between controlled and uncontrolled components in React?
- Controlled Components: These components have their form data controlled by React via state. Any change to the input is handled by updating the state, and the input value is derived from the state.
- Uncontrolled Components: These components store their form data internally within the DOM and rely on refs to access the values.
Example of a controlled component:
const [value, setValue] = useState('');
return <input value={value} onChange={(e) => setValue(e.target.value)} />;
12. What is the difference between useEffect and useLayoutEffect?
- useEffect: Runs asynchronously after the render is committed to the screen. It does not block the painting of the UI.
- useLayoutEffect: Runs synchronously after all DOM mutations but before the browser has a chance to paint. It can block the painting of the UI, making it more suitable for synchronizing with layout changes.
13. How would you handle forms in React?
Forms in React are typically handled using state and event handlers. Each input field’s value is stored in state and updated via an onChange handler. React provides a declarative approach to handle form submissions, validation, and resetting.
Example:
const [formData, setFormData] = useState({ name: '', email: '' });
const handleSubmit = (e) => {
e.preventDefault();
// Process form data here
};
return (
<form onSubmit={handleSubmit}>
<input
type="text"
value={formData.name}
onChange={(e) => setFormData({ ...formData, name: e.target.value })}
/>
<input
type="email"
value={formData.email}
onChange={(e) => setFormData({ ...formData, email: e.target.value })}
/>
<button type="submit">Submit</button>
</form>
);
14. What are higher-order components (HOC) in React?
Higher-order components (HOCs) are functions that take a component and return a new component with enhanced behavior. HOCs are commonly used for reusing logic across multiple components, such as authentication, logging, or tracking.
Example:
function withLogger(WrappedComponent) {
return function EnhancedComponent(props) {
console.log('Component Rendered:', WrappedComponent.name);
return <WrappedComponent {...props} />;
};
}
15. What are React keys, and why are they important?
Keys are special attributes used in React to identify which items in a list have changed, been added, or removed. They help React optimize rendering by ensuring efficient updates to the DOM. Without keys, React will re-render the entire list, which can negatively affect performance.
Keys must be unique among sibling elements, commonly the id or a unique identifier in the dataset.
Example:
const items = ['apple', 'banana', 'orange'];
return (
<ul>
{items.map((item, index) => (
<li key={index}>{item}</li>
))}
</ul>
);
16. Explain the concept of lifting state up in React.
Lifting state up refers to moving shared state to the closest common ancestor of components that need to access it. This allows multiple child components to share and update the same state, avoiding inconsistent data across components.
For example, if two sibling components need to access the same piece of data, the state can be moved up to their parent component. The parent then passes the state and event handlers down via props.
17. What is Prop Drilling, and how can it be avoided?
Prop drilling occurs when data is passed from a parent component down through several layers of child components, even if intermediate components don’t need that data. This can make the component tree difficult to manage and understand.
To avoid prop drilling, you can use:
- Context API: It allows you to share data globally without having to pass props manually through every level.
- State management libraries like Redux or MobX: These provide a global store to manage and access data across the app.
18. What is the difference between React.PureComponent and React.Component?
- React.Component: Standard base class for creating components. It re-renders every time its state or props change, regardless of whether the new values are the same as the old ones.
- React.PureComponent: It is a version of React.Component that implements shallow comparison on props and state. It prevents unnecessary re-renders by re-rendering only when props or state have changed, providing a performance boost in cases where deep comparison is not needed.
Example of React.PureComponent:
class MyComponent extends React.PureComponent {
render() {
return <div>{this.props.name}</div>;
}
}
19. What are error boundaries in React?
Error boundaries are React components that catch JavaScript errors anywhere in the child component tree, log those errors, and display a fallback UI. Error boundaries are useful for preventing crashes of the whole app when certain parts of it encounter an error.
You can define an error boundary by using a class component and implementing the componentDidCatch lifecycle method or using getDerivedStateFromError.
Example:
class ErrorBoundary extends React.Component {
constructor(props) {
super(props);
this.state = { hasError: false };
}
static getDerivedStateFromError(error) {
return { hasError: true };
}
componentDidCatch(error, errorInfo) {
console.error(error, errorInfo);
}
render() {
if (this.state.hasError) {
return <h1>Something went wrong.</h1>;
}
return this.props.children;
}
}
20. What is the useReducer hook, and how is it different from useState?
The useReducer hook is an alternative to useState for managing complex state logic. It is used when the state logic involves multiple sub-values or depends on previous state values. It accepts a reducer function and an initial state, and returns the current state and a dispatch function.
- useState: Simpler and more common for managing individual pieces of state.
- useReducer: Better suited for complex state updates, especially when there are multiple state transitions or actions.
Example using useReducer:
const initialState = { count: 0 };
function reducer(state, action) {
switch (action.type) {
case 'increment':
return { count: state.count + 1 };
case 'decrement':
return { count: state.count - 1 };
default:
throw new Error();
}
}
function Counter() {
const [state, dispatch] = useReducer(reducer, initialState);
return (
<>
<p>Count: {state.count}</p>
<button onClick={() => dispatch({ type: 'increment' })}>+</button>
<button onClick={() => dispatch({ type: 'decrement' })}>-</button>
</>
);
}
21. What is React’s useRef hook, and when would you use it?
useRef is a hook in React that creates a mutable object that persists across re-renders. It’s commonly used to access or manipulate DOM elements directly or store values that do not trigger a re-render when changed.
Example of accessing a DOM element with useRef:
function FocusInput() {
const inputRef = useRef(null);
const handleFocus = () => {
inputRef.current.focus();
};
return (
<>
<input ref={inputRef} />
<button onClick={handleFocus}>Focus the input</button>
</>
);
}
22. What is code splitting in React, and how can you implement it?
Code splitting is a technique used to load parts of a React application on demand, rather than loading the entire application upfront. It helps improve performance by reducing the initial bundle size. React supports code splitting using dynamic import() and the React.lazy() function, along with Suspense for lazy loading components.
Example:
const LazyComponent = React.lazy(() => import('./LazyComponent'));
function MyComponent() {
return (
<Suspense fallback={<div>Loading...</div>}>
<LazyComponent />
</Suspense>
);
}
23. What are controlled and uncontrolled inputs in React?
- Controlled Inputs: The value of the input is controlled by React via state. Any change in the input updates the state, and the component re-renders with the new value.
- Uncontrolled Inputs: The input value is handled by the DOM itself. React uses refs to read the value from the DOM when necessary but does not manage the value in the component’s state.
Example of a controlled input:
const [value, setValue] = useState('');
return <input value={value} onChange={(e) => setValue(e.target.value)} />;
24. What is reconciliation in React?
Reconciliation is the process React uses to update the UI efficiently. When the state or props of a component change, React compares the new element tree with the previous one, determines what has changed, and updates only the necessary parts of the DOM. This process is powered by the Virtual DOM and the concept of diffing.
25. How would you implement a higher-order component (HOC) in React?
A higher-order component (HOC) is a function that takes a component and returns a new component with enhanced functionality. It’s used for code reuse, logic encapsulation, and conditional rendering.
Example:
function withLoading(Component) {
return function WithLoadingComponent({ isLoading, ...props }) {
if (isLoading) return <p>Loading...</p>;
return <Component {...props} />;
};
}
This HOC can now be used to wrap any component that requires a loading state.
26. How does React handle events differently from regular HTML?
React uses a synthetic event system to handle events in a consistent way across browsers. Instead of attaching event listeners directly to DOM elements, React wraps them in its own event system, normalizing the event object to ensure cross-browser compatibility.
Events in React are named using camelCase (e.g., onClick instead of onclick), and the event handlers receive a synthetic event object that has the same properties as a native event.
These additional questions dive deeper into React concepts and should help provide a solid foundation for interview preparation.
27. What are React Portals, and why would you use them?
React Portals allow you to render a component’s child into a different part of the DOM tree, outside the parent component. This is useful when dealing with modals, tooltips, or pop-ups that need to visually and structurally appear outside the normal DOM hierarchy but still belong to the parent component in terms of functionality.
Example:
import ReactDOM from 'react-dom';
function Modal({ children }) {
return ReactDOM.createPortal(
<div className="modal">{children}</div>,
document.getElementById('modal-root') // Rendered outside the usual DOM hierarchy
);
}
You’d use Portals when the component needs to break out of the layout restrictions of the parent but should still retain the parent’s logic, such as event handlers.
28. What is memoization in React, and how can you implement it?
Memoization in React is a technique used to optimize the performance of components by caching the result of expensive function calls and returning the cached result when the same inputs occur again. React provides the React.memo() higher-order component and the useMemo hook for memoization.
- React.memo(): It is used to memoize entire components, ensuring that they only re-render if their props change.
- useMemo(): It is used inside functional components to memoize values, preventing unnecessary recalculations during re-renders.
Example using React.memo:
const ExpensiveComponent = React.memo(function MyComponent({ data }) {
console.log('Rendered only when data changes');
return <div>{data}</div>;
});
29. What is the significance of React.StrictMode, and how does it help during development?
React.StrictMode is a wrapper component that helps developers identify potential problems in their code. It doesn’t render anything to the UI but helps catch side effects in the render phase by running certain lifecycle methods and hooks twice in development mode. It also highlights issues like:
- Deprecated lifecycle methods
- Unsafe side effects in useEffect
- Warnings for legacy string ref API
Usage:
<React.StrictMode>
<App />
</React.StrictMode>
React.StrictMode improves code robustness and prepares the application for future React updates.
30. What is the useCallback hook, and when should you use it?
useCallback is a React hook that memoizes a callback function, ensuring that the same function instance is returned on re-renders unless its dependencies change. This prevents unnecessary re-creations of functions that could trigger unnecessary re-renders of child components.
It’s especially useful when passing callback functions to optimized components that rely on reference equality, such as components wrapped in React.memo.
Example:
const handleClick = useCallback(() => {
console.log('Button clicked');
}, []); // The same function instance is retained unless the dependencies change
31. What are hooks rules, and why are they important?
React hooks must follow two specific rules to ensure they work correctly:
- Only Call Hooks at the Top Level: Hooks should only be called at the top level of a component or custom hook, not inside loops, conditions, or nested functions. This ensures that hooks are always called in the same order across re-renders.
- Only Call Hooks from React Functions: Hooks should be called from React functional components or custom hooks. They should not be called from regular JavaScript functions to prevent breaking React’s state management.
Following these rules ensures that React can properly manage component states and lifecycles, preventing bugs and unpredictable behavior.
32. What is the difference between useEffect and useLayoutEffect?
- useEffect: Runs after the DOM has been painted. It’s used for side effects like data fetching, subscriptions, or manually updating the DOM. It doesn’t block browser painting, making it non-blocking.
- useLayoutEffect: Fires synchronously after all DOM mutations and before the browser paints the UI. It’s used for operations that need to happen before the user sees updates (e.g., measuring DOM elements). This hook blocks the rendering process, so it’s best used sparingly and only when necessary.
Example:
useEffect(() => {
console.log('useEffect: Runs after painting');
});
useLayoutEffect(() => {
console.log('useLayoutEffect: Runs before painting');
});
33. What is the difference between controlled and uncontrolled components in React?
- Controlled Components: React controls the form inputs, meaning the form values are tied to the component’s state. Any change in the form value updates the state, and the state becomes the “single source of truth.”
Example:
const [value, setValue] = useState('');
return <input value={value} onChange={(e) => setValue(e.target.value)} />;
- Uncontrolled Components: The form input’s value is handled by the DOM itself. You use refs to access the input values.
Example:
const inputRef = useRef();
return <input ref={inputRef} />;
Uncontrolled components can be simpler in some cases but offer less control over the form data.
34. Explain the concept of reconciliation in React.
Reconciliation is the process React uses to efficiently update the DOM when the state of a component changes. React maintains a virtual DOM, which is a lightweight copy of the actual DOM. When state changes, React computes the differences between the current virtual DOM and the previous one (this process is called “diffing”). Based on the differences, React updates only the parts of the actual DOM that changed, minimizing unnecessary updates.
35. What is React's Context API, and when would you use it?
The Context API provides a way to pass data through the component tree without having to manually pass props at every level (avoiding “prop drilling”). It allows you to define a global value accessible to all components within a certain subtree.
You would use the Context API when multiple components at different levels need access to the same data, such as user authentication status, theme, or settings.
Example:
const ThemeContext = React.createContext('light');
function App() {
return (
<ThemeContext.Provider value="dark">
<Toolbar />
</ThemeContext.Provider>
);
}
function Toolbar() {
return <ThemeButton />;
}
function ThemeButton() {
const theme = React.useContext(ThemeContext);
return <button>{theme}</button>;
}
36. What is the purpose of the dangerouslySetInnerHTML attribute in React?
dangerouslySetInnerHTML is React’s replacement for directly using innerHTML in the DOM. It allows you to set HTML content from a string. However, it can be dangerous because it may expose your app to Cross-Site Scripting (XSS) attacks if the content isn’t properly sanitized.
Example:
<div dangerouslySetInnerHTML={{ __html: "<p>Some HTML content</p>" }} />
You should use this feature sparingly and ensure that the HTML content is safe and properly sanitized.
37. What is React's Suspense, and how is it used for lazy loading components?
Suspense is a component that allows you to defer rendering part of the component tree until some condition is met (typically data loading or lazy-loaded components). It’s primarily used for code-splitting with React.lazy but will also be useful for data fetching in future versions of React.
Example:
const LazyComponent = React.lazy(() => import('./LazyComponent'));
function App() {
return (
<React.Suspense fallback={<div>Loading...</div>}>
<LazyComponent />
</React.Suspense>
);
}
38. How do you handle side effects in functional components?
In functional components, side effects like data fetching, subscriptions, and manual DOM manipulation are handled using the useEffect hook. The hook allows you to run code after the component renders and clean up resources when the component unmounts.
Example:
useEffect(() => {
// Code to fetch data or subscribe to an event
return () => {
// Clean up subscriptions or resources
};
}, []); // Empty array means the effect runs only on mount and unmount
39. What is the difference between key and ref in React?
- key: A unique identifier used by React to efficiently update the DOM when rendering lists. It helps React differentiate between elements to avoid unnecessary re-renders. For example, when rendering an array of components, each item should have a unique key to maintain consistent ordering and optimize the update process.
Example:
{items.map(item => (
<Item key={item.id} data={item} />
))}
- ref: A reference to access the DOM elements or class component instances directly. You use ref to interact with DOM elements or trigger actions outside React’s declarative flow, such as focusing an input field programmatically.
Example:
const inputRef = useRef();
<input ref={inputRef} />;
40. What is the useImperativeHandle hook, and when should you use it?
useImperativeHandle is a React hook that allows you to customize the instance value that is exposed when using ref. It is typically used in conjunction with forwardRef to allow parent components to interact with child component instances in a controlled way.
You would use useImperativeHandle when you want to limit the functionality or customize the way a component is accessed through ref.
Example:
const MyInput = React.forwardRef((props, ref) => {
const inputRef = useRef();
useImperativeHandle(ref, () => ({
focus: () => {
inputRef.current.focus();
}
}));
return <input ref={inputRef} />;
});
41. What are React Fragments, and why are they useful?
React Fragments allow you to group a list of children components without adding an extra DOM node, such as a div. This is useful when you need to return multiple elements from a component without affecting the layout or adding unnecessary nesting in the DOM structure.
Example:
return (
<>
<Header />
<Content />
<Footer />
</>
);
Using Fragments keeps the DOM clean and free from unnecessary parent nodes.
42. Explain the difference between state and props in React.
- state: A component’s local data, which can change over time. It is managed internally by the component and is mutable. State changes trigger re-rendering of the component.
Example:
const [count, setCount] = useState(0);
- props: Short for “properties”, props are immutable data passed from a parent component to a child component. They are used to communicate between components.
Example:
<MyComponent name="John" />;
In summary, state is local and mutable, while props are external and immutable.
43. What are Higher-Order Components (HOCs) in React?
A Higher-Order Component (HOC) is a pattern where a function takes a component as an argument and returns a new component. HOCs are used to reuse component logic across different parts of the application. They are commonly used for tasks like enhancing components with additional props, state management, or lifecycle logic.
Example:
function withLogging(WrappedComponent) {
return function EnhancedComponent(props) {
console.log('Component is rendered');
return <WrappedComponent {...props} />;
};
}
Here, withLogging is an HOC that adds logging functionality to any wrapped component.
44. What are controlled forms, and why are they useful in React?
Controlled forms are forms in which the input values are controlled by React’s state. The form elements (such as input, textarea, etc.) are linked to state values and updated via event handlers. This provides more control over the form data, as the component fully manages the input’s value.
Example:
const [value, setValue] = useState('');
return (
<input
value={value}
onChange={(e) => setValue(e.target.value)}
/>
);
Controlled forms make validation, formatting, and submission handling easier because you have direct access to the form’s current data via the component’s state.
45. What is React’s ErrorBoundary, and how do you use it?
ErrorBoundary is a component used to catch JavaScript errors in the child component tree, log those errors, and display a fallback UI instead of crashing the entire app. It can only catch errors during rendering, lifecycle methods, and constructors of the child components.
Example:
class ErrorBoundary extends React.Component {
constructor(props) {
super(props);
this.state = { hasError: false };
}
static getDerivedStateFromError() {
return { hasError: true };
}
componentDidCatch(error, info) {
console.error('Error:', error, 'Info:', info);
}
render() {
if (this.state.hasError) {
return <h1>Something went wrong.</h1>;
}
return this.props.children;
}
}
ErrorBoundary helps prevent the entire app from crashing due to small, recoverable errors.
46. What is the useReducer hook, and how is it different from useState?
useReducer is a React hook that allows you to manage complex state logic. It’s similar to useState, but instead of returning the state and a function to update it, useReducer returns the current state and a dispatch function to trigger updates based on an action.
It’s useful when you have complex state logic that involves multiple sub-values or when the next state depends on the previous state.
Example:
const initialState = { count: 0 };
function reducer(state, action) {
switch (action.type) {
case 'increment':
return { count: state.count + 1 };
case 'decrement':
return { count: state.count - 1 };
default:
throw new Error();
}
}
const [state, dispatch] = useReducer(reducer, initialState);
In contrast to useState, useReducer is ideal when you need a more structured way to handle updates.
47. What are Synthetic Events in React?
Synthetic events are React’s wrapper around the browser’s native events. They work identically across all browsers to ensure consistent event behavior. React’s synthetic events pool and reuse event objects, improving performance by reducing memory overhead. However, because of this pooling, event properties may not persist if accessed asynchronously. You can use event.persist() to retain the event for future use.
Example:
function handleClick(event) {
event.persist();
console.log(event.type); // "click"
}
Using synthetic events makes event handling consistent across browsers and platforms.
48. What is the useTransition hook, and how does it improve user experience?
useTransition is a React hook that allows you to create non-blocking UI updates. It’s useful for distinguishing between urgent state updates (like typing) and non-urgent updates (like loading data). By marking updates as non-urgent, React can prioritize urgent updates and provide a smoother user experience.
Example:
const [isPending, startTransition] = useTransition();
function handleChange(e) {
startTransition(() => {
setSearchTerm(e.target.value);
});
}
useTransition makes the UI more responsive by ensuring that essential updates (like text input) are processed first, and heavier tasks (like re-rendering a list) are handled in the background.
49. Explain the difference between componentDidMount and useEffect.
- componentDidMount: A lifecycle method available in class components that runs once after the component has been rendered. It’s mainly used for tasks like fetching data or setting up subscriptions.
- useEffect: A hook used in functional components to mimic lifecycle behaviors like componentDidMount, componentDidUpdate, and componentWillUnmount. By providing an empty dependency array ([]), useEffect runs only once when the component mounts, similar to componentDidMount.
Example:
useEffect(() => {
// Effect code here (e.g., data fetching)
}, []); // Runs once, like componentDidMount
useEffect offers more flexibility as it works for both mounting and updating behavior, depending on its dependency array.
50. What is the purpose of useRef, and how is it different from useState?
useRef is a hook used to persist values between renders without causing a re-render when the value changes. It’s commonly used to directly reference DOM elements but can also be used to store mutable values.
- useRef: Updates to useRef values do not trigger a re-render.
- useState: Updates to useState trigger a re-render of the component.
Example:
const countRef = useRef(0);
countRef.current++;
useRef is useful when you need to track values across renders without impacting performance by triggering re-renders.
51. What is the difference between a function component and a class component in React?
- Function Component: A simple JavaScript function that returns JSX. It doesn’t have lifecycle methods or state without using hooks like useState and useEffect. It is lightweight and easier to read.
Example:
const MyComponent = () => {
return <div>Hello, World!</div>;
};
- Class Component: A class-based component that extends React.Component. It has access to lifecycle methods (componentDidMount, componentDidUpdate) and can manage its own state using this.state and this.setState().
Example:
class MyComponent extends React.Component {
render() {
return <div>Hello, World!</div>;
}
}
52. What are React Hooks, and why were they introduced?
React Hooks were introduced in React 16.8 to allow function components to manage state and other React features without the need for class components. Hooks simplify the structure and logic of components by removing the need for lifecycle methods and providing a more functional approach to managing state and side effects.
Common hooks include:
- useState: For managing state.
- useEffect: For handling side effects like data fetching.
- useContext: For consuming context without needing to wrap components in context consumers.
Hooks were introduced to make code simpler, improve reusability of logic, and eliminate the need for complex class-based component structures.
53. What is the purpose of the React.memo() function?
React.memo() is a higher-order component used to optimize performance by preventing unnecessary re-renders. When a component is wrapped in React.memo(), React will only re-render it if its props change. This is useful for functional components that render frequently with the same props.
Example:
const MyComponent = React.memo((props) => {
return <div>{props.value}</div>;
});
In this case, MyComponent will only re-render if props.value changes, thus improving performance.
Also Read: What is .NET? What is .Net used for?

Conclusion
Preparing for a React JS interview can seem daunting given the vast array of concepts and techniques the library encompasses. However, by systematically understanding and mastering the core principles—such as component architecture, state management, hooks, and performance optimization—you can confidently demonstrate your proficiency and problem-solving abilities to potential employers.
This compilation of React JS interview questions and answers is designed to provide a comprehensive overview of both fundamental and advanced topics. From understanding the nuances between state and props to implementing efficient performance strategies with hooks like useMemo and useCallback, each question targets key areas that are frequently explored in technical interviews.
Key Takeaways:
- Deep Understanding of Core Concepts: Ensure you have a solid grasp of React’s core principles, including the virtual DOM, component lifecycle, and JSX syntax. These foundational elements are essential for building efficient and scalable applications.
- Proficiency with Hooks: Modern React development heavily relies on Hooks. Familiarize yourself with useState, useEffect, useContext, and more specialized hooks like useReducer and useTransition to manage state and side effects effectively.
- Performance Optimization: Demonstrating knowledge of performance enhancement techniques, such as memoization with React.memo and optimizing re-renders, can set you apart as a candidate who values efficient code.
- Advanced Patterns: Understanding advanced patterns like Higher-Order Components (HOCs), Render Props, and Context API showcases your ability to write reusable and maintainable code.
- Error Handling and Best Practices: Implementing error boundaries and adhering to best practices ensures your applications are robust and user-friendly, even in unexpected scenarios.
- Practical Application: Beyond theoretical knowledge, being able to apply concepts through coding examples and real-world scenarios is crucial. Practice building projects and solving problems to reinforce your understanding.
Final Advice:
- Stay Updated: React is continually evolving, with new features and best practices emerging regularly. Keep abreast of the latest updates and incorporate them into your skill set.
- Hands-On Practice: Engage in building projects or contributing to open-source to apply what you’ve learned. Practical experience is invaluable and often highlights your capabilities better than theoretical knowledge alone.
- Mock Interviews: Conduct mock interviews with peers or use online platforms to simulate the interview environment. This practice can help reduce anxiety and improve your ability to articulate your thoughts clearly.
- Understand the Why: Beyond knowing how to use React features, strive to understand why certain patterns or practices are recommended. This deeper comprehension will enable you to make informed decisions and adapt to various challenges during development.
Take control of your career and land your dream job
Sign up with us now and start applying for the best opportunities!
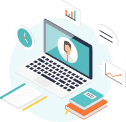