Table of Contents
Toggle
Introduction
As an intermediate Python programmer, you are entering a phase that demands a deeper understanding of the programming language. Intermediate Python interviews evaluate your ability to apply the language to real-world scenarios. In this blog, we have compiled the top Python intermediate interview questions to help you ace your 2024 Python interviews. So, let’s dive in!

Top Python Intermediate Interview Questions
1. What do you mean by Python literals?
Literals are notations that represent a fixed value in the source code. Literals in Python refer to the raw data we assign to variables or constants while programming.
There are five types of literals:
- String Literals
- Numeric Literals
- Boolean Literals
- Literal Collections
- Special Literals
2. What is the map function in Python?
Map in Python functions as an iterator and helps return a result after applying a function to every item of an iterable (tuple, lists, etc.). Python developers use it when they want to employ a single transformation function to all the iterable elements. The iterable and function are passed as arguments to the map in Python.
3. Explain generators in Python.
Generators in Python are a way to create iterators using a function. They allow you to iterate over a potentially large set of data without loading the entire set into memory at once. Generators use the yield keyword to produce a series of values over multiple calls to the generator function.
4. What do you understand by iterators in Python?
Iterators are objects that implement the iterator protocol. They consist of the __iter__() method (returns the iterator object) and the __next__() method (returns the next value from the iterator). Iterators are used to iterate through a sequence of elements, and many built-in Python objects like lists, tuples, and dictionaries are iterable.
5. Is it necessary to declare variables with respective data types in Python?
No. Since Python is a dynamically typed language, you don’t need to explicitly declare the data type of a variable. The data type of a variable is automatically identified by the Python Interpreter based on the type of value assigned.
6. What is Dict and List Comprehension?
Python comprehensions help create lists and dictionaries in Python. Comprehension helps dictionaries, sets from a given list, dictionary, or a set, or build altered and filtered lists. You can also create concise expressions using Comprehensions. With Comprehensions, there is no need for explicit loops. This can save time and help reduce code size during development.
Comprehensions are beneficial when:
- Performing mathematical operations on the entire list
- Combining multiple lists into one
- Flattening a multi-dimensional list
- Performing conditional filtering operations on the entire list
7. How to write comments in Python?
Comments in Python start with the # symbol. Single-line comments can be written after #, and multiline comments are often created using triple-quotes (‘‘ or “””).
8. Is multiple inheritance supported in Python?
Python supports multiple inheritance, that is, a class can inherit attributes and methods from more than one parent class. A scenario where a class is instantiated from more than one individual parent class is a multiple inheritance. This adds functionality and advantages.
9. What are Python modules?
In Python, range and xrange are functions used to iterate inside a loop for a fixed number of times. Although function wise they are the same, there are differences regarding the Python version support for these functions and their return values.
Xrange() is not supported in Python 3, but to iterate inside for loops, the range() function is used. However, the xrange() function is used in Python 2 to iterate inside for loops.
As the range() method keeps the entire list of iterating numbers in memory, it consumes more memory. The xrange() method keeps only one number at a time in memory, and hence, takes less memory.
10. What do you understand by the word Tkinter?
Tkinter is a standard built-in toolkit for GUI development in Python that we can start using by importing it into the script.
11. Is Python fully object oriented?
Yes, Python is a fully object-oriented programming (OOP) language. Everything in Python is an object, and the language supports basic OOP concepts such as inheritance, polymorphism, and more, except access specifiers. Python doesn’t support strong encapsulation. Although, it has a convention that can be used for data hiding.
12. Give any two differences between NumPy and SciPy.
- NumPy, or Numerical Python, is used for numeric computations on numerical data saved in arrays. SciPy, or Scientific Python, is used to perform operations such as integration, differentiation, and more.
- NumPy has some linear algebraic functions, but they are not full-fledged. SciPy has full-fledged algebraic functions for algebraic computations.
13. What are the file processing modes supported in Python?
The common file processing modes include:
Three modes for opening files:
- read-only mode (r)
- write-only mode (w)
- read–write mode (rw)
To open a file using the above modes, append ‘t’ with them as follows:
- read-only mode (rt)
- write-only mode (wt)
- read–write mode (rwt)
Open a binary file appending ‘b’ with the modes as follows:
- read-only mode (rb)
- write-only mode (wb)
- read–write mode (rwb)
Use the append mode (a) to append the content in the files:
- ‘at’ for text files
- ‘ab’ for binary files
14. What do file-related modules in Python do? Name some file-related modules in Python.
File-related modules in Python can manipulate text files and binary files in a file system. These modules can create text or binary files and update content by carrying out operations like copy, delete, and more. Some examples are:
- os: Operating system interface
- shutil: High-level file operations
- glob: Pattern matching on file names
- os.path: Common pathname manipulations
15. What are the benefits of NumPy arrays compared to Python lists?
NumPy arrays are more efficient for numerical operations than nested lists in the following ways:
- NumPy is easy and convenient for programmers as it has a highly readable syntax.
- NumPy arrays make coding more efficient as they consume a lot less memory.
- NumPy arrays do not add heavy processing to the runtime as they execute faster.
16. Explain encapsulation in Python.
When you wrap up the variables and different functions into a single entity or capsule, it is called encapsulation in Python. An example of encapsulation is the Python class.
17. What is polymorphism in Python?
In the context of Python, the code’s ability to take multiple forms is called polymorphism. For example, if the parent class has a method named XYZ, then the child class can also have a method with the same name with its own variables and parameters.
18. Is it possible to call parent class without its instance creation?
If the base class is instantiated by other child classes or if the base class is a static method, it is possible.
19. How can parent members be accessed inside a child class?
You can use the name of the parent class to access attributes inside a child class. Call the constructor of parent class inside the constructor of child class. Following this step, the object of a child class can access the methods and attributes of the parent class.
20. How can you check if a class is a child of another class?
We can use the issubclass() method in Python to do this. It tells you if any class is a child of another class by returning true or false accordingly. Additionally, we can use the instance() to check if an object is an instance of a class.
21. What are unit tests in Python?
In Python, unit testing is an important function used to test individual units or components of code to ensure their proper function. The two main frameworks that make unit testing easier are unittest and PyTest.
22. How can one convert a string to all lowercase?
We need to use the lower() function to convert a string to lowercase. It is a built-in string method that returns a new string with all characters converted to lowercase.
23. How to generate random numbers in Python?
You need to import the random module to do this. For example, random.randint(a, b) generates a random integer between a and b.
24. How to remove the last object from a list in Python?
Use the pop() method to remove the last object from a list:
my_list = [1, 2, 3, 4]
my_list.pop()
25. What is the method to reverse a list in Python?
We can use the reverse() method or the [::-1] slicing technique to reverse a list in Python:
original_list = [1, 2, 3, 4, 5]
reversed_list = original_list[::-1]
26. Write a code to count the number of capital letters in a file.
count = 0
text = countletter.read()
for character in text:
if character.isupper():
count += 1
You can also use the generator expansion feature to convert the whole code block into a one-line code:
count = sum(1 for line in countletter for character in line if character.isupper())
27. Write a code to sort a numerical list in Python.
numbers = ["2", "5", "7", "8", "1"]
numbers = [int(i) for i in numbers]
numbers.sort()
print(numbers)
28. How can one delete values from a Python array?
- The del statement deletes one or more items from an array.
- The remove() method removes the given item.
- The pop() method removes an item at the given index.
29. What is the process for appending values to a Python array?
The extend(), append(), and insert() functions help to add elements in an array. Here is an example:
x=arr.array('d', [11.1 , 2.1 ,3.1] )
x.append(10.1)
print(x) #[11.1,2.1,3.1,10.1]
x.extend([8.3,1.3,5.3])
print(x) #[11.1,2.1,3.1,10.1,8.3,1.3,5.3]
x.insert(2,6.2)
print(x) # [11.1,2.1,6.2,3.1,10.1,8.3,1.3,5.3]
30. What is the use of ternary operators in Python?
Conditional statements in Python can be shown using the ternary operator.
Syntax:
x , y=10,20
count = x if x<y else y
31. Why doesn’t Python deallocate all memory upon exit?
This is because of circular references. When Python exists, for the modules with circular references to other objects, or the objects that are referenced from the global namespaces, the memory is not always deallocated or freed. Also, the bits that are allocated by the C library are not possible to free. Python tries to deallocate every object on exit as it has its own cleanup mechanism.
32. What is the difference between /, %, and //?
- / is the division operator (results in a float).
- % is the modulus operator (remainder of the division).
- // is the floor division operator (results in an integer, discards the fractional part).
33. What is the purpose of “in,” “is,” and “not” operators?
Special functions that produce a corresponding result by taking one or more values (operands) are called operators.
- The “in” operator tells if the element is present in a given Sequence or not.
- The “is” operator returns the true value when both the operands are true.
- The “not” operator returns the inverse of the boolean value based on the operands.
34. What would be the output for the following code block?
list1 = [2, 33, 222, 14, 25]
print(list1[-2])
The output would be 14. In Python, you can access elements from the end of the list using negative indexing. The index -1 represents the last element, -2 represents the second-to-last element, and so on. In the given code, list1[-2] refers to the second-to-last element in the list list1, which is 14.
35. How do you create an empty class in Python?
A class with no code defined within its block is called an empty class. It can be generated using the pass keyword. The empty class serves as a placeholder until you define the properties and methods.
36. Write a Python program to count the total number of lines in a text file.
def file_count(fname):
with open(fname) as f:
for i, _ in enumerate(f):
pass
return i + 1
print("Total number of lines in the text file:",
file_count("file.txt"))
37. How will you read a random line in a file?
The random module can be used to do this. Here is an example:
import random
def read_random(fname):
lines = open(fname).read().splitlines()
return random.choice(lines)
print(read_random('hello.txt'))
38. How are access specifiers used in Python?
It can be done using naming conventions and underscores (_ and __) before variable or method names.
39. How to delete files in Python?
The os.remove() function can be used to delete files in Python. You need to import the OS Module for this. Here is a code that can be used:
import os
os.remove("file_name.txt")
40. Can we remove duplicate elements from a list? How?
The set() function is used to remove duplicate elements from the list. For example:
demo_list = [5, 4, 4, 6, 8, 12, 12, 1, 5]
unique_list = list(set(demo_list))
output = [1, 5, 6, 8, 12]
41. How can the items of a list in place be randomized in Python?
You can randomize the items of a list in place using the random.shuffle() function:
import random
my_list = [1, 2, 3, 4, 5]
random.shuffle(my_list)
42. What do *args and **kwargs mean in Python?
Arguments that are passed to the function are “args,” whereas keyword arguments that are passed along with the values into the function are “kwargs.”
43. What does len() do?
It is an inbuilt function that helps calculate the length of sequences like list, Python string, and array.
my_list = [1, 2, 3, 4, 5]
length = len(my_list)
print(length)
44. Write a command to open the file c:\hello.txt for writing.
f= open(“hello.txt”, “wt”)
45. Which one of the following statements is invalid? Why?
- xyz = 1,000,000
- x y z = 1000 2000 3000
- x,y,z = 1000, 2000, 3000
- x_y_z = 1,000,000
The second option is invalid. Variable names in Python cannot contain spaces, and multiple variables cannot be assigned in this way without commas to separate them. Also, the statement is syntactically incorrect as the values to be assigned are not separated by commas.
46. Write a code to display the contents of a file in reverse.
filename = "filename.txt"
with open(filename, "r") as file:
lines = file.readlines()
for line in reversed(lines):
print(line.rstrip())
47. What is the use of the ‘with’ statement in Python? What is its syntax?
The ‘with’ statement is used in Python to open a file and close it as soon as the block of code, where ‘with’ is used, exits. This eliminates the need to use the close() method.
Syntax:
with open("filename", "mode") as file_var:
48. How is the ‘super’ function used in Python?
We can use super() to access the super keyword in the child class.
class Parent(object):
# Constructor
def __init__(self, name):
self.name = name
class Child(Parent):
# Constructor
def __init__(self, name, age):
'''
In Python 3.x, we can also use super().__init__(name)
'''
super(Child, self).__init__(name)
self.age = age
def display(self):
# Note that Parent.name can’t be used
# here since super() is used in the constructor
print(self.name, self.age)
# Driver Code
obj = Child("ChildClassInstance", 9)
obj.display()
49. Give an example of how a series can be created from the dictionary object in pandas.
import pandas as pd
dict_info = {'key1' : 2.0, 'key2' : 3.1, 'key3' : 2.2}
series_obj = pd.Series(dict_info)
print (series_obj)
Output:
x 2.0
y 3.1
z 2.2
dtype: float64
50. How will you identify and deal with missing values in a data frame?
We can use the ‘IsNull()’ and ‘isna()’ methods to identify if a data frame has missing values. Here is a code for doing this:
Missing_data_count = df.isnull().sum()
51. Write a code to add a new column to Pandas data frame.
import pandas as pd
data_info = {'first' : pd.Series([1, 2, 3], index = ['a', 'b', 'c']),
'second' : pd.Series([1, 2, 3, 4], index = ['a', 'b', 'c', 'd'])}
df = pd.DataFrame(data_info)
# To add new column third
df['third']=pd.Series([10,20,30], index= ['a','b','c'])
print(df)
# To add new column fourth
df['fourth'] = df['first'] + info['third']
print(df)
52. Write a code to delete indices, rows and columns from a data frame.
The del df.index.name should be executed to remove the index by name. You can also use the df.index to assign a name to None.
For example, consider this data frame:
Column 1
Names
Max 1
Will 2
Ellen 3
Jin 4
Here is how you can drop the index name “Names”:
df.index.name = None
# Or run the below:
# del df.index.name
print(df)
Column 1
Max 1
Will 2
Ellen 3
Jin 4
To delete a row/column from a data frame:
- Use the drop() method to delete row/column from a data frame.
- The axis argument is passed in order to the drop method if the value is 0, it signals to drop/delete a row and a column if it’s 1.
- By setting the value of ‘inplace’ to True, we can try to delete rows/columns in-place. This ensures that it is deleted without the need for reassignment.
- The drop_duplicates() method can delete the duplicate values from the row/column.
53. Show how the first row can be reindexed as the name of the columns in Pandas.
new_header = df.iloc[0] # grab the first row for the header
df = df[1:] # take the data less the header row
df.columns = new_header # set the header row as the df header
54. What is reindexing in Pandas?
Reindexing refers to changing the row and column labels of a data frame to match a new set of labels. It can be used to rearrange, add, or remove rows and columns in a data frame, aligning the data with a specified set of labels.
55. What is an Armstrong number in Python?
Armstrong number in Python is a mathematical property used in coding and math puzzles. It is a number that equals the sum of its individual digits, each raised to the power of the number of digits. For example, 153 is an Armstrong number because 1^3 + 5^3 + 3^3 equals 153.
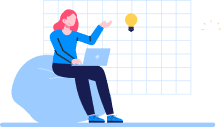
Don't miss out on your chance to work with the best!
Apply for top job opportunities today!

Conclusion
Intermediate knowledge of Python mainly tests your problem-solving skills and your ability to write clean, readable, and well-structured code, among other things. In this blog, we have included questions to help you evaluate your interview prep for these skills, among other things. Remember that these questions are meant to assess your current knowledge, but more importantly, inspire continuous learning. Best of luck with your Python interviews! If you are a Python developer looking for interesting job opportunities, then sign up with Olibr now!
FAQs
Here are a few intermediate Python programming jobs:
- Senior Python Engineer – Data Platform
- Senior Full Stack Developer
- Information System Security Professional
- Data Warehouse Engineer
- AWS Cloud Engineer
Python has a relatively straightforward syntax and is one of the easiest programming languages to learn.
Based on how you want to use Python in your career, you can gain proficiency in HTML, CSS, JavaScript, Java, or the C languages (C, C++, C#).
Both are popular programming languages. Although Java is faster, Python is simpler and easier to learn. Both are well-established, platform-independent, and has a large, supportive community.
Take control of your career and land your dream job!
Sign up and start applying to the best opportunities!
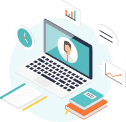