Table of Contents
Toggle
Introduction
Advanced Go (Golang) interviews typically cover more than just basic syntax and simple programming tasks. Candidates should show a deep understanding of Go’s runtime, how memory is managed, advanced concurrency patterns, ways to optimize performance, and clever use of the standard library. Interviewers might ask about specific features of the language, best practices for writing efficient and easy-to-maintain code, and strategies for solving real-world problems. Here are some advanced Go interview questions and answers that go beyond beginner and intermediate topics.
Read in detail: WHAT IS GOLANG? WHAT IS IT USED FOR?
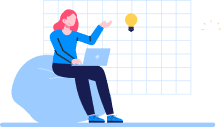
Don't miss out on your chance to work with the best
Apply for top global job opportunities today!

Top Advanced Golang Interview Questions and Answers
1. What is the purpose of sync.Pool in Go? How does it work?
sync.Pool is used to create a pool of reusable objects that can help reduce the overhead of allocating and deallocating memory frequently. It’s particularly useful in high-performance applications where garbage collection can become a bottleneck.
How it works:
- sync.Pool provides a pool of temporary objects which can be reused. When an object is needed, Get is called, which retrieves an object from the pool if one is available or creates a new one if the pool is empty.
- When an object is no longer needed, it is put back into the pool using Put, making it available for future use.
package main
import (
"fmt"
"sync"
)
func main() {
var pool = sync.Pool{
New: func() interface{} {
return new(int)
},
}
v := pool.Get().(*int)
*v = 42
fmt.Println(*v)
pool.Put(v)
fmt.Println(pool.Get().(*int))
}
2. Explain how the Go scheduler works. What are Goroutines and how does Go manage them?
The Go scheduler is a part of the Go runtime that manages the execution of goroutines. Goroutines are lightweight threads managed by the Go runtime.
- Goroutines: They are functions or methods that run concurrently with other functions or methods. Goroutines are much cheaper than traditional threads in terms of memory and scheduling overhead.
- Scheduler: Go uses a cooperative scheduler that runs on a work-stealing algorithm. It divides the execution into logical processors (GOMAXPROCS) and schedules goroutines on these processors.
The Go runtime maps M (machine, or OS threads) to P (logical processors) and each P manages a set of G (goroutines).
3. What is the memory model in Go? Explain how memory is managed.
The memory model in Go defines how the program sees memory and how operations on memory are performed. Go uses a garbage-collected heap and a stack for memory management.
- Stack: Each goroutine has its own stack, which is small at creation (a few KB) and grows or shrinks dynamically.
- Heap: Managed by a garbage collector which identifies and frees memory that is no longer in use.
The Go memory model guarantees that if one goroutine writes to a variable and another reads from it, there must be synchronization (via channels, sync primitives like Mutex, etc.) to ensure visibility of the write.
4. How does Go handle panic and recover? Can you provide an example?
Panic is used to handle unexpected errors in Go. When a function encounters a situation it cannot handle, it calls panic. This causes the function to stop executing and start unwinding the stack, executing deferred functions along the way. recover is used to regain control of a panicking goroutine.
Example
package main
import "fmt"
func main() {
defer func() {
if r := recover(); r != nil {
fmt.Println("Recovered from", r)
}
}()
fmt.Println("Calling function that panics")
mayPanic()
fmt.Println("Returned normally from mayPanic")
}
func mayPanic() {
panic("Something went wrong!")
}
5. What are the different ways to optimize Go code? Provide some techniques.
Several techniques can be used to optimize Go code, including:
- Profiling: Use pprof to profile CPU and memory usage.
- Avoiding unnecessary allocations: Minimize allocations by reusing objects and slices.
- Using sync.Pool: For managing temporary objects.
- Efficient concurrency: Properly use goroutines and channels.
- Inlining: Write small functions that can be inlined by the compiler.
- Reducing garbage collection overhead: By reducing the allocation rate.
- Optimizing I/O operations: Use buffered I/O and minimize system calls.
- Avoiding reflection: Since it can be slower and less safe.
6. How does Go's type embedding work? Can you provide an example?
Type embedding in Go allows one type to include another type, gaining its methods and fields. It’s similar to inheritance but uses composition.
Example:
package main
import "fmt"
type Person struct {
Name string
Age int
}
func (p Person) Greet() {
fmt.Println("Hello, my name is", p.Name)
}
type Employee struct {
Person // Embedding Person
Position string
}
func main() {
e := Employee{
Person: Person{Name: "Alice", Age: 30},
Position: "Engineer",
}
e.Greet() // Accessing the embedded Person's method
fmt.Println(e.Position)
}
7. Explain Go's garbage collector and how it works.
Go uses a concurrent, tri-color mark-and-sweep garbage collector, which is designed to minimize pause times and work concurrently with the application.
- Tri-color marking: It partitions objects into three sets (white, grey, and black) to track which objects are reachable and which are not.
- Mark phase: It marks all reachable objects.
- Sweep phase: It reclaims the memory occupied by unreachable objects.
- Concurrent: Runs alongside the program, reducing pause times.
8. What is a select statement in Go? How does it work?
The select statement lets a goroutine wait on multiple communication operations (channel operations). It blocks until one of its cases can proceed.
Example:
package main
import (
"fmt"
"time"
)
func main() {
ch1 := make(chan string)
ch2 := make(chan string)
go func() {
time.Sleep(1 * time.Second)
ch1 <- "message from ch1"
}()
go func() {
time.Sleep(2 * time.Second)
ch2 <- "message from ch2"
}()
select {
case msg1 := <-ch1:
fmt.Println(msg1)
case msg2 := <-ch2:
fmt.Println(msg2)
case <-time.After(3 * time.Second):
fmt.Println("timeout")
}
}
9. What is the purpose of the unsafe package in Go? Provide examples of its usage.
The unsafe package provides facilities for low-level programming that can break the type safety of Go programs. It’s used to perform operations that are not possible through standard Go constructs, such as pointer arithmetic and direct memory access.
Examples:
- Converting a pointer to a uintptr and back:
package main
import (
"fmt"
"unsafe"
)
func main() {
var x int = 42
p := &x
fmt.Println(*p) // 42
up := uintptr(unsafe.Pointer(p))
p2 := (*int)(unsafe.Pointer(up))
fmt.Println(*p2) // 42
}
- Accessing struct fields through unsafe:
package main
import (
"fmt"
"unsafe"
)
type MyStruct struct {
a int64
b int32
}
func main() {
s := MyStruct{a: 42, b: 21}
bPtr := (*int32)(unsafe.Pointer(uintptr(unsafe.Pointer(&s)) + unsafe.Offsetof(s.b)))
fmt.Println(*bPtr) // 21
}
10. Explain the difference between sync.Mutex and sync.RWMutex.
Both sync.Mutex and sync.RWMutex are synchronization primitives used to protect shared resources from concurrent access, but they have different use cases.
- sync.Mutex: A mutual exclusion lock that allows only one goroutine to access a critical section at a time.
- Methods: Lock() and Unlock()
- Suitable for cases where either read or write operations need to be synchronized.
- sync.RWMutex: A read-write mutex that allows multiple readers or a single writer to access the critical section.
- Methods: RLock(), RUnlock(), Lock(), and Unlock()
- Suitable for cases where read operations are more frequent than write operations.
Example:
package main
import (
"fmt"
"sync"
)
func main() {
var mu sync.Mutex
var rwmu sync.RWMutex
// Using sync.Mutex
mu.Lock()
fmt.Println("Locked with Mutex")
mu.Unlock()
fmt.Println("Unlocked Mutex")
// Using sync.RWMutex
rwmu.RLock()
fmt.Println("Read locked with RWMutex")
rwmu.RUnlock()
fmt.Println("Read unlocked RWMutex")
rwmu.Lock()
fmt.Println("Write locked with RWMutex")
rwmu.Unlock()
fmt.Println("Write unlocked RWMutex")
}
11. How can you handle large JSON datasets efficiently in Go?
Handling large JSON datasets efficiently can be achieved using streaming techniques provided by the encoding/json package in Go. Instead of unmarshaling the entire JSON into memory, you can use the Decoder to read and process the JSON incrementally.
Example:
package main
import (
"encoding/json"
"fmt"
"os"
)
func main() {
file, err := os.Open("large.json")
if err != nil {
panic(err)
}
defer file.Close()
decoder := json.NewDecoder(file)
for {
var obj map[string]interface{}
if err := decoder.Decode(&obj); err != nil {
if err == os.ErrClosed {
break
}
panic(err)
}
fmt.Println(obj)
}
}
12. What are Go's build constraints and how are they used?
Build constraints (also known as build tags) are used to include or exclude files from the build based on the conditions specified in the comments at the top of the file.
Example:
- File for Linux-only:
// +build linux
package main
import "fmt"
func main() {
fmt.Println("This code runs only on Linux")
}
- File for multiple conditions:
// +build darwin amd64
package main
import "fmt"
func main() {
fmt.Println("This code runs on macOS and AMD64 architecture")
}
13. How does Go's defer statement work, especially in the context of function returns and error handling?
The defer statement in Go schedules a function call to be run after the function completes, but before the actual return. Deferred functions are executed in Last-In-First-Out (LIFO) order.
Example:
- Defer with function return:
package main
import "fmt"
func main() {
fmt.Println(f()) // Outputs: 2
}
func f() (result int) {
defer func() {
result++
}()
return 1
}
- Defer with error handling:
package main
import (
"fmt"
"os"
)
func main() {
f, err := os.Open("file.txt")
if err != nil {
fmt.Println(err)
return
}
defer f.Close()
// Use f
}
14. What are context packages in Go, and how do they improve the handling of timeouts and cancellations?
The context package in Go provides a way to manage deadlines, cancellations, and other request-scoped values across API boundaries and between processes. It helps to propagate cancellation signals and deadlines across goroutines.
Example:
package main
import (
"context"
"fmt"
"time"
)
func main() {
ctx, cancel := context.WithTimeout(context.Background(), 2*time.Second)
defer cancel()
go func() {
select {
case <-time.After(1 * time.Second):
fmt.Println("Completed work")
case <-ctx.Done():
fmt.Println("Cancelled:", ctx.Err())
}
}()
time.Sleep(3 * time.Second)
}
15. Explain the concept of interfaces in Go. How do you implement and use them effectively?
Interfaces in Go define a set of method signatures but do not implement them. They provide a way to specify the behavior that types must implement, enabling polymorphism.
Example:
- Defining and using interfaces:
package main
import "fmt"
type Speaker interface {
Speak() string
}
type Dog struct{}
func (d Dog) Speak() string {
return "Woof!"
}
type Cat struct{}
func (c Cat) Speak() string {
return "Meow!"
}
func main() {
var s Speaker
s = Dog{}
fmt.Println(s.Speak()) // Woof!
s = Cat{}
fmt.Println(s.Speak()) // Meow!
}
Empty interface: The empty interface interface{} can hold values of any type because every type implements at least zero methods.
package main
import "fmt"
func main() {
var x interface{}
x = 42
fmt.Println(x) // 42
x = "hello"
fmt.Println(x) // hello
}
16. What is the reflect package, and how can it be used in Go?
The reflect package in Go provides a way to inspect the type and value of variables at runtime, allowing for dynamic type operations.
Example:
- Inspecting type and value:
package main
import (
"fmt"
"reflect"
)
func main() {
var x float64 = 3.4
fmt.Println("type:", reflect.TypeOf(x))
fmt.Println("value:", reflect.ValueOf(x))
}
- Modifying a value via reflection:
package main
import (
"fmt"
"reflect"
)
func main() {
var x float64 = 3.4
v := reflect.ValueOf(&x).Elem()
v.SetFloat(7.1)
fmt.Println(x) // 7.1
}
17. What is an interface type assertion in Go, and how does it work?
Type assertion in Go is used to extract the underlying value of an interface variable or to test whether the interface value holds a specific type.
- Single return: Asserts that the interface holds the specified type. It panics if the assertion is not true.
var i interface{} = "hello"
s := i.(string)
fmt.Println(s) // hello
- Comma, ok idiom: Safely tests if the interface holds the specified type and returns a boolean indicating success or failure.
var i interface{} = "hello"
s, ok := i.(string)
if ok {
fmt.Println(s) // hello
} else {
fmt.Println("Type assertion failed")
}
18. How can you create and use custom errors in Go?
Creating and using custom errors in Go can be done by defining types that implement the error interface.
Example:
Custom error type:
package main
import (
"errors"
"fmt"
)
type MyError struct {
Msg string
Code int
}
func (e *MyError) Error() string {
return fmt.Sprintf("%s (code: %d)", e.Msg, e.Code)
}
func doSomething() error {
return &MyError{Msg: "Something went wrong", Code: 123}
}
func main() {
err := doSomething()
if err != nil {
fmt.Println(err)
}
// Type assertion to access custom fields
if myErr, ok := err.(*MyError); ok {
fmt.Println("Error code:", myErr.Code)
}
}
Using errors package for wrapping and unwrapping:
package main
import (
"errors"
"fmt"
)
var ErrNotFound = errors.New("not found")
func doSomething() error {
return fmt.Errorf("an error occurred: %w", ErrNotFound)
}
func main() {
err := doSomething()
if errors.Is(err, ErrNotFound) {
fmt.Println("Not found error")
}
}
19. How does Go's HTTP/2 support work, and what are the benefits of using HTTP/2?
Go’s HTTP/2 support is built into the net/http package. HTTP/2 is automatically used if the server and client support it.
Benefits of HTTP/2:
- Multiplexing: Multiple requests and responses can be sent over a single TCP connection.
- Header compression: Reduces the overhead of HTTP headers.
- Stream prioritization: Allows for prioritizing important resources.
- Server push: Servers can push resources to clients before they are requested.
Example:
- HTTP/2 Server:
package main
import (
"fmt"
"net/http"
)
func main() {
http.HandleFunc("/", func(w http.ResponseWriter, r *http.Request) {
fmt.Fprintln(w, "Hello, HTTP/2!")
})
server := &http.Server{
Addr: ":8080",
}
fmt.Println("Serving on https://localhost:8080")
err := server.ListenAndServeTLS("server.crt", "server.key") // Ensure TLS is enabled
if err != nil {
panic(err)
}
}
- HTTP/2 Client:
package main
import (
"fmt"
"net/http"
)
func main() {
client := &http.Client{}
resp, err := client.Get("https://localhost:8080")
if err != nil {
panic(err)
}
defer resp.Body.Close()
fmt.Println("Response protocol:", resp.Proto)
}
20. What is a goroutine leak, and how can it be detected and prevented?
A goroutine leak occurs when goroutines are created but never terminated, leading to memory and resource exhaustion.
Detection:
- Profiling tools: Use tools like pprof to detect goroutine leaks.
import _ "net/http/pprof"
- Runtime stack dump: Use the runtime package to print the stack trace of all goroutines.
import "runtime"
func dumpGoroutines() {
buf := make([]byte, 1<<20)
runtime.Stack(buf, true)
fmt.Printf("%s\n", buf)
}
Prevention:
- Ensure goroutines terminate: Use channels to signal when a goroutine should stop.
- Use context for cancellation: Use context. Context to handle cancellations and timeouts.
Example:
package main
import (
"context"
"fmt"
"time"
)
func main() {
ctx, cancel := context.WithTimeout(context.Background(), 1*time.Second)
defer cancel()
go func(ctx context.Context) {
for {
select {
case <-ctx.Done():
fmt.Println("Goroutine exiting")
return
default:
// Simulate work
time.Sleep(100 * time.Millisecond)
}
}
}(ctx)
time.Sleep(2 * time.Second)
}
21. What are the differences between net/http and fasthttp packages in Go?
- net/http: The standard HTTP package in Go, providing a robust and easy-to-use HTTP server and client implementation. It’s widely used and well-supported but might not be the fastest in high-performance scenarios.
- Pros: Simplicity, standard library, well-tested, supports HTTP/2.
- Cons: Relatively slower performance compared to fasthttp.
- fasthttp: An alternative HTTP package optimized for high performance and low memory footprint.
- Pros: High performance, low memory allocation, optimized for high concurrency.
- Cons: Non-standard API, might require more effort to integrate with existing projects, less mature compared to net/http.
Example:
- net/http Server:
package main
import (
"fmt"
"net/http"
)
func main() {
http.HandleFunc("/", func(w http.ResponseWriter, r *http.Request) {
fmt.Fprintln(w, "Hello, net/http!")
})
fmt.Println("Serving on http://localhost:8080")
http.ListenAndServe(":8080", nil)
}
- fasthttp Server:
package main
import (
"github.com/valyala/fasthttp"
)
func main() {
requestHandler := func(ctx *fasthttp.RequestCtx) {
ctx.WriteString("Hello, fasthttp!")
}
server := &fasthttp.Server{
Handler: requestHandler,
}
server.ListenAndServe(":8080")
}

Conclusion
Preparing for advanced Go (Golang) interviews requires a deep understanding of the language’s more complex features and its runtime behavior. Remember, successfully sitting through an interview is not just about knowing the right answers but also about showing your problem-solving approach and your ability to write efficient, maintainable code. Good luck with your interview preparation! Also, sign up with Olibr to find top opportunities for Golang Developers.
Take control of your career and land your dream job
Sign up with us now and start applying for the best opportunities!
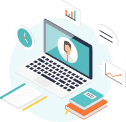
FAQs
Concentrate on understanding Go’s concurrency model, memory management, performance optimization techniques, and advanced usage of the standard library. Practice writing idiomatic Go code and solving complex problems.
Be ready to discuss goroutines, channels, sync package primitives (like Mutex, WaitGroup, Cond, etc.), and patterns for concurrent programming. Explain how you handle synchronization, avoid race conditions, and ensure efficient concurrent execution.
Go scheduler, garbage collection, reflection, interfaces and type assertions, context package for cancellation and timeouts, profiling and optimizing Go programs, and using sync.Pool.
Discuss best practices such as error handling, writing clean and idiomatic code, using proper design patterns, and maintaining a clear code structure. Demonstrate how you optimize code for performance and memory usage.
You might be asked to implement concurrent data structures, design APIs, solve performance bottlenecks, manage large datasets, or refactor existing code for better readability and efficiency.