Table of Contents
Toggle
Introduction
If you’re a beginner and curious to learn Next, then this guide is meant for you! Next.js’ versatile capabilities and outstanding features have taken control over web development. Hence, making it a crucial tool for developers. In today’s article, we have come up with a complete Guide to Next.js that will help beginners grasp the concept and use it for seamless web development.

What is Next.js?
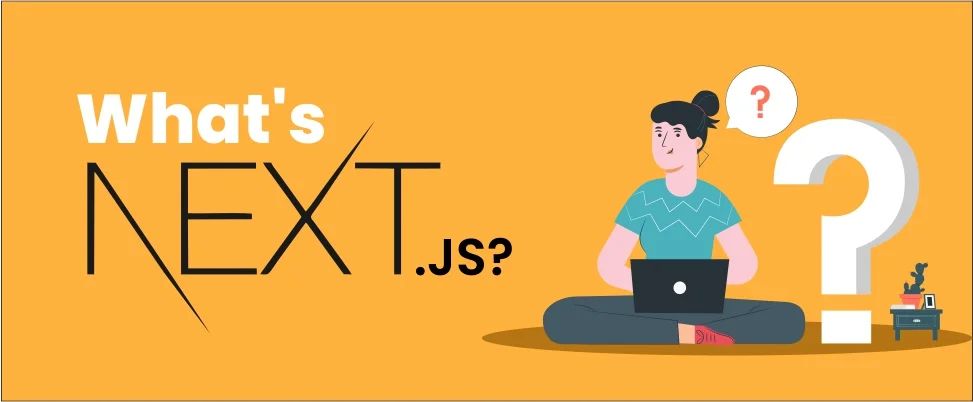
Next.js is an open-source React framework primarily used to build dynamic websites and web applications. It comes with automated routing, Server-Side Rendering (SSR), and compile-time rendering (SSG) features. This enables developers to build performant and scalable web applications.

Features of Next.js
- It allows developers to modify changes in the application without manual interruptions and then automatically reflect on the server.
- Developers can choose the styling with ease with the in-built support of CSS.
- Prefetch prop helps to optimize the performance by navigating automatic prefetch with the linked page resources.
- Automatic routing maps web page components to corresponding routes and then sets up the route for the newly created page in the application.
- Server-side rendering promotes fast initial load times and improves SEO to rank your site better in search engines.
- Automatic code split optimizes JavaScript code load and prevents unnecessary code from loading.
- Similarly, each route segment comes with its own layout that is shared across all the pages. This in return, maintains consistency and allows specific layouts for different sections in your app.
Now that you know what exactly is Next.js and its key features. Let’s learn how to install Next.js into your system.
Read more- What is Next.js? And What it is Used for?

Steps to Install Next.js Application
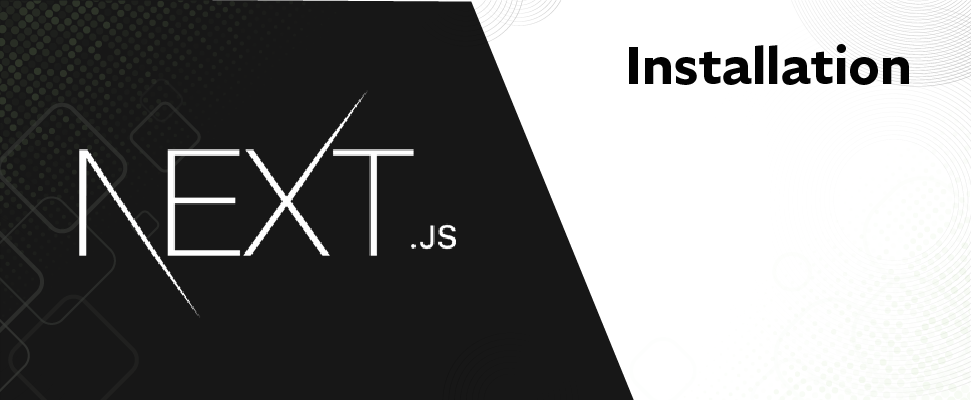
- To install and create a Next.js application in your system add the following command:
npx create-next-app my-next-project - It will install all the files and folders and set up the configurations as well as the other necessary items required to run the Next.js project.
- You will find the following scripts in your “package.json” file.
“dev“: “next dev“, // This will help your app to run a development server on localhost:3000
“build“: “next build“, // It will ensure that the application is ready for deployment
“start“: “next start“, // It prepares your Next.js app for running but you must execute the next build command first.
“lint“: “next lint” // It alerts you if there are any issues with your code.
- To run your Next.js project add the following code:
npm run dev
Note: Before running this command make sure that you’re in the project folder i.e., “my-next-project” then only run the above command. - Once you’re done following the above steps, you need to run on localhost:3000, you will see a default app.
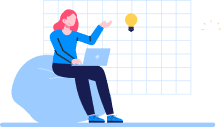
Don't miss out on your chance to work with the best!
Apply for top job opportunities today!

Adding Routes and Pages
Setting up the routes in Next.js is a piece of cake, when you write the command “create-next-app” in the above steps folder “pages” is created by default. This page folder maintains all the routes and the React component file is handled in a subdirectory in a separate route. Let’s say your folder contains three files those are index.js, about.js, and articles.js, these files will automatically transform into localhost/index, localhost/about, localhost/articles. The following is an example of the about.js page:
function AboutPage()
{
return (
<div>
<h1>About</h>
)
}
export default AboutPage
To create nested routes, make a subfolder as “article” on your page directory. Now, create a React component “contact.js” file inside the “article” folder. This file is accessible from this path “localhost/articles/contact”. Ultimately, you have two files i.e., “page/articles” and “page/article/index.js”, which represent the same path as “localhost/blog”. In such cases, Next.js renders the “article.js” file. Similarly, one can define dynamic routing in Next.js but you only need to write a file within brackets for e.g, page/article/[slug].js, have a look at the following code:
Import { useRouter } from ’next/router’
function PostPage()
{
const router = useRouter()
return (
<div>
<h1> My post: {router.query.slug} </h1>
</div>
)
}
export default PostPage

Linking
You have set up your first route now you have to connect the page with those routes, for that, you need to add ‘next/link’ at the beginning. Have a look at the following code, we have linked the page with the About page:
import Link from ‘next/link’
export default function Home() {
return (
<div>
<h1>Home</h1>
<Link href="about">About</link>
</div>
)
}
Sometimes you need to redirect routes to different pages in those cases, you need to use the “useRouter” hook along with the “.push()” method. The following is a dummy example:
import Link from ‘next/link’
import { useRouter } from ‘next/router’
function About()
{
const router = useRouter()
return
(
<div>
<h1>About Page</h1>
<p>This is the about page</p>
<button onClick={() => router.push(‘/’)}>Return to Home
</button>
</div>
)
}

SEO in Next.js
Meta tags play a crucial role in SEO and in order to achieve a better ranking in search engines, you must have this element within the HTML body. Due to this reason, you need to install React Helmet in your application. To add the metadata in your Next.js use the head component, here is the example:
import Link from "next/link";
import Head from "next/head";
export default function About() {
return (
<div>
<Head>
<title>About | My Cool Site</title>
<meta name="description" content="Hi, this is app built in Next.js" />
</Head>
<h1>About Me</h1>
<div>
<Link href="/">
<a>Home</a>
</Link>
<Link href="/article/test">
<a>My Blog Post</a>
</Link>
</div>
</div>
);
}

Components and Head Component
It is always a good idea to store all the “non-page” folders separately in your project root directory. This way you don’t need to create a component or layout files frequently. In Next.js the initial page load time is done on the server-side by modifying the HTML file from your page. Title and meta tags are managed by the head component in Next.js.
import Head from ‘next/head’
function layout (
{ title, keywords, description, children }
)
{
return
(
<div>
<Head>
<title>{title}</title>
<meta name = ‘description’ content={description}/>
<meta name= ‘keywords’ content={keywords}/>
</Head>
{children}
</div>
)
}
export default layout
Layout.defaultProps = {
title: ‘This is my first Next.js app title’,
description: ‘Next.js is the best for beginners’,
keywords: ‘web development, React, Next.js, JavaScript’
}

Page Not Found Error Message
If have deleted or moved content from your site or else you’re modifying the content, you can provide a custom 404 error page for users. Whenever Next.js encounters the 404 error, it will redirect the page. The following is the example for 404 error page.
import Layout from ‘. ./components/Layout’
function NotFoundPage()
{
return (
<Layout>
Sorry the page you are looking for is nowhere to be found
</Layout>
)
}
export default NotFoundPage

Data Fetching from Server-Side in Next.js
In Next.js, you need to use the “getServerSideProps” method to render each request on the server side. You can add this function at the end of the file and then Next.js will automatically fill with necessary data in your component props.
export async function getServerSideProps()
{
const res = await fetch (‘http://server-name/api/items’)
const items = await res.json()
return
{
props: {items}
}
}

Fetch Data during Build Time
export async function getStaticProps()
{
const res = await fetch (‘http://server-name/api/items’)
const items = await res.json()
return
{
props: {items},
}
}

To Sum Up
By following the above-mentioned steps, you can initiate your web development process. Eventually, you can start learning advanced topics such as API integration, state management, and authentication. Getting expertise in all of these will definitely make you a pro in Next.js. So keep practicing and exploring. Happy coding!
Take control of your career and land your dream job!
Sign up and start applying to the best opportunities!
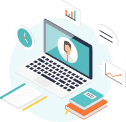
FAQs
It would not be a good idea to directly start with Next.js because Next.js itself is a React framework. If you learn the basics of React first, it will be quite simpler to start with Next.js. Otherwise, it will be difficult to understand how these two technologies work together.
Absolutely yes, it offers a pre-configured fundamental structure and functionality in your project that will save you time and effort.
Next.js is an ideal choice for creating dynamic single-page applications. It offers flexibility and support for client-side routing.
Yes, Next.js comes with a built-in server that helps in local development and testing.
React.js framework offers diverse options each one is tailored to specific development. All of these frameworks have their pros and cons, the best one depends on your project-specific needs. Whether you prioritize server-side rendering, or looking for a superfast, UI design, or form handling, there’s a React framework ready to assist you. So with this, we have come to the end of the 16 Best React.js Frameworks in your development process that will not only elevate your project quality but also enhance productivity.