Table of Contents
Toggle
Introduction
Every industry has a set of best practices to ensure long-term success and foster a culture of continuous improvement. The same goes for the IT and Development industry, which enforces the integration of best practices into processes to achieve better results. If you are a beginner in the coding industry, a good understanding of the best coding practices can help you establish yourself as a sincere team member. Also, a seasoned coding expert who can seamlessly work respecting the coding best practices is a valuable team member. In this blog, let’s explore the need to have best practices for coding and understand the best coding practices.
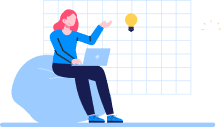
Don't miss out on your chance to work with the best
Apply for top global job opportunities today!

Coding Best Practices for Beginners
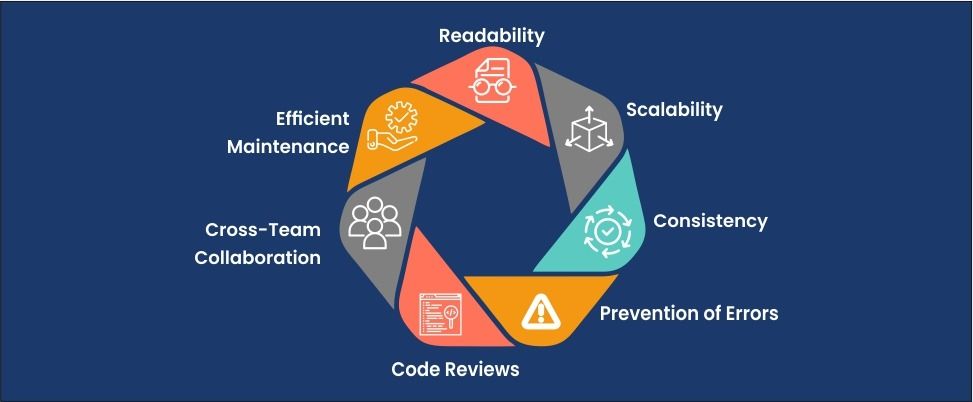
In a typical coding team, various roles team up to ensure the successful development and delivery of software projects. Whether it is programming for beginners or experienced developers, coding best practices are essential for several reasons:
Readability
Best practices ensure that your code is easy to understand, as code is often read more than it is written. This ensures better collaboration and maintenance.
Scalability
Best coding practices ensure that a codebase can grow and adapt to new requirements without becoming unmanageable.
Consistency
A team that works well around the best coding practices helps write code that is similar in style. This makes it easier to integrate and review code from different developers.
Prevention of Errors
When best practices are followed consistently, common mistakes are easier to catch. This helps prevent bugs and improve code quality.
Code Reviews
Standard best coding practices offer clear criteria that can be used to review code. This improves the process of feedback and revisions.
Cross-Team Collaboration
Best Coding practices ensure standard processes are in place when working across large teams and collaborating with other developers.
Efficient Maintainance
Coding best practices are in place to facilitate the performance of applications, as it simplifies debugging, refactoring, and maintenance tasks.

Coding Best Practices for Beginners
Courses designed to teach coding for beginners generally include a section that elaborately explains the best coding practices that you should remember as you learn to code.
Here are the key best coding practices for a new developer on the block:
Code Indentation
Proper indentation makes your code more readable and helps others understand the structure of your code. Let’s understand this with two examples:
C++
#include <stdio.h>
int find(int A[], int size) { int ret = -1; for(int i = 0; i < size; i++) {
if(A[i] > ret){ ret = A[i];
}
}
return ret;}
int main() { int A[]={1, 4, 7, 13, 99, 0, 8, 5}; printf("\n\n\t%d\n\n", find(A, 8));
return 0; }
This is an example of a code that will, of course, work without errors and produce correct output. However, even a seasoned programmer will find it challenging to identify the boundaries of functions, loops, and conditional blocks without proper indentation.
Let’s see how the code can be rewritten with proper indentation:
#include <stdio.h>
int find(int A[], int size) {
int ret = -1;
for (int i = 0; i < size; i++) {
if (A[i] > ret) {
ret = A[i];
}
}
return ret;
}
int main() {
int A[] = {1, 4, 7, 13, 99, 0, 8, 5};
printf("\n\n\t%d\n\n", find(A, 8));
return 0;
}
Meaningful Naming
The name of the code should be a clue to what it does. The best practice is to give intuitive names to classes, functions, variables, objects, and constants. At the same time, it is important to maintain the readability of the code. Let’s understand more with an example. Here is a code snippet with unclear naming, making it difficult to understand and reuse.
C++
#include <stdio.h>
int find(int A[], int size) {
int ret = -1;
for (int i = 0; i < size; i++) {
if (A[i] > ret) {
ret = A[i];
}
}
return ret;
}
int main() {
int A[]={1, 4, 7, 13, 99, 0, 8, 5};
printf("\n\n\t%d\n\n", find(A, 8));
return 0;
}
However, this code serves the same function but has been named meaningfully, which makes it easy to understand.
C++
#include <stdio.h>
int findLargest(int inputAry[], int inputArySize) {
int largest = -1;
for (int loop = 0; loop < inputArySize; loop++) {
if (inputAry[loop] > largest) {
largest = inputAry[loop];
}
}
return largest;
}
int main() {
int A[]={1, 4, 7, 13, 99, 0, 8, 5};
printf("\n\n\t%d\n\n", findLargest(A, 8));
return 0;
}
Contextual Comment
It is common knowledge that while the code is for the compiler, the comments exist for the coders. This implies that the comments should be helpful if the code is not self-explanatory. The best practice is to add comments that explain the purpose of complex code sections without over-commenting. Also, it is crucial to add comments that let your readers get the context, whether you have written or modified a code. Here is a good example of contextual commenting:
C++
#include <stdio.h>
/**
* Finds the largest integer from the given array (inputAry)
* of size (inputArySize).
*/
int findLargest(int inputAry[], int inputArySize) {
//Assumption: array will have +ve elements.
//Thus, the largest is initialized with -1 (smallest possible value).
int largest = -1;
// Iterate through all elements of the array.
for (int loop = 0; loop < inputArySize; loop++) {
//Replace largest with element greater than it.
if (inputAry[loop] > largest) {
largest = inputAry[loop];
}
}
//returns the largest element of the array
return largest;
}
int main() {
int A[]={1, 4, 7, 13, 99, 0, 8, 5};
printf("\n\n\t%d\n\n", findLargest(A, 8));
return 0;
}
DRY Principle
The “Don’t Repeat Yourself” principle ensures there is no duplication of code. Code can be made reusable by abstracting repeated sections into functions, improving both clarity and maintainability. This makes development more efficient. Additionally, without code duplication, it is easier to debug throughout the program.
Low Coupling and High Cohesion
These two complementary principles work together to ensure your applications can be maintained, scaled, and tested. While high cohesion facilitates integration between related parts of a codebase, low coupling allows separation between unrelated parts of a codebase.
Units that need to know about each other are kept close by high cohesion. It helps in finding related code when you need to extend code. Low coupling reduces dependencies between unrelated units. Failure to follow this best practice can lead to high coupling and low cohesion, which can cause excessive dependencies and negative impacts, such as bugs.
Consider the Context
Since coding guidelines differ based on the organization, industry, and even programming languages, it is important to keep in mind the context. While it is crucial to be aware of the best coding practices, it is also important to avoid generalizing rules without understanding the situation well.

Coding Best Practices for Experienced Programmers
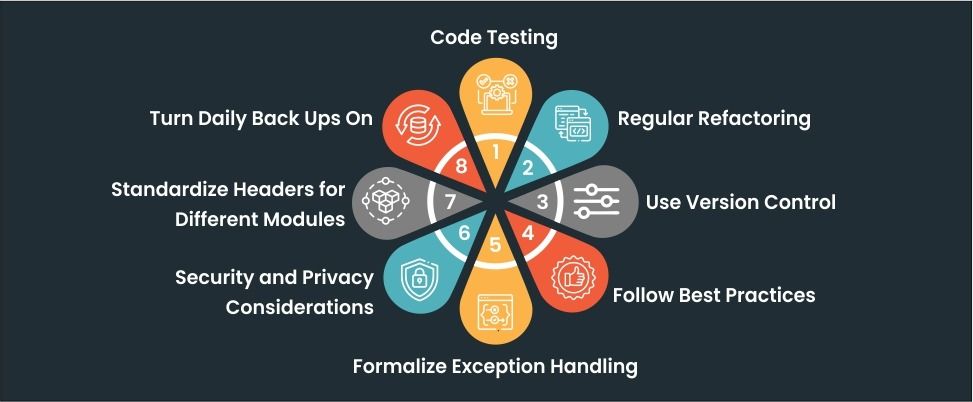
The way software works can reflect how well the best coding practices are being followed. Experienced programmers are at the forefront of writing code and building software that is high-quality, efficient, and easily scalable. Hence, it is crucial that they should follow the advanced coding best practices stringently.
Here are a few key practices:
Code Testing
Implementing automated unit tests, integration tests, and end-to-end tests helps ensure your code works as expected and also allows you to catch regressions early. Many experienced coders, in fact, write tests before writing the actual code, which tests each piece of functionality thoroughly.
Regular Refactoring
Experienced coders often have to restructure existing code without altering its external behavior to improve its readability, performance, and maintainability. Regular refactoring removes technical debt and keeps the codebase clean. It also identifies and addresses code smells and ensures code quality.
Use Version Control
It is a standard practice among experienced coders to use Git Flow and other branching strategies. This helps to handle feature development, bug fixes, and releases. Additionally, seasoned programmers also write clear and descriptive commit messages to document changes effectively.
Formalize Exception Handling
A team of experienced coders believes in implementing a consistent approach to exception handling across the codebase as it helps manage errors gracefully. Seasoned coders use logging to capture and analyze exceptions. This makes it easier to debug and monitor your application.
Follow Best Practices
Although it might seem like an obvious action, often, a coding team with different levels of experience and tight deadlines might find it difficult to follow the best practices. Thus, it becomes the responsibility of the experienced team members to enforce coding standards and style guides to ensure consistency and readability.
Security and Privacy Considerations
Seasoned coders should validate all inputs so that injection attacks and other vulnerabilities can be avoided. Additionally, it is also essential to encrypt sensitive data both in transit and at rest to protect user privacy.
Standardize Headers for Different Modules
What this does is that it offers clear information about each module, such as its purpose, author, and date of creation. Maintaining thorough documentation for each module helps with an easy understanding of the code.
Turn Daily Back Ups On
The horrors of data loss can translate into wasting millions to billions of dollars to revive data. Turning on daily backups of your code and data is the simplest way to prevent data loss and ensure recovery from unexpected issues. A great best practice is to opt for automated backup solutions as it reduces the risk of human error and streamlines the process.

Conclusion
In any industry, best practices serve to enhance efficiency, consistency, and quality. Best coding practices for developers offer structured methods to ensure that your code is readable, maintainable, and scalable. Whether you are a new coder or an experienced programmer, these best practices ensure that the process of software development has a high success rate and minimum hiccups. It is important to understand and apply the best coding practices to ensure high-quality, efficient, and secure code, facilitating better collaboration and long-term project success. Sign up with Olibr now for an in-depth professional skills assessment.
Take control of your career and land your dream job
Sign up with us now and start applying for the best opportunities!
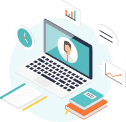
